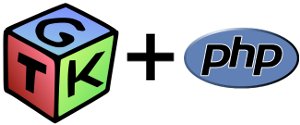
Many find it hard to believe, but not only govnokod and scripts can be written in PHP, but also small GUI applications. Small, because the topic is not very popular and poorly lit due to the fact that there are more convenient and powerful tools for development, respectively, the tools are quite raw. Although for writing applications "for yourself" or course \ diploma is quite suitable.
The article provides an example of creating a simple GTK + application in PHP.
GTK + is a cross-platform library of interface elements that is popular in Linux systems and has recently been used quite often in Windows.
I will try to answer in advance the question: “Why is it necessary, can I write it in Python?”. Of course you can, but:
1. It’s hard to believe, but there are people who know PHP well and they don’t have time to learn Python, but you need to write a GUI app.
2. Writing in PHP works wherever there is PHP with the necessary libraries (Windows, Linux, MaxOS).
Let's start
Usually, to demonstrate the charms of a language or library, HelloWorld! Is written, but we will go a little further and write a calculator.
')
The whole process is quite simple and consists of two steps:
1. Development of the interface in the Glade Interface Editor
2. Develop an application using the interface created in the previous step.
Installation
Of course, it is not necessary to use Interface Builder to create an interface. Glade file is xml, which you can write with your hands or you can create an interface directly in a PHP script. But since the purpose of this article is to show the simplicity of creating applications, we will go the easy way and use Drag'n'Drop as the interface editor.
You can download it here:
http://glade.gnome.org/sources.htmlOr:
[aptitude|yum] install glade
The PHP library that we will use only works with PHP 5.1 and higher, so we acquire it:
http://php.net/downloads.phpBy default, there is no GTK library in PHP, so it needs to be installed.
Downloading:
http://gtk.php.net/download.phpInstall:
http://gtk.php.net/manual/en/tutorials.installation.phpFor installation on Linux, it is better to pull the source from SVN. Before configuration, install cairo-php:
pecl install cairo-beta
If the configuration falls by an error: "` lt_if_append_uniq (lt_decl_varnames, ... "do in the folder php-gtk:
cat /usr/share/aclocal/ltoptions.m4 /usr/share/aclocal/ltversion.m4 /usr/share/aclocal/ltsugar.m4 /usr/share/aclocal/lt~obsolete.m4 >> aclocal.m4
Then restart ./buildconf and ./configure
Interface
We will not invent any complicated features, we will make a simple interface.
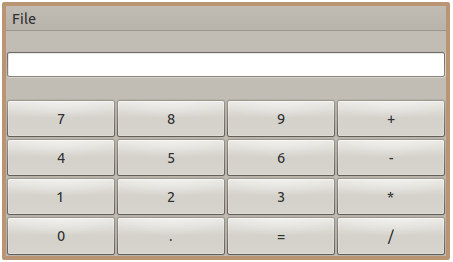
First we create the Window from the Common tab, which we set Visible = true. On the window we overtighten the Vertical Box divided into 3 regions. In the upper region we place the Menu Bar, in the middle Text Entry, and in the lower Table (4x4) in which we create the buttons as in the figure above.
Now we will write event handlers for the interface elements.
For each button:
- Click on the button
- Turn on the tabals Signals
- In the field “Clicked” we write enterValue for buttons 0-9, performAction for operation buttons and clearCalc for “C”.
- In order for PHP to understand which button is pressed, we prescribe a Name for each of them, for example:
"0": "input0"
...
"9": "input9"
"*": "Action_mul"
"/": "Action_div"
"+": "Action_add"
"-": "action_min"
"=": "Action_calc"
"C": "action_clear"
And the final touch - exit from the application when clicking on File-> Quit, for this we set the quit handler for the activate signal for this menu item.
After that, we save the created form in the calc.glade file, in the LibGlade format, and put it in the folder with the future application.
Programming
Create a PHP file "calc.php" in which we write:
<?php $glade = new GladeXML('calc.glade'); Gtk::main();
The first line loads the file with the interface, the second creates all the elements.
Run and enjoy the form:
php calc.php
Now you need to write the implementation of event handlers and the calculator itself.
The event handlers that we described in the Glade Interface Editor are ordinary functions or methods of the PHP class. At each handler, one argument is passed to the call - the object for which the handler is called. For simplicity, the name of this object will be used as an identifier of the operation or the value that you need to enter.
Create a class “Calc” which will contain event handlers and methods necessary for calculating:
<?php class Calc { protected $glade; protected $firstParam = null; protected $operation = null; function __construct($glade) { $this->glade = $glade; } protected function calculate($operation) { $secondParam = (float) $this->glade->get_widget('entry1')->get_text(); $firstParam = (float) $this->firstParam; $result = 0; switch($this->operation) { case '*': $result = $firstParam * $secondParam; break; case '/': $result = ($secondParam > 0 ? $firstParam / $secondParam : 0); break; case '+': $result = $firstParam + $secondParam; break; case '-': $result = $firstParam - $secondParam; break; } $this->glade->get_widget('entry1')->set_text($result); $this->firstParam = $result; $this->operation = null; } public function performAction($obj) { if ($this->firstParam == null) { $this->firstParam = $this->glade->get_widget('entry1')->get_text(); $this->glade->get_widget('entry1')->set_text(''); } if ($this->operation == null) { $this->operation = str_replace( array('action_mul', 'action_add', 'action_min', 'action_div'), array('*', '+', '-', '/'), $obj->name ); $this->glade->get_widget('entry1')->set_text(''); } else { $this->calculate($obj->name); } } public function enterValue($obj) { $this->glade->get_widget('entry1')->set_text( $this->glade->get_widget('entry1')->get_text(). str_replace('input', '', $obj->name) ); } public function clearCalc($obj) { $this->firstParam = null; $this->operation = null; $this->glade->get_widget('entry1')->set_text(''); } public function quit() { exit; } }
Finally, we point out that all event handlers are stored in the Calc class:
$glade->signal_autoconnect_instance(new Calc($glade));
Well that's all.
php calc.php
All code of the Github example:
https://github.com/kooler/php-glade-example