Most programs from Sysinternals use undocumented features. I had enough of this fact to be interested in this topic. I wonder how cool guys use undescribed functions in their equally cool programs.
We assume that we are lazy programmers to the necessary degree, we know C, in trouble with WinAPI and with the architecture of modern Windows and we have Ida Pro, hehe. We want to accomplish the task beautifully, quickly and efficiently, without reinventing the wheel (and so that we don’t have to strongly overload our hands and head).
Let's look for experimental function. A lot of tasty can be found in ntdll.dll. I myself am writing from under Win7 64, but I took the 32-bit version of the wonderful library. Just in case:% SystemDisk% \ Windows \ System32 \ ntdll.dll.
To make it easy, open ntdll in Ida and see which functions are exported. If there is no Ida, then you can take any program that works with PE files (for example, PETools). We are interested in functions with the prefix Rtl (Run Time Library). That is, during the execution of our code, we can ask this system function for the service.
')
After a small search for a simple function, it was found - RtlComputeCrc32.
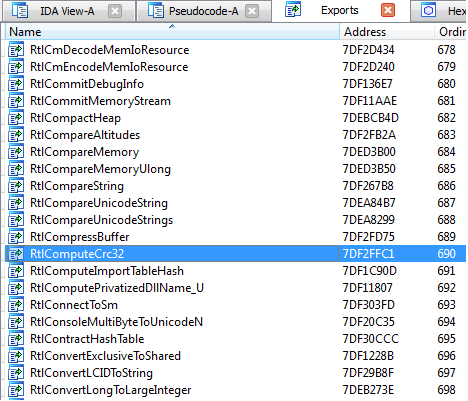
Double-click the function name to get its disassembled code. You can study the function with any other disassembler like HDasm or W32Dasm. In order not to waste space, I’ll give the pseudocode RtlComputeCrc32 kindly provided by the Ida decompiler (in the body of the function, press F5 if Hex-Rays Decompiler is available in Edit-> Plugins plugins).

Immediately we get a lot of information! We need to think what we are actually looking for. We need:
1) the name of the function to get its address in ntdll;
2) the function prototype to create the correct pointer to it;
3) an approximate principle of operation in order to pass the correct arguments to it and correctly process the result;
Items 1-2 we already have from pseudocode. Our task now is to understand the functions and on its basis to write a program that calculates the CRC32 from something.
By pseudocode, it is easy to understand that the function enumerates the bytes of the array
a2 , the size of which is
a3 , and
a1 - the initialization value of the algorithm. Having done the calculations with bytes, it obtains an index from the RtlCrc32Table table (double click will show the monstrous table). We google CRC32 and examples of implementation and we understand that everything is correct.
Things are easy - take advantage of loot. I made an empty console application in Visual Studio, but, of course, you can do it in any other environment.
GetModuleHandle () returns the handle of ntdll, GetProcAddress () - the address of the function. Use a pointer to a function of type UndocFoo to call RtlComputeCRC32 ().
#include <stdio.h> #include <windows.h> typedef INT (WINAPI *UndocFoo)(INT accumCRC32, const BYTE* buffer, UINT buflen); int main() { HMODULE hDLL = GetModuleHandle(TEXT("ntdll.dll")); if (hDLL == NULL) { puts("[-] Failed to find ntdll.dll\n"); return EXIT_FAILURE; } puts("[+] Got ntdll.dll handle\n"); UndocFoo ComputeCrc32 = (UndocFoo)GetProcAddress(hDLL, "RtlComputeCrc32"); if (ComputeCrc32 == NULL) { puts("[-] Failed to find RtlComputeCrc32\n"); return EXIT_FAILURE; } puts("[+] Found RtlComputeCrc32 address\n"); puts("[*] Calling RtlComputeCrc32...\n"); BYTE buffer[] = {0x01, 'a', 7}; INT iCRC32 = ComputeCrc32(INT(0), (BYTE*)buffer, 3); printf("[+] Computed CRC32 --> 0x%x\n\n", iCRC32); puts("Press any key to quit\n"); getchar(); return EXIT_SUCCESS; }
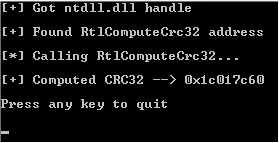
Success. You can check with any online calculator.
Our bytes 016107 yielded CRC32 = 0x1c017c60.

The same thing produced an online computer:
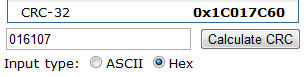
"
So, without spending time on implementing your own function or using someone else's big code, we made such a wonderful program. It was easy and fun.