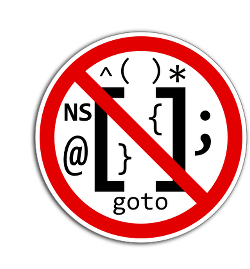
The other day, out of curiosity, I decided to see what stage
the Eero project is in - an Objective-C dialect with an alternative light syntax. It turned out that a large scope of work had already been done and Eero was a very interesting development.
Code example
For starters, the traditional comparison of a small piece of code on Eero and Objective-C.
Objective-c #import <Foundation/Foundation.h> @interface FileHelper : NSObject @property (readonly) NSString* name; @property (readonly) NSString* format; -(NSFileHandle*) openFile: (NSString*) path; -(NSFileHandle*) openFile: (NSString*) path withPermissions: (NSString*) permissions; @end @implementation FileHelper -(NSString*) name { return @"Macintosh"; } -(NSString*) format { return @"HFS+"; } -(NSFileHandle*) openFile: (NSString*) path { return [NSFileHandle fileHandleForReadingAtPath: path]; } -(NSFileHandle*) openFile: (NSString*) path withPermissions: (NSString*) permissions { NSFileHandle* handle = nil; if ([permissions isEqualTo: @"readonly"] || [permissions isEqualTo: @"r"]) { handle = [NSFileHandle fileHandleForReadingAtPath: path]; } else if ([permissions isEqualTo: @"readwrite"] || [permissions isEqualTo: @"rw"]) { handle = [NSFileHandle fileHandleForUpdatingAtPath: path]; } return handle; } @end
Introduction
Eero is a fully interfaced and binary compatible Objective-C dialect. It is implemented using the LLVM / clang compiler fork, i.e. generates a binary code directly, thanks to which standard LLVM / Clang development tools work with it, including a debugger, a static analyzer and others. Also declared experimental conversion of Eero code into standard Objective-C code
1 .
In Eero, you can directly use all Cocoa frameworks and any Objective-C / C ++ code, you just need to connect the necessary header files. The reverse process is also easy: if the interface for an Eero-class is defined on Objective-C, then it can be used directly, the binary files are fully compatible.
')

Essentially, Eero is a powerful syntactic sugar for Objective-C, increasing readability, security, and reducing code. According to the author
(Andy Arvanitis) , initially he just wanted to get rid of the brackets in Objective-C, and chose the name of the language when he saw the model of the pedestal table, which successfully performed its functions without unnecessary interfering legs. So, the language is named after the Finnish table architect
Eero Saarinen . I am not strong in Finnish, but apparently the name should sound something like an “ero” with the accent on the first syllable.
Syntax
The documentation is written qualitatively and is easy to read, the motivation is indicated for each language feature, why it was decided to do this, it is basically: readability, security,
DRY ,
WYSIWYG . There are many interesting details in the language, I will not consider everything, but I will limit myself to a small subjective list.

First, in Eero, padding is used to select blocks instead of curly braces, as in Python. Semicolon at the end of instructions and definitions is not required.
int count = 0 while ( count < 100 ) something = false count++ i++; j++

In Eero, sending messages is recorded using dot notation, for messages with no arguments, this is similar to the notation for properties. Arguments are passed after the colon, several arguments are separated by commas.
id myarray = NSMutableArray.new myarray.addObject: myobject myarray.insertObject: myobject, atIndex: 0

Since an object cannot be represented in Objective-C except in terms of a pointer, in Eero all class type variables are treated as pointers, without using an asterisk
(*) .
NSString mystring = myobject.description NSString otherString = (NSString) someObject

When declaring local variables, you can omit their type, it will be automatically deduced from the assigned expression. To do this, use the special operator ": =".
i := 100 mystring := myobject.description

Eero implements such a wonderful thing as
namespaces . The “NS” prefix is ​​enabled by default, i.e. All types from the
Foundation framework can be used without a prefix.
mystring := String.stringWithUTF8String: "Hello, World"
The compiler first checks the names of the entities as they appear in the code, and, if the name is not found, adds the registered prefixes. Additional prefixes are declared using the directive
using prefix
.
using prefix AB ... theAddressBook := AddressBook.sharedAddressBook
However, in the current implementation there is a significant drawback: the occurrence of collisions as a result of the omission of prefixes is not monitored in any way, the first suitable option is simply used:
void AAPrint(String str) NSLog('AA: %@', str); void BBPrint(String str) NSLog('BB: %@', str); using prefix AA using prefix BB ... Print('test')

In Eero, it is not necessary to specify the names of method arguments, in the case of omission, they are automatically determined from the selector. For example, in the following method, the arguments will be named
bytes ,
length, and
encoding :
initWithBytes: const void*, length: UInteger, encoding: StringEncoding
The type of the return value is specified after the arguments, the default is
void .
initWithBytes: const void*, length: UInteger, encoding: StringEncoding, return id
In Objective-C, when a method has optional parameters, it is customary to define several methods with different numbers of arguments. In Eero, you can declare methods with optional parameters.
interface MyClass openFile String, [withPermissions: String], return FileHandle end
In the implementation, the default value of an optional argument is denoted by the "=" sign.
implementation MyClass openFile: String, withPermissions: String = 'r', return FileHandle handle := nil if permissions == 'r' handle = FileHandle.fileHandleForReadingAtPath: file else if permissions == 'w' or permissions == 'rw' handle = FileHandle.fileHandleForUpdatingAtPath: file return handle end
You can specify the default return value, which for example allows you to define very compact getters:
implementation MyClass model, return String = 'G35' serialNumber, return String = 'X344434AABC' end

Properties are very simple to declare. Attributes are, if necessary, specified in curly braces.
interface MyClass String name {nonatomic, copy} String desc {readonly} end

Indentation is used when defining blocks, and the caret "^" is not used. Compared to Objective-C syntax, blocks look very simple:
myblock := (int x, int y) if x < 0 printf( "value was negative! (%d)\n", x ) x = 0 return x + y
A compact form of writing is also supported for simple blocks consisting only of the returned expression:
xyblock := (int x, int y | return x + y) descriptions := mylist.mapWith: (id element | return element.description)

Eero has operator overloading. Firstly, for all objects the operator "==" is an alias for the
isEqual method. What is important primarily for readable and safe string comparison:
mystring := MutableString.new mystring.appendString: 'Hello, World' if mystring == 'Hello, World'
When using the "+" binary operator, the message
stringByAppendingString will be sent to all objects that can respond to it (mainly
NSString and its subclasses):
helloString := 'Hello' worldString := 'World' helloWorldString := helloString + ', ' + worldString
Similarly, the operator "<<" sends the message
appendString :
mystring := '' mystring << 'Hello, World'
For your classes, you can overload the following operators:
Operator | Selector | Note |
+ | plus: | Including operator + = |
- | minus: | Including operator - = |
* | multipliedBy: | Including operator * = |
/ | dividedBy: | Including operator / = |
% | modulo: | Include operator% = |
< | isLessThan: | |
<= | isLessThanOrEqualTo: | |
> | isGreaterThan: | |
> = | isGreaterThanOrEqualTo: | |
<< | shiftLeft: | |
>> | shiftRight: | |

Well, finally,
goto
not allowed in the compiler level language. :)
Installation and use
This section is written from the experience of using Eero in the assembly 2013-12-08, XCode 5.0.2 and Mac OS X 10.8.5.Putting Eero is very simple. It is enough to
download and install the plug-in for Xcode, which already contains the fork compiler LLVM. The installation will also add
Xcode templates to create new
.eero and
.eeh files. After installation, just restart Xcode and that's it, you can get to work.
Syntax highlighting, autocompletion works. However, the drop-down list does not work with class navigation, instead the static inscription
“No Selection” .

More precisely, Xcode does not see the classes and methods defined in the file, but you can use the instructions
#pragma mark ...
, they are visible in the drop-down list.
Checked the debugger
(he debugger) , everything is in order. Unfortunately, however, Xcode crashed while trying to view
quick help to the highlighted code. Auto-indents are not always working correctly, but this is just a small thing. No more problems detected.
Conclusion
Personally, Eero made the most pleasant impression on me. Yes, the language is quite young
(domain eerolanguage.org was registered in January 2011, and the project appeared on github in December of the same year) , and therefore there are drawbacks characteristic of this stage of development
(mainly with integration with the IDE) . However, intractable and critical problems are not visible, but because I had a strong desire to try it in future projects.
Links
1) At the moment, the conversion function Eero -> Objective-C is damp, I have successfully worked only on elementary examples.