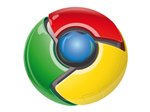
Browser extensions are very popular nowadays. There is always a reason to write an extension, and there are still plenty of them.
In this article I want to talk about how I wrote a small extension for google chrome for personal use. And the purpose of the article is to help a young programmer who has difficulty understanding English. Not everyone in the 3rd course will be able to read Google documentation, which is only in English. And I want to make an extension.
If you search, then in Russian there is nothing sensible about the development of extensions for chrome, only this
article is available describes the very basics.
')
This post will be a more advanced version.
Let's get startedI do not want to go into the very basics and therefore I recommend reading
this article for a start.
Download my extension and watch the code in parallel with reading.
We already know approximately what
manifest.json is and why it is needed.
In short, this is the main file extension, which tells the browser what things the extension will use, and the basic parameters (name, description, etc.).
Let's go directly to my extension.
This is a simple script that removes ads from the freelance page that make it difficult for me to look for ads that are interesting to me (more precisely, I’m only looking for long-term projects). Addition to the existing built-in filter.
manifest.json{
"name" : "Ffilter" ,
"version" : "1.0" ,
"background_page" : "bg.html" , // background .
"icons" : {
"48" : "icon_48.png" ,
"128" : "icon_128.png"
},
"page_action" :{ //
"default_title" : "Ffilter" ,
"default_icon" : "icon_19.png" ,
"default_popup" : "popup.html" //
},
"permissions" : [ //
"tabs" , "http://www.free-lance.ru/*"
],
"content_scripts" :[{ // DOM .
"matches" : [ "http://www.free-lance.ru/*" ],
"js" : [ "jq.js" , "script.js" ]
}]
}
* This source code was highlighted with Source Code Highlighter .
Consider.
The name, the version is clear to everyone.
background_page - here should be the name of the background page. The background page is a very important element, although for some applications it is not mandatory. But in our cases we cannot do without it.
The background page always works when the extension is working (that is, when it is enabled). She is always alone and can communicate and control all other elements.
Icons need different sizes to display in the address bar, in the list of extensions, near the address bar.
page_action is an important object. It tells the browser that our extension will be individually for each tab, that is, the icon will be displayed in the address bar, and not on the panel (for example,
gmail checker ). Extensions like
gmail checker are not considered individual, they open once for the entire browser (for them, the
browser_action object is used in the manifest instead of the
page_action object, in the pictures, respectively).

default_title ,
default_icon - name, icon, respectively.
default_popup is the name of the html extension page that will pop up when you click on the icon. Look at the previous topic, if not all is clear.
permissions is an array with permissions. It is useful for us to communicate with the system object
tabs and contact the address of the freelance (meaning not
ajax requests, but
js working with the page).
content_scripts is an important object for us; it is this object that allows
js to be used on the freelance page. We specify the address of the page and specify the
js files that will be executed immediately after the
body is loaded. I use
jQuery and define my functions. Order matters.
It is important to know that the extension scripts cannot see the scripting objects / variables of the page itself. This means that if the page already has its own
jQuery, we will not be able to use it, you should definitely upload your own. This is called
isolated worlds and it is sometimes convenient. Scripts from the extension, of course, can manipulate the
DOM .
We go further.
If you have correctly imagined everything, then you can see that our extension consists of 3 main objects: a background page (one for all), a window with a filter (for each page), and scripts on each page.
The general algorithm is as follows:
The filter should communicate only with the script on the current page. The script should only take instructions from the filter on the current page and execute them. Both that and another should not communicate with the background page, but the background page has to control the filter. After all, the filter should appear only on the freelance page. And, by the way, the
page_action extension is always hidden by default, and it needs to be enabled via the background page, which it does when the freelancing page loads.
bg.htmlchrome.tabs.onUpdated.addListener( function (id,info,tab) {
if (info.url)
if (/free-lance.ru/.test(info.url))
chrome.pageAction.show(id);
});
* This source code was highlighted with Source Code Highlighter .
Only
js code is given (only it is needed).
chrome.tabs is the system object with which you work the most, as you guessed, it is responsible for the tabs.
We hang our function on the
onUpdated event (update tab, click on the link). The parameters are the tab identifier, the update information, the tab object itself. We need information - it contains the address of the current page. We check if this is freelance and if so, then call the
show method of the
chrome.pageAction object passing the identifier of this tab there.
chrome.pageAction is the object responsible for the extensions inside the address bar, and we ask to show the icon in the tab we need (where the freelancing has just opened).
All actions of the background page are over, the site has opened, the icon has appeared, now you can click on it and popup.html will appear.
popup.htmlIt makes no sense to disassemble all the code, it is only important to know how to access the script that is waiting on the page from this window, how to organize the transport?
In general, the documentation immediately suggests this method:
chrome.tabs.executeScript( null , {code: "alert('hello!')" });
* This source code was highlighted with Source Code Highlighter .
or you can:
chrome.tabs.executeScript( null , {file : “script.js”});
* This source code was highlighted with Source Code Highlighter .

But think how to transfer parameters there? Only string? (by the way,
null - this means that we want to execute the script on the current tab)
It suited me, I was looking for a way to transfer objects. And this method was found, but it was hidden in the documentation.
port is a special object in chrome, with which you can communicate from script to background page and popup, or from background page and popup to script.
To connect to the script you need to connect through tabs:
var port = chrome.tabs.connect(id);
* This source code was highlighted with Source Code Highlighter .
where id is the number of the required tab with the script.
port is the returned transport object.
To connect to the background page or pop-up window, you need to refer to the extension object:
var port = chrome.extension.connect();
* This source code was highlighted with Source Code Highlighter .
One extension - the identifier is not required.
You can listen to these connections equally there and there:
chrome.extension.onConnect.addListener( function (port){
port.onMessage.addListener(MyFunc);
});
* This source code was highlighted with Source Code Highlighter .
Why
onMessage will become clearer later.
Let's go back to popup.html.
In this place I associate with the script, which is “on duty” on this page.
For me it became a problem that you need to specify the tab ID, but I don’t know this ID. It's funny that we need the current tab, and we could just send
null instead of a number, but this will not work - you need to know the ID. How?
He killed a lot of time for this and did this:
chrome.windows.getCurrent( function (w){
chrome.tabs.getSelected(w.id, function (t){
port = chrome.tabs.connect(t.id);
})
});
* This source code was highlighted with Source Code Highlighter .
We ask to tell which window is currently active, and the window object is returned to us in the
callback . Further we ask to tell the current tab in this window and it is returned to us also in
callback . And only then we open the connection with the script.
It works, but it seems to me that this is wrong - there must be a simpler way.
When all the filter data is collected, by clicking on the button we send an object to this port using the method:
port.postMessage(obj);
* This source code was highlighted with Source Code Highlighter .
And at that moment script.js starts working.
In
script.js , this is important:
chrome.extension.onConnect.addListener( function (port){
port.onMessage.addListener(Filtr);
});
* This source code was highlighted with Source Code Highlighter .
We listen to the port and wait for the incoming connection with the
port object.
This object has an
onMessage event - an event when a message is sent here. We hang our
Filtr function, which will take all the arguments that will be sent using
port.postMessage () at the other end. The
Filtr function will remove all ads that are listed in the object.
EverythingThat's all. It works and helps me in my job search.
It was difficult to understand without knowledge of English.
I hope you come in handy.
Documentation extension .