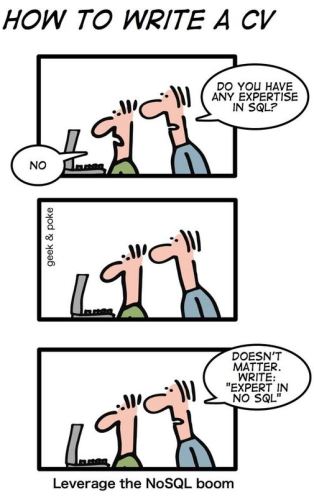
Currently, more and more high-load projects are operating with a huge amount of data. And it is no longer possible to get by with the classical relational storage model for this information. NoSQL databases are becoming increasingly popular (NoSQL stands for Not only SQL). One such database is MongoDB, which has already earned the attention of companies such as
Disney, craiglist, four square . In addition, there have repeatedly written about her:
NoSQL using MongoDB, NoRM and ASP.NET MVCMongoDB Sharding on the fingersMongoDB replication on fingersThis is another article about working with MongoDb in a .net environment.
')
What is required:
1. Download (
http://www.mongodb.org/downloads ), unpack and run mongod (this is the server)
2. Driver (
https://github.com/mongodb/mongo-csharp-driver/downloads )
3. Let's go
Database creation.
By default, the database is located in the folder c: / data / db
Run mongo.exe and create a new database:
use mongoblog
Immediately set the access parameters (username and password):
db.addUser("admin", "masterkey")
A feature of MongoDb is that it is a document-oriented database, and does not contain information about the structure, so here we are done. Immediately proceed to the description of the data models.
We will have two entities, Article (Article) and Comment (Comment), and the Article will contain many Comments:
public partial class Article { [BsonId] public ObjectId Id { get; set; }
Next, consider working with MongoDB:
- connect to mongo
- adding an article
- article change
- indexing
- delete article
- output articles (filtering / paging / sorting)
- adding comments
- post removal
- Search
- backup base
# 1 Connection to the database.
By default, the database occupies port 27017 on the server (we usually have localhost). Connect to the database:
public MongoDBContext(string ConnectionString, string User, string Password, string Database) { var mongoUrlBuilder = new MongoUrlBuilder(ConnectionString); server = MongoServer.Create(mongoUrlBuilder.ToMongoUrl()); MongoCredentials credentials = new MongoCredentials(User, Password); database = server.GetDatabase(Database, credentials); }
# 2 / # 3 Adding / editing an entry
To add an object to the collection, you must obtain a collection by name.
var collection = database.GetCollection<T>(table);
To add, you can run the command:
collection.Insert<T>(obj);
or (as for change)
collection.Save<T>(obj);
MongoDb independently adds the _id field - a unique parameter. If, when the Save command is executed, the object has an Id that already exists in the collection, then an update of this object will be executed.
# 4 Indexing
For a quick search, we can add an index for any field. For indexing, use the command
collection.EnsureIndex(IndexKeys.Descending("AddedDate"));
This will speed up sorting by this field.
# 5 Removal
To delete by id a query is created (Query). In this case it is
var query = Query.EQ("_id", id)
and the command is executed:
collection.Remove(query);
# 6 Conclusion of articles
To display the values by applying the filter and sorted, and even with a paging cursor is used.
For example, to select from the collection the unreleased (IsDeleted = false) sorted by descending date (AddedDate desc) 10th page (skip 90 elements and display the following 10) a cursor is composed:
var cursor = collection.Find(Query.EQ("IsDeleted", false)); cursor.SetSortOrder(SortBy.Descending("AddedDate")); cursor.Skip = 90; cursor.Limit = 10; foreach (var obj in cursor) { yield return obj; }
# 7 Add comments.
Since MongoDb is a document-oriented database, there is no separate table with comments, and the comment is added to the comments array in the article, after which the article is saved:
var article = db.GetByID<Article>("Articles", articleId); comment.AddedDate = DateTime.Now; article.Comments.Add(comment); db.Save<Article>("Articles", article);
# 8 Delete a comment.
The comment is deleted in the same way. We get the article object, delete the current comment from the list of comments and save the object.
# 9 Search.
To find an element containing a given substring, you must specify the following query:
Query.Matches("Text", @".* .*").
There are 2 problems as the search is case sensitive, i.e. Peter! = Peter, and besides, we have many fields.
The first problem can be solved
using regular expressions.But in order to get rid of both problems at once, I created a Bulk field where all the fields + comments are written in lower case and I search for this field.
# 10 Backup Base.
It is backup and not replication. In order to have access to the database file, you need to execute lock (the database will continue to work, only at this moment all write commands will be cached so that it will be executed later). Then the database can be copied, archived and put on ftp. After this procedure, the base must be unlocked (Unlock).
Commands:
public void Lock() { var command = new CommandDocument(); command.Add("fsync", 1); command.Add("lock", 1); var collection = server.AdminDatabase.RunCommand(command); } public void Unlock() { var collection = server.AdminDatabase.GetCollection("$cmd.sys.unlock"); collection.FindOne(); }
If something goes wrong, then the database server will need to be restarted with the command:
mongod --repair
Sources
A trial application (on asp.net mvc) can be found at:
https://bitbucket.org/chernikov/mongodbblogYes, and about the performance in relation to the rest of the
link .