Foxby is a library for working with Word documents in OpenXml format. Designed for generating documents
from a template and
making changes to existing documents. With it, you can stop working with COM + or editing an OpenXML tree through the
OpenXML SDK and go to the mechanism for constructing a document structure from meta elements in a declarative form.
In the class of tasks: creating paragraphs, creating and filling in tables, lists, working with images, formatting, etc. In fact, the library is a wrapper over the OpenXML SDK with the API in the form of a
Fluent interface .
Benefits of using
We ourselves use this library already in two projects. The first version was written by
Eskat0n and was born as part of the project subsystem for working with Word documents, but later was put into an independent project.
')
The following advantages can be highlighted:
- On the server where your application is running, no installation of Microsoft Office is required.
- No problem with frozen Word processes.
- Works much faster than COM +
- Creating and modifying documents does not require writing to the hard disk, everything happens in RAM
- Convenient Fluent API for working with Word objects
- Ability to build your own language markup document
Installation
The latest version can be installed via
NuGet :

Source Code and License
The source code is written in .NET 4.0 / C # and uploaded to
Google Code: fluent-openxml by
IndyCode under the
LGPL license.
Examples of using
Below are examples with which you can start working with the library.
Example # 1 - Hello Word!
private static void Main()
{
using ( var docxDocument = new DocxDocument(SimpleTemplate.EmptyWordFile))
{
var builder = new DocxDocumentBuilder(docxDocument);
builder
.Tag(SimpleTemplate.ContentTagName,
x => x.Center.Paragraph(z => z.Bold.Text( "Hello Word!" )));
File .WriteAllBytes( string .Format( @"D:\Word.docx" ), docxDocument.ToArray());
}
}
* This source code was highlighted with Source Code Highlighter .
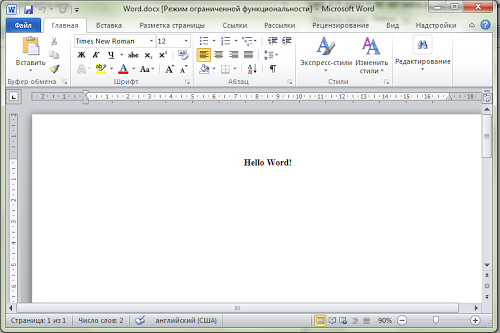
SimpleTemplate.EmptyWordFile is a template that is embedded in the library itself. There is nothing in the template except tags for the header, content, and footer. This template is perfect for test purposes and the generation of simple documents.
Instead of File.WriteAllBytes, which writes the file to disk, an array of bytes can be transferred as FileResult, so that the user immediately receives the generated document.
The attachment hierarchy should help to understand the ideology of working with the library:
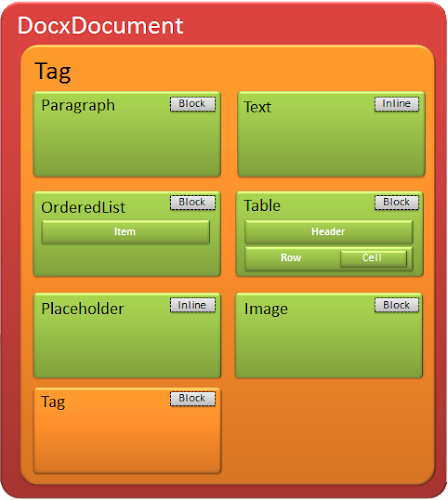
Example 2 Create a contract
It is often required to create a contract or invoice. This example shows a typical script for creating a document. For simplicity, variables are not taken from the database, but are determined by variables.
private static void Main()
{
string customerName = " .." ;
string orderNumber = "4" ;
string itemName1 = "" ;
string itemSumm1 = "5 000" ;
string itemName2 = " " ;
string itemSumm2 = "6 342" ;
string summ = "11 342" ;
using ( var docxDocument = new DocxDocument(SimpleTemplate.EmptyWordFile))
{
var builder = new DocxDocumentBuilder(docxDocument);
builder
.Tag(SimpleTemplate.ContentTagName,
x => x
.Center.Paragraph(z => z.Bold.Text( string .Format( " №{0}" , orderNumber)))
.Right.Paragraph( string .Format( " {0} ." , DateTime .Now.ToString( "dd MMMM yyyy" )))
.Left.Paragraph( string .Format( ", {0}, :" , customerName))
.Table(t => t.Column( "" , 70).Column( ", ." , 30),
r => r.Row(itemName1, itemSumm1)
.Row(itemName2, itemSumm2)
.Row(w => w.Right.Bold.Text( ":" ), w=>w.Center.Bold.Underlined.Text(summ))
));
File .WriteAllBytes( string .Format( @"D:\Word.docx" ), docxDocument.ToArray());
}
}
* This source code was highlighted with Source Code Highlighter .
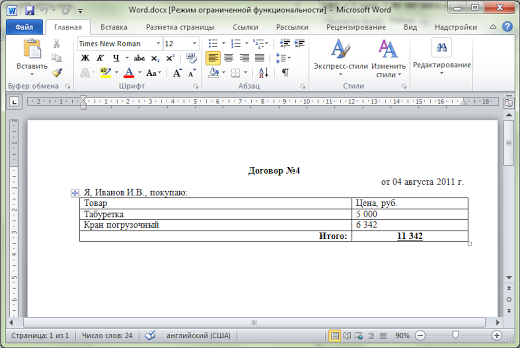
Example # 3 - Modifying the created document
Scenario:
- Create a document signed by Ivanov K.I.
- We send the document for approval
- The electronic document is returned and they say that Petrov A.I. should sign.
- Change the signer in the document
private static void Main()
{
using ( var docxDocument = new DocxDocument(SimpleTemplate.EmptyWordFile))
{
var builder = new DocxDocumentBuilder(docxDocument);
builder
.Tag(SimpleTemplate.ContentTagName,
x => x.Left.Paragraph( " ..." )
.Right.Paragraph(z => z.Placeholder( "SIGNER_NAME" , " .." )));
File .WriteAllBytes( string .Format( @"D:\Word.docx" ), docxDocument.ToArray());
}
using ( var docxDocument = new DocxDocument( File .ReadAllBytes( @"D:\Word.docx" )))
{
var builder = new DocxDocumentBuilder(docxDocument);
builder
.Placeholder( "SIGNER_NAME" , x => x.Text( " .." ));
File .WriteAllBytes( string .Format( @"D:\Word.docx" ), docxDocument.ToArray());
}
}
* This source code was highlighted with Source Code Highlighter .

Example # 4 - Create a document from scratch
private static void Main()
{
using ( var docxDocument = new DocxDocument())
{
docxDocument.AddOpenCloseTag( "CONTENT" );
var builder = new DocxDocumentBuilder(docxDocument);
builder
.Tag( "CONTENT" , x => x.Left.Paragraph( " ..." ));
File .WriteAllBytes( string .Format( @"D:\Word.docx" ), docxDocument.ToArray());
}
}
* This source code was highlighted with Source Code Highlighter .

Example 5 - Unit Tests
Quite a lot of unit tests have been written to the library, with the help of which you can understand the work scenarios even better. Download the source code with tests from
Google Code: fluent-openxml .
Buns
Alexander Zaitsev had fun and made a rather interesting implementation for formatting text:
builder.Tag( "CONTENT" , x => x.Paragraph( " " .Bold() + " " .Italic() + " " .Underlined() + "" ));
* This source code was highlighted with Source Code Highlighter .
This method can be used for text, as an alternative to the Bold property.
Feedback
This library can be better and work with a large number of different specific situations if you give us
feedback .
LinksVideo and slides from the
DotNetConf conference
: Announcement of the OpenSource library for creating and modifying documents in the OpenXml format via the Fluent interface