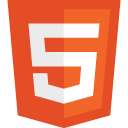
Thanks to the innovations of HTML5, creating Drag and Drop interfaces has become much easier. Unfortunately, these innovations do not yet have extensive browser support, but I hope this will change soon (currently working in Firefox 4+, Chrome and Opera 11.10).
Markup
Immediately I say, the article is written more for beginners than for professionals. Therefore, some points will be described in great detail.
First, we need to create an HTML file with the following content:
')
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title>HTML5 Drag and Drop </title> <link rel="stylesheet" href="/style.css"> <script src="//ajax.googleapis.com/ajax/libs/jquery/1.6.2/jquery.min.js"></script> <script src="/script.js"></script> </head> <body> <form action="/upload.php"> <div id="dropZone"> , . </div> </form> </body> </html>
All the work we will have with the dropZone container. Now add the styles for our document (style.css):
body { font: 12px Arial, sans-serif; } #dropZone { color: #555; font-size: 18px; text-align: center; width: 400px; padding: 50px 0; margin: 50px auto; background: #eee; border: 1px solid #ccc; -webkit-border-radius: 5px; -moz-border-radius: 5px; border-radius: 5px; } #dropZone.hover { background: #ddd; border-color: #aaa; } #dropZone.error { background: #faa; border-color: #f00; } #dropZone.drop { background: #afa; border-color: #0f0; }
In styles, you can notice three states for the dropZone element: during hover, if there is some kind of error and upon successful execution.
Boot script
Now we get down to the most interesting thing - writing JavaScript code. First, we need to create variables, in one of which we place our dropZone, and in the second we specify the maximum file size.
$(document).ready(function() { var dropZone = $('#dropZone'), maxFileSize = 1000000;
Next we need to check if the browser supports Drag and Drop, for this we will use the FileReader function. If the browser does not support Drag and Drop, then inside the dropZone element we will write “Not supported by the browser!” And add the class “error”.
if (typeof(window.FileReader) == 'undefined') { dropZone.text(' !'); dropZone.addClass('error'); }
The next thing we do is to animate the effect of dragging and dropping the file onto the dropZone. We will track these actions with the help of the ondragover and ondragleave events. But, since these events cannot be tracked on a jQuery object, we need to refer not only to “dropZone”, but to “dropZone [0]”.
dropZone[0].ondragover = function() { dropZone.addClass('hover'); return false; }; dropZone[0].ondragleave = function() { dropZone.removeClass('hover'); return false; };
Now we need to write an “ondrop” event handler - this is an event when the overloaded file was dropped. In some browsers, when dragging files into the browser window, they automatically open, so that this does not happen, we need to cancel the standard browser behavior. We also need to remove the hover class, and add the drop class.
dropZone[0].ondrop = function(event) { event.preventDefault(); dropZone.removeClass('hover'); dropZone.addClass('drop'); };
Next we need to add a check for the file size, for this we add the following code line to the ondrop handler:
var file = event.dataTransfer.files[0]; if (file.size > maxFileSize) { dropZone.text(' !'); dropZone.addClass('error'); return false; }
Now we need to write an AJAX request that sends our file to the handler. To create an AJAX request, we will use the XMLHttpRequest object. Add two event handlers for the XMLHttpRequest object: one will show the progress of the file download, and the second the result of the download. We specify the file upload.php as a handler.
var xhr = new XMLHttpRequest(); xhr.upload.addEventListener('progress', uploadProgress, false); xhr.onreadystatechange = stateChange; xhr.open('POST', '/upload.php'); xhr.setRequestHeader('X-FILE-NAME', file.name); xhr.send(file);
Now let's take a look at the download progress and download result. In the function “uploadProgress” there is nothing complicated, just the simplest mathematics.
function uploadProgress(event) { var percent = parseInt(event.loaded / event.total * 100); dropZone.text(': ' + percent + '%'); }
In the stateChange function, we have to check whether the boot process is complete, and if so, it is necessary to check if any error has occurred. The successful request code is “200”, but if the code is different from this, then this means that an error has occurred.
function stateChange(event) { if (event.target.readyState == 4) { if (event.target.status == 200) { dropZone.text(' !'); } else { dropZone.text(' !'); dropZone.addClass('error'); } } }
Writing javascript parts complete.
Server part
All we have to do is write a simple handler that will save the file in the right place for us. I will not go deep into writing a handler, but only give a small example in PHP.
<?php $uploaddir = getcwd().DIRECTORY_SEPARATOR.'upload'.DIRECTORY_SEPARATOR; $uploadfile = $uploaddir.basename($_FILES['file']['name']); move_uploaded_file($_FILES['file']['tmp_name'], $uploadfile); ?>
That's all, I hope the article was useful for you.
Download the source files
here .
Translation and revision of the article:
HTML5 Native Drag And Drop File Upload