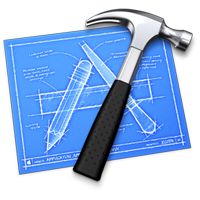
Intro
Before I started learning Objective-C, I programmed first in PHP, then in Python and Ruby. I liked Ruby the most. I liked its simplicity, brevity, but at the same time and power. And about a week ago, I finally managed to start a hackintosh on my computer (I now have Macbook Early 2008 Black). It turned out to be OS X Lion Golden Master. I knew that applications for poppies and iPhones were written in Objective-C, I even tried to learn it, but without OS X it was unpleasant or something. Putting Xcode 4.2 (I’m already a registered iOS developer), I wrote some very simple console applications. And every time I followed a tutorial, or simply tried to write code myself, it occurred to me that, it turns out, Ruby and Objective-C have much in common (although this is logical, since both languages ​​were made under the influence of
Smalltalk ), despite the fact that these two languages ​​have completely different purposes.

So, here I will describe some things that will help Ruby programmers understand Objective-C, for the most part theoretical, without code. I also apologize if some things will not be explained in a completely different way, I came from the world of Ruby.
1. Dynamism
We all know that Ruby is a dynamic programming language. But what about Objective-C? Is it just a superstructure over C? Not really. It turns out that Objective-C is also to some extent a dynamic language. There are many things in it (for example, sending a message) and decisions are made directly at the time of launch, and not at compile time.
2. Object Model
In Ruby, everything is an object. For Objective-C, this is not always true. Since it is a superstructure over C, basic data types (such as
char, float, double, int, struct ) are not objects. However, the Foundation framework represents functional wrappers over them, as well as such seemingly familiar things as strings, mutable arrays, hashes, etc.
')
3. Sending messages
In Ruby, when we call an object method, an implicit message is sent:
object.send :method, args
that is, a method call is converted to sending a message. In Objective-C, something like this happens:
[object method];
This is the sending of the message, not the method call, because if you call a nonexistent method, we will not get any errors at the compilation stage, only at run time (which proves the dynamism of the language). During compilation,
[object method]
converted to a C-shnu function
objc_sendMsg(id object, SEL selector)
.
As farcaller suggests in the comments:clang3 on non-existent methods can give compilation errors
By the way, in C ++ terminology, methods in Ruby and Objective-C are virtual .
4. Lax typing
Every rubist knows that with Ruby he doesn’t have to worry about the types of variables. But not everyone knows that, in principle, with Objective-C too. If we do not know the type of the object, in Objective-C you can simply specify the type of the object id, which means that the variable can take on the value of any type. After all, in fact, id is a pointer to an arbitrary object.
However, this does not work for C-type types, only for Objective-C objects. Although it may be necessary to resort to C types infrequently, many C types have an implementation on Objective-C - NSNumber, NSString, NSArray, etc.
5. Class declarations, object creation, and properties
In Ruby, we can simply define a class, in Objective-C we have to define the description and the implementation of the class itself. Usually the description and implementation are located in different files. In Ruby, we can define any object variable from any method, in Objective-C we must define in advance all variables used within the class.
In principle, objects are created quite easily in both languages. In Ruby, you can simply send a message to class
new
myObject = MyClass.new
in Objective-C, you first need to allocate memory to the object (
alloc
), and then initialize it (
init
,
initWith…
)
MyClass * myObject = [[MyClass alloc] init];
In order to gain access to the instance variables, we must define two methods, the getter and the setter. In Ruby, we can simply write
attr_accessor :var
; in Objective-C, we have to write
type * variable
in the section for describing class variables,
@property(retain) type * variable;
after describing the methods, and
@synthesize variable;
in class implementation.
A small digression: since Objective-C is an add-on to C, we also have to take care of memory. The LLVM compiler supports one interesting feature - Automatic Reference Counting , which takes care of freeing memory. Xcode version 4.2 and higher uses the LLVM compiler by default, that is, you can and do not care about memory management, although, in principle, for a complete understanding of the language it would not be a bad idea to understand such a not entirely simple topic like memory management.6. Protocols and mixins
In Ruby, we can define modules, and then mix them into classes, so something like multiple inheritance is implemented. In Objective-C, there is no such thing specifically. But in it there are protocols. As
Wikipedia says:
A protocol is simply a list of method descriptions. An object implements a protocol if it contains implementations of all the methods described in the protocol. Protocols are convenient because they allow to select common features of heterogeneous objects and transfer information about objects of previously unknown classes.
That is, for example, the method can accept objects that implement the Serializable protocol and be sure that the object responds to the
serialize
message.
7. Categories and extension classes
If we want to extend a class, in Ruby we can simply write:
class String def my_super_method “New string” end end
Thus, we will add the
my_super_method
method to the class (although this is not the only way). In Objective-C, we can do something similar using categories:
@interface NSString (HelloWorld) + (NSString *)helloWorld; @end @implementation NSString (HelloWorld) + (NSString *)helloWorld { return @”Hello, World!”; } @end
Categories are usually saved to files ExtensibleClass + NameCategories - NSString + HelloWorld.m and NSString + HelloWorld.h, and then imported.
Here are just a few things that made it easier for me to understand Objective-C. I hope you were interested. Good luck!I recommend to pay attention to: