When developing cross-platform games and applications, most developers release the Android version of their game either paid or free (with ads), and often the full paid version + lite is free, again with ads.
When developing a game using
Marmalade (formerly Airplay SDK), it became necessary to introduce advertising
(in this article, AdMob is used as an example), and since there is no sensible information, examples, or tutorials, I had to dig myself. As a result, everything was not so difficult.
And so what we need:
- Marmalade SDK 5.0 and above (the 5.0 version was used in this article)
- Android NDK r5 (with the requirements set in the requirements and the NDK_ROOT system variable)
- GoogleAdMobAdsSdk-4.1.0.jar
Create an extension
Go to the directory where Marmelade is installed, a folder with extensions (for example c: \ Marmalade \ 5.0 \ extensions), and create your own folder with the name of the new extension
AdmobAds . It is desirable that the extension was in a directory with other extensions.
Now you need to create a text document
AdmobAds.s4e , which should contain the following:
#include <s3eTypes.h>
functions:
s3eResult InitAds (const char * pub_id) S3E_RESULT_ERROR run_on_os_thread
s3eResult ShowAds () S3E_RESULT_ERROR run_on_os_thread
s3eResult HideAds () S3E_RESULT_ERROR run_on_os_thread
This means that the extension will have 3 methods:
- InitAds () which will accept as input publisher_id
- ShowAds () - show ads
- HideAds () - hide ads
')
Now you need to generate an extension code for Android, to do this, right-click on
AdmobAds.s4e and select
Build Android Extension . After that, template source code for java and cpp, and other auxiliary files will be generated in the source folder.

After the code has been generated, open ... \ AdmobAds \ source \ android \
AdmobAds.java which in turn contains the method described in
AdmobAds.s4e :
/ *
implementation of the AdmobAds extension.
Add android-specific functionality here.
These functions are called via JNI from native code.
* /
/ *
* NOTE:
* be overwritten (unless --force is specified) and is intended to be modified.
* /
import com.ideaworks3d.marmalade.LoaderAPI;
class AdmobAds
{
public int InitAds (final String pub_id)
{
return 0;
}
public int ShowAds ()
{
return 0;
}
public int HideAds ()
{
return 0;
}
}
Attention: the method is not executed in the UI stream, and to display the same Toast, you must add its launch via runOnUiThread:
import com.ideaworks3d.marmalade.LoaderActivity;
import android.widget.Toast;
...
public int InitAds (final String pub_id)
{
LoaderActivity.m_Activity.runOnUiThread (new Runnable () {
@Override
public void run () {
Toast.makeText (LoaderActivity.m_Activity, pub_id, Toast.LENGTH_LONG) .show ();
}
});
return 0;
}
LoaderActivity.m_Activity - the main activity of the application, it can be inherited if necessary.
Now let's check how everything works, double-click on it.
- AdmobAds_android.mkb
- AdmobAds_android_java.mkb
If everything was done correctly then build failed should not occur.
In order to connect the extension to the project, you must perform a couple of steps:
- For example, copy the HelloWorld example
- Add a sub -project (extension) to s3eHelloWorld.mkb
subprojects
{
AdmobAds
}
- Save and open (dependencies will be generated and extension to the project will be linked)
- Add the following changes to s3eHelloWorld.cpp:
#include "AdmobAds.h"
int main ()
{
if (AdmobAdsAvailable ()) {
InitAds ("a14bd815 ...");
}
The
AdmobAdsAvailable () method is automatically generated when an extension is created, and returns true if the extension is available for this platform (that is, in our case for Android it will be true and for iOS, win, etc. will return false).
After compiling GCC ARM (Release), we launch the export tool (Deployment tool), specify the file from the build directory of the deploy_config.py, select from the list of Android platforms, click Deply All. After that we start exporting the application for the selected platforms. If the build is successful, download the apk file to the phone (!) And check

Saw Toast - it means everything is fine, now it remains to connect Admob advertising
Advertise AdmobAds extension
Open and edit the previously described source code for the AdmobAds.java extension and add an advertisement there:
import android.view.ViewGroup.LayoutParams;
import android.view.View;
import android.widget.Toast;
import com.google.ads.Ad;
import com.google.ads.AdRequest;
import com.google.ads.AdRequest.ErrorCode;
import com.google.ads.AdSize;
import com.google.ads.AdView;
import com.ideaworks3d.marmalade.LoaderAPI;
import com.ideaworks3d.marmalade.LoaderActivity;
class AdmobAds
{
private static final int ADVIEW_NOT_INITIALIZED = 1;
private adView adView;
public int InitAds (final String pub_id)
{
LoaderActivity.m_Activity.runOnUiThread (new Runnable () {
@Override
public void run () {
Toast.makeText (LoaderActivity.m_Activity, pub_id, Toast.LENGTH_LONG) .show ();
adView = new AdView (LoaderActivity.m_Activity, AdSize.BANNER, pub_id);
adView.loadAd (new AdRequest ());
LoaderActivity.m_Activity.addContentView (adView, new LayoutParams (LayoutParams.WRAP_CONTENT, LayoutParams.WRAP_CONTENT));
}
});
return 0;
}
public int ShowAds ()
{
if (adView! = null) {
LoaderActivity.m_Activity.runOnUiThread (new Runnable () {
@Override
public void run () {
adView.setVisibility (View.VISIBLE);
}});
} else {
return ADVIEW_NOT_INITIALIZED;
}
return 0;
}
public int HideAds ()
{
if (adView! = null) {
LoaderActivity.m_Activity.runOnUiThread (new Runnable () {
@Override
public void run () {
adView.setVisibility (View.INVISIBLE);
}});
} else {
return ADVIEW_NOT_INITIALIZED;
}
return 0;
}
}
As you can see, there is nothing unusual, a standard example of code for introducing advertising from the AdMob wiki
Run
- AdmobAds_android.mkb
- Edit AdmobAds_android_java.mkb and add the path to GoogleAdMobAdsSdk-4.1.0.jar there
#! / usr / bin / env mkb
# Builder mkb file for the java portion of the AdmobAds extension on android
platform JAVA
files
{
(source / android)
AdmobAds.java
}
librarypath "$ MARMALADE_ROOT / s3e / deploy / plugins / android / android.jar"
librarypath "$ MARMALADE_ROOT / s3e / loader / android / s3e_release.jar"
librarypath "c: /Marmalade/5.0/examples/HelloWorld_java/GoogleAdMobAdsSdk-4.1.0.jar"
option output-name = lib / android / AdmobAds.jar
- Perform AdmobAds_android_java.mkb
Now you need to edit s3eHelloWorld.mkb and add there
deployments
{
android-manifest = AndroidManifest.xml
android-external-jars = GoogleAdMobAdsSdk-4.1.0.jar
}
AndroidManifest.xml you can copy the generated one (next to the .apk file there is an intermediate_files directory) and add there:
<activity android: name = "com.google.ads.AdActivity"
android: configChanges = "keyboard | keyboardHidden | orientation" />
...
<uses-permission android: name = "android.permission.INTERNET" />
<uses-permission android: name = "android.permission.ACCESS_NETWORK_STATE" />
Now we repeat the export procedure, upload to the phone:
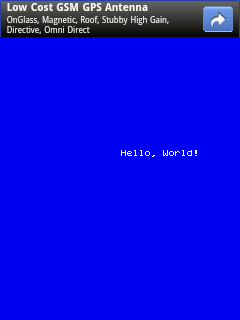
On the android emulator, for some reason, it flies out, apparently not always friendly with marmalade, but this example is tested and works on the phones Nexus S, huawei u8110, huawei ideos u8150, Samsung i5500
The basis was taken information from the following sources
Google AdMob Ads Android FundamentalsEDK tutorialMarmalade documentationAt the request of the guys from Marmalade, they put the source code on their gita (HelloWorld's attention has been slightly modified - added the ability to show / hide ads)
https://github.com/marmalade/admob