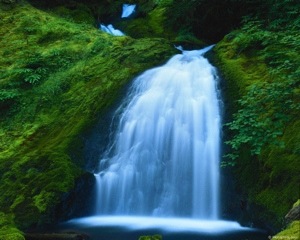
In the current project it became necessary to perform a consecutive series of ajax requests and, at the end - to do something with all their results.
A rather typical task, the pattern of its solution is usually called
waterfall . Implementations of this pattern are for node.js, although some also work in the browser -
async .
But it was not desirable to include the whole module for the sake of one method. Copy / paste also did not do, but for reasons of rather aesthetic. In async, the callback function is passed to the method, but jQuery.Deferred is used everywhere. There is no difference, of course, but my “sense of beauty” did not allow me to “break” the style of the project :)
As a result, I wrote a small utility like jQuery.when
Unlike the jQuery.when arguments, you need to pass not jqxhr, but the functions that return them, otherwise the meaning of the waterfall is lost. Although it will not cause errors, since waterfall accepts any type of argument.
')
Using it is easy.
1. Perform 3 queries in turn and show the results:
$.waterfall( function() { return $.ajax(...) }, function(arg1) { return $.ajax(...) }, function(arg2) { return $.ajax(...) } ) .fail(function(error) { console.log('fail'); console.log(error); }) .done(function() { console.log('success'); console.log(arguments); })
2. We will execute one request, check what it has returned and decide what to do next:
$.waterfall( function() { return $.ajax(...) }, function(arg1) { return arg1 && arg1.answer == 42 ? $.ajax(...) : false; }, function(arg2) { return $.ajax(...) } ) .fail(function(error) { console.log('fail'); console.log(error) }) .done(function() { console.log('success'); console.log(arguments) })
3. waterfall can take any type of argument. false will immediately interrupt the waterfall, other values other than functions and deferred objects will simply be added to the result:
var dfrd = $.Deferred(); $.waterfall( function() { return $.ajax(...) }, $.ajax(...),
That's all. Criticism and advice are welcome.
Githaba code