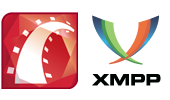
The article will tell you how to receive messages from your Rails application via XMPP (Jabber) and vice versa, manage the application by sending commands via XMPP.
A bit of theory
XMPP (Extensible Messaging and Presence Protocol), previously known as Jabber, is an XML-based, open, free-to-use protocol for instant messaging in near real-time mode. Originally designed to be easily extensible, the protocol, in addition to sending text messages, supports voice, video and file transmission over the network.
')
Unlike commercial instant messaging systems such as AIM, ICQ, WLM and Yahoo, XMPP is a decentralized, extensible and open system. Anyone can open their instant messaging server, register users on it and interact with other XMPP servers. Based on the XMPP protocol, many private and corporate XMPP servers are already open. Among them are quite large projects, such as Facebook, Google Talk, Yandex, VKontakte and LiveJournal.
If you do not have a jabber account (I will call XMPP Jabber, in the old-fashioned way), you can quickly start it on
Jabber.ru or use
Google Talk . There will be no problems with the client for the jabber, almost any “asechny” will suit, for example,
Miranda or
QIP .
What is it for?
I am using message sending:
- To search for broken links. When a user on my site hits a 404 page, I receive a message with the address of this page and the address of the page from which this transition was made.
- For instant information about ordering goods, payment, etc.
- To receive messages from users who do not require a response. For example, when filling out the "wish form".
I give commands to the Rails application:
- To restart the application. This is much faster than climbing the server via ssh and reloading the rails with a long command.
- To add / remove rows in the database. I was too lazy to write a full-fledged interface to work with the database, which very rarely needs to add / delete several records.
Well, you can use a bunch of Rails + XMPP for these and any bolder tasks. For example, uploading files and other content to the server, chatting with users, etc.
What do we need?
We need the
XMPP4R gem. Install:
gem install xmpp4r
Immediately, I note that the heme is very unstable behaves under windows, so it is better to work with it on Linux.
Next, we need a jabber account for our Rails application, i.e. the address from which it will send you messages and receive commands from you. Register an account.
Sending messages
Connection and authentication of the robot (I will call the part of our Rails application responsible for communication).
require "xmpp4r" robot = Jabber::Client::new(Jabber::JID::new("robot@xmpp.ru")) robot.connect robot.auth("password")
I think everything is clear here,
robot@xmpp.ru is the address of the jabber account you registered for the application,
password is the password to it. Now send the message.
message = Jabber::Message::new("you@xmpp.ru", ", !") message.set_type(:chat) robot.send message
Where
you@xmpp.ru is your jabber address. That's all. The message has already emerged from your tray.
Feedback
Now the most interesting thing is that we will send commands to the robot (via the jabber client). To do this, first connect the robot and make it declare its presence and willingness to communicate. Those. the robot will be online, the green bar in the jabber client will be on.
robot = Jabber::Client::new(Jabber::JID::new("robot@xmpp.ru")) robot.connect robot.auth("password") robot.send(Jabber::Presence.new.set_show(nil))
Now, turn on the waiting cycle for incoming messages. In doing so, we will check that the messages come from the correct address, i.e. the robot is not trying to command an attacker.
robot.add_message_callback do |message| # if message.from.to_s.scan("you@xmpp.ru").count > 0 # case message.body # when "hello" message = Jabber::Message::new("you@xmpp.ru", " !") message.set_type(:chat) robot.send message when "restart" File.open(Rails.root.to_s + "/tmp/restart.txt", "a+") end else # message = Jabber::Message::new("you@xmpp.ru", " !") message.set_type(:chat) robot.send message end end
Having received the message
“hello” , the robot will respond with greetings to us, and receiving
“restart” will restart the Rails application (if it works via Passenger).
Final class
In the previous example, the robot received a command to restart the application from us and ... died. It must be revived again by running the appropriate script. Of course, I don’t want to do this every time after reloading the application. Therefore, I propose to run the robot with the application. I wrote a daemon that includes a class that makes it easier to work with XMPP, and also allows the robot to live, regardless of the reboot.
We will
arrange this class in a separate Ruby file, for example,
“xmpp_daemon.rb” , and put it in the
“config / initializers” folder, which will allow it to run along with the rails.
#coding: utf-8 require "xmpp4r" class Xmpp # @@my_xmpp_address = "you@xmpp.ru" # - @@robot_xmpp_address = "robot@xmpp.ru" #- @@robot_xmpp_password = "password" # - @@site_name = "sitename.ru" # , # xmpp- def self.connect @@robot = Jabber::Client::new(Jabber::JID::new(@@robot_xmpp_address)) @@robot.connect @@robot.auth(@@robot_xmpp_password) end # def self.message(text) self.connect message = Jabber::Message::new(@@my_xmpp_address, "[#{@@site_name}]\n#{text}") message.set_type(:chat) @@robot.send message end # def self.listen self.connect @@robot.send(Jabber::Presence.new.set_show(nil)) @@robot.add_message_callback do |message| # if message.from.to_s.scan(@@my_xmpp_address).count > 0 # case message.body # when "hello" Xmpp.message " !" when "restart" Xmpp.message "..." File.open(Rails.root.to_s + "/tmp/restart.txt", "a+") end else # Xmpp.message " !" end end end end Xmpp.listen
Now, from any Rails application controller, we can easily send a message.
Xmpp.message ", !"
Thank you for your attention, I hope this will be useful to someone.
Manageru -
Zakgo -
Enspé