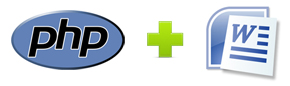
What if you need to create a lot of Word files of the same type, but with different content? For example, fill out forms, receipts.
There are 3 options:
1) use one of the libraries to work with Word documents
2) save the document in docx format, open it with the archiver and inside we will see "\ word \ document.xml" - clean xml, which can be worked through str_replace (thanks to
Enuriru for the hint)
3) use a third-party service that will do most of the work for me
')
The first option was dropped immediately, because it was necessary to create a document with complex formatting, and there was no time or desire to create it manually, prescribing numerous parameters for each line.
The second variant is good and simple when we work with word documents in the .docx format, but unfortunately it does not support the .doc format.
In the process of working out the third option, I came across an interesting solution
LiveDocxBenefits:
- the template file can be created in the usual way through Word
- document presentation in doc, docx, rtf, pdf formats
- no need to bother with the presentation of the Word document via html or XML
- easy connection
- reliability - the service has existed for a long time and there is even a ready-made library from Zend for it
Disadvantages:
- in the free version, the limit on 250 generated documents per day
- the template cannot be changed (for example, it is impossible to generate a table with the number of rows equal to the number of elements in the database)
This is how the file sent by the customer looked like.
Let's get started
1) First you need to mark the template. Open the file and mark up the required fields with special Word's variables mergeField, this is how it is done in Word 2007:
- Insert => Express blocks => Field
A window appears.
Select the field type: MergeField => In the name field, write the name of the variable => Click OK
Thus mark the entire document, you get something like:
Save the document, put in the directory of our application.
2) Let's work with the code
We register on the
LiveDocx website, we get a login and password.
If you are a happy / unlucky owner of Zend Framework, then everything is quite simple, LiveDocx support goes straight out of the box:
// $livDoc = new Zend_Service_LiveDocx_MailMerge(array( 'username' => 'yourusername', 'password' => 'yourpassword' )); // mergeFields Word $livDoc->assign('orderNum',' '); $livDoc->assign('orderDay',date('d', ' ')); // $documentPath = 'contract_bid_for_customer.doc'; $livDoc->setLocalTemplate($documentPath); // $livDoc->createDocument(); $doc = $livDoc->retrieveDocument('doc'); // header("Cache-Control: public"); header("Content-Description: File Transfer"); $fileName = ".doc"; header("Content-Disposition: attachment; filename=$fileName"); header("Content-Type: application/msword"); header("Content-Transfer-Encoding: binary"); echo $doc; die;
If you prefer plain php, you won't be hungry either.
To work, you need the included soap module, in most cases it is enabled by default, but check how you are:
phpinfo ();
SOAP section
You should see Soap client enabled and Soap server enabled.
// WSDL ini_set ('soap.wsdl_cache_enabled', 0); // date_default_timezone_set('Europe/Moscow'); // Soap $soap = new SoapClient('https://api.livedocx.com/1.2/mailmerge.asmx?WSDL'); $soap->LogIn( array( 'username' => 'yourusername', 'password' => 'yourpassword' ) ); // $data = file_get_contents('contract_bid_for_customer.doc'); // .doc $soap->SetLocalTemplate( array( 'template' => base64_encode($data), 'format' => 'doc' ) ); // $fieldValues = array ( 'orderNum' => ' ', 'orderDay' => ' ' ); // c SOAP function assocArrayToArrayOfArrayOfString ($assoc) { $arrayKeys = array_keys($assoc); $arrayValues = array_values($assoc); return array ($arrayKeys, $arrayValues); } // LiveDocx $soap->SetFieldValues( array ( 'fieldValues' => assocArrayToArrayOfArrayOfString($fieldValues) ) ); // $soap->CreateDocument(); $result = $soap->RetrieveDocument( array( 'format' => 'doc' ) ); $doc = base64_decode($result->RetrieveDocumentResult); // SOAP $soap->LogOut(); // header("Cache-Control: public"); header("Content-Description: File Transfer"); $fileName = ".doc"; header("Content-Disposition: attachment; filename=$fileName"); header("Content-Type: application/msword"); header("Content-Transfer-Encoding: binary"); echo $doc; die;
3) At the output we have this file
LiveDocx supports other formats: DOCX, RTF and PDF.
You can read more here:
livedocx.comphplivedocx.org/articles/getting-started-with-phplivedocxblog.zendguru.com/2010/02/13/creating-word-processing-document-using-zend_service_livedocx