@functions @helpers
Most of the helpers in asp.net mvc 3 are extensions to the System.Web.Mvc class.
HtmlHelper.However, it is not always convenient to create a separate static class for 2-3 helpers needed in one view. And it is completely inconvenient to create helpers returning large blocks of html-code: neither syntax highlighting, nor intellectuals, nor other buns.
To solve these problems in Razor there are two great blocks. They allow you to create helpers directly in .cshtml files.
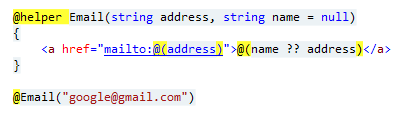
@Functions block
A Razor page is a class that inherits from System.Web.Mvc.
WebViewPage <TModel>. Usually, only his void Execute () method is used, which generates html-code
')
The @functions block allows you to add your methods, properties, fields, etc.
@functions
{
string HelloWorld()
{
return "Hello world" ;
}
string Now
{
get { return DateTime .Now.ToString( "dd MMMM" ); }
}
class SomeClass
{
...
}
}
Such an approach can greatly simplify the code. Suppose we have a certain enum:
enum State
{
Created,
ProcessingStage1,
ProcessingStage2,
Accepted,
Rejected
}
and depending on the status, we need different pictures and color of the frame:
@functions
{
enum State
{
Created,
ProcessingStage1,
ProcessingStage2,
Accepted,
Rejected
}
string GetStateImg( State state)
{
switch (state)
{
case State .Created:
return "created.png" ;
case State .ProcessingStage1:
case State .ProcessingStage2:
return "processing.png" ;
case State .Accepted:
return "accepted.png" ;
case State .Rejected:
return "rejected.png" ;
default :
return "" ;
}
}
string GetStateBorderColor( State state)
{
switch (state)
{
case State .Accepted:
return "green" ;
case State .Rejected:
return "red" ;
default :
return "yellow" ;
}
}
public static int SomeFunc( int a, int b)
{
return a + b;
}
}
as a result, gets the opportunity to do so:
< div class ="border @(GetStateBorderColor(State.Accepted))" >
< img src ="~/images/@(GetStateImg(State.Accepted))" />
...
</ div >
Of course, the
GetStateImg and
GetStateBorderColor methods could be rendered somewhere in the .cs code in some helper class, but it is much more logical to keep the helpers needed by only one view in the same view.
@Helpers block
The
@functions block has a small flaw: it is assumed that this block should contain the usual functions / properties of the class and, as a result, you cannot insert html into the function body using all the joys of Razor.
For this task, the
@helpers block is
used .
declare helper:
@helper Email( string address, string name = null)
{
< a href ="mailto:@(address)" > @(name ?? address) </ a >
}
and use
@Email( "google@gmail.ru" )
And the most wonderful
You can collect frequently used helpers and functions into one .cshtml file, call it, for example,
Helpers.cshtml , put it in the
AppCode and then in any view you can write this:
@ Helpers .Email( "some state" )
PS Of course, the methods must be public and static so that you can use them from other classes.