
Hello!
In this post I want to talk about such an element of the UI Android as
ProgressDialog and in general about the topic of displaying progress in the application, possible implementations and problems.
')
Under the cat you will find some thoughts on the topic + quite a bit of code. Probably, the topic about which I will speak may seem obvious to many, but looking at the same “solutions” in applications from Merket, everything seems to be not so straightforward. Plus, I would be interested to hear your thoughts or best practice on the topic.
Problem or Solution # 0
ProgressDialog - the basic element of Android, is a very popular tool when you need to display the progress of the task. A kosher example of implementing ProgressDialog + AsyncTask can be taken from the user
mike114 here .
Everything would be fine, but there is one major drawback: ProgressDialog
modal . Those. while it is running, the user is unable to interact with the UI. Yes, sometimes this is justified, especially when there are simply no alternative options for action, for example, during the initial logon, but in many situations this is not justified, because makes the user wait for the operation to finish, although he could do something more useful.
There are a lot of examples. Here
, habripuser user
bugrimov uses ProgressDialog to display progress while content is being loaded from the server. At the same time, if for some reason, this does not happen so quickly, the user will have to wait until something returns to him, although instead he could run several parallel tasks and while one loads are loaded already loaded.
Note: ProgressDialog is a cunning element, since it is so familiar and, as a result, understandable to Android users, that they will most likely forgive you for its unreasonable use.Decision number 1. Show nothing at all
Pros:- As a result of this decision, the user is not blocked in any way and is free to do what he pleases. Here, the username rude similarly loads the background image for the list.
Minuses:- The user is in the dark all the time, he doesn’t know whether something is still being done or not, and this can be quite annoying.
- For this and all subsequent decisions without ProgressDialog, it consists in the complicating logic of work, since Now, during the execution of the task, the user can do a lot more actions than with the blocking ProgressDialog, then it will be necessary to take this further into account, for example, to process the restart of the task before completing the same previous one.
In some situations, this may be a normal solution, for example, if you are writing your ICQ-like client, there is no point in showing progress to download the status of the interlocutor, this operation may be transparent to the user.
Those. conclusion that can be made: it is not necessary to reach idiocy in displaying progress, if the user does not need information about the process of performing the operation, you can omit the display of progress.
Decision number 2. Use ProgressBar
ProgressBar is a basic Android component that allows you to display the progress of progress right on View.
In order to get something ala:
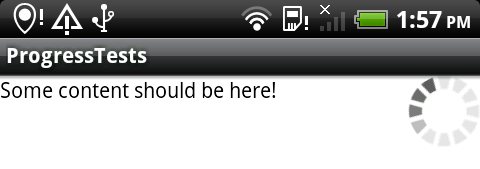
It is enough to create a regular xml resource and define the ProgressBar in it:
<ProgressBar android:layout_width="wrap_content" android:layout_height="wrap_content" style="?android:attr/progressBarStyleInverse" android:layout_alignParentRight="true"/>
Further, this progress bar can be used, like all other components inside layouts:
... progressBar = (ProgressBar) findViewById(R.id.progress_bar); progressBar.setVisibility(View.VISIBLE); ...
This solution is used in the Android Market, if during the installation / update go to the list of installed applications, you can see the progress of the current tasks.
More often, a similar approach is used when loading multiple elements of the same type, first display the empty forms with the ProgressBar inside, and then fill the contents with the loading.
Pros:- Again, that does not block the user.
- And also, in comparison with the first decision, it shows progress, i.e. the user is aware of something happening.
Minuses:- It takes up space on the screen, and on mobile devices every “scrap” of usable surface is worth its weight in gold. Of course, you can hide it when there is no progress, but then the logic of the UI will be broken, in which each element takes its place.
- Another disadvantage in positioning, because Since this progress is tied to the screen, it is not always obvious what to do if you switch to another screen, i.e. show something to the user or not, and most importantly where.
- And another minus is that the position of the progress bar is unconsciously associated with the element next to which it is located, i.e. you need to think through the interface more carefully so that in the end there are no false assumptions that a particular element is being updated, although the whole screen is actually being updated.
- The general minus of all non-ProgressDialog solutions: complication of the operation logic (see Decision # 1).
Decision number 3. Use the ProgressBar inside the Notification Area
This solution is also used in the Android Market, when the user launches the installation of the application, a separate progress is added to the Notification area, allowing the user to go there regardless of the current location.
To add a ProgressBar to the Notification Area, you first need to create an XML resource, for example, like this:
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:paddingLeft="5dip" android:paddingRight="5dip"> <TextView android:id="@+id/text_progress" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentTop="true" android:textColor="@android:color/black" android:textStyle="bold" /> <ProgressBar android:id="@+id/status_progress" android:layout_width="fill_parent" android:layout_height="20dip" android:layout_centerVertical="true" android:progressDrawable="@android:drawable/progress_horizontal" android:indeterminate="false" android:indeterminateOnly="false" /> </RelativeLayout>
Next you need to add a new layout in the Notification Area:
final Intent intent = new Intent(this, Main.class); final PendingIntent contentIntent = PendingIntent.getActivity(getApplicationContext(), 0, intent, 0);
To work with the created progress bar you can use the following code:
As a result, the cell in the Notification Area will look like this:
Pros:- Again, do not block the user.
- As in decision number 2, progress is shown and the user is aware that something is happening.
- This solution is not tied to the windows of the program, i.e. you can go to it from any window; you do not need to change the windows themselves.
- No additional space is required in the window to display progress.
Minuses:- After completion of the display of the notification in the Notification Area (not the completion of it itself, but only the message that it appeared), progress is not visible, i.e. the user does not know something is happening or not. This means that he will have to periodically look there, which may not be very convenient.
- This solution is not sufficiently intuitive.
- The general minus of all non-ProgressDialog solutions: complication of the operation logic (see Decision # 1).
Decision number 4. Use the ProgressBar inside the title bar of the application
This solution is used in many products, for example, in
gReader .
For implementation, you need to create a layout with the contents, in my case it is only the ProgressBar:
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent"> <ProgressBar android:id="@+id/status_progress" android:layout_width="wrap_content" android:layout_height="wrap_content" style="?android:attr/progressBarStyleSmallTitle" android:layout_alignParentRight="true" android:layout_centerVertical="true" android:indeterminateOnly="true" android:visibility="invisible"/> </RelativeLayout>
And in the
onCreate method
, customize the existing title bar with our layout:
Window window = getWindow(); window.requestFeature(Window.FEATURE_CUSTOM_TITLE); setContentView(R.layout.main);
In the end, it should look like this:
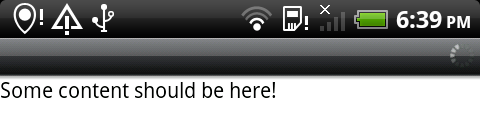
This example is designed for the back of an arbitrary custom layout, i.e. all content defined in this layout will go to the title bar, i.e. not only ProgressBar, if you only need it, then for this there is a simpler possibility:
requestWindowFeature(Window.FEATURE_INDETERMINATE_PROGRESS); setContentView(R.layout.main); setProgressBarIndeterminateVisibility(true);
Pros:- Again, do not block the user.
- Unlike solution number 3, progress is always noticeable.
- As in decisions # 2 and # 3, progress is shown and the user is aware that something is happening.
- No additional space is required in the window to display progress.
Minuses:- Progress is tied to the window, and if you need to show it between different windows, it will need to be provided separately.
- The title bar is inferior in height to the Notification area and therefore less information can be contained in it, otherwise it will become poorly distinguishable.
- This solution is only suitable for title'ov with a standard set of styles. Those. On Activity inside TabHost it can not be attached.
- The general minus of all non-ProgressDialog solutions: complication of the operation logic (see Decision # 1).
Decision number 5. Use all solutions
In my opinion, this is the best solution!
You are not obliged to use only one thing, it all depends on the problem to be solved, as trite as it sounds. Sometimes it's best not to show anything, sometimes ProgressDialog, sometimes variations of the ProgressBar, and sometimes something else. I repeat, it
all depends on the task .
Instead of conclusion
If you make a decision, then do not proceed from considerations of simplicity of development and do not limit yourself to the solutions you know, learn. Think about the users, about how they would be more convenient and understandable, and they will appreciate it.
If you use solutions other than those listed here, please tell us about them, I think many Android developers will be interested in such examples.