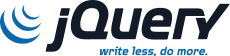
Most recently (May 3) jQuery 1.6 was released and that's what's new in this js-library, let's see.
The most "fun" is that in the new release there are important changes that are incompatible with previous versions of jQuery. As a result, when switching to a new version, it is quite possible that you will have to view and modify an existing code.
Critical changes
Below are just the changes that could bring down the code for older versions.
Data Access in Data Attributes
Now the access to the values in these attributes corresponds to the
W3C HTML5 spec specification, i.e. Keys must be transferred in camel-case format.
<div id="div1" data-test-value="123"></div>
For jQuery 1.5, we wrote this:
var tv = $('#div1').data('test-value'); // => 123 // var d = $('#div1').data(); // => { : 123 } tv = d['test-value']; // => 123
Now for jQuery 1.6
you need to write like this:
var tv = $('#div1').data('testValue'); // => 123 // var d = $('#div1').data(); // => { "testValue": 123 } tv = d.testValue; // => 123
')
.prop (), .removeProp () and .attr ()
Also, the properties of elements are now separated from their attributes. To access the attributes, the .attr () method remains, and to access the properties of an element that can change dynamically (the attribute values do not change), now
you need to do it through .prop (). The simplest example would be the behavior of
<input type="checkbox" />
: in 1.5, calling
.attr('checked')
returned true / false depending on the setting of the flag, but in version 1.6
.attr('checked')
returns a string value written to the attribute, or
undefined
if the attribute is absent, and the state of the flag must be obtained via
.prop('checked')
.
You can play with examples here: at 1.5
jsfiddle.net/dV27a/1 , at 1.6
jsfiddle.net/9zEC9/1.removeProp () should be used with extreme caution, since fraught with glitches in some browsers.In the second example, you can see a bug in Google Chrome, and this browser bug is reproduced without jQuery. The glitch is as follows: if the <input type="checkbox" />
element sets its checked property through its js-object model, then the flag initially changes, but if you delete this property so delete cb.checked
, then changing this property does not result in change the state of the flag.
In connection with the above, some changes have appeared for Boolean attributes (such as checked, selected and similar), now
.attr('checked', true/false)
adds or deletes the specified attribute (and we remember that now this function works with an attribute, not an object property).
Uncritical changes
Extensibility .attr () and .val ()
The new version added the ability to influence the operation of the
.attr()
functions through the
jQuery.attrHooks
object and the
.val()
function through the
jQuery.valHooks
object. For example:
jQuery.attrHooks.selected = { set: function( elem, value ) { if ( value === false ) { jQuery.removeAttr(elem, “selected”); return value; } } };
jQuery.map (Object)
Now the jQuery.map () function can take as its first argument not only an array, but also an object, however, it will return an array of values in any case.
$.map({ f: 1, s: 2 }, function(v, k) { return ++v; });
CSS dependent change
The developers added the ability to change the css-properties of elements with the
.css()
function depending on their value using the "+ =" and "- =" instructions, as was done in
.animate()
.
$("#item").css("left", "+=10px");
Defered objects
Improvements have been made to deferred-objects that are added in 1.5 (for those who don’t know about them yet, I advise you to read
jQuery Deferred Object (detailed description) )
deferred.always ()
When you need a handler to be called for any outcome, you had to write
deferred.then(handler, handler)
before, and now you can write
deferred.always(handler)
deferred.pipe ()
This is a new method and solves several problems at once, firstly, using it, you can create value filters that are passed to the handlers by parameters, secondly, you can build chains of pending tasks (for example, asynchronous requests — this topic was raised in the comments to my previous article and now the developers have provided a solution to this problem).
An example of a filter (for deferred.reject is the same), the second and first parameter in the second handler are the values that the first handler returns.
var defer = $.Deferred(), filtered = defer.pipe(function( value ) { return value * 2; }); defer.resolve( 5 ); filtered.done(function( value ) { alert( "Value is ( 2*5 = ) 10: " + value );
Run:
jsfiddle.net/txzPjAn example of a chain of asynchronous requests, the second handler will be passed parameters with which the second deferred-object will be completed.
var request = $.ajax( '/echo/json/', { dataType: "json", type: 'post', data: { json: '{ "test": 8 }' }, delay: 3 } ), chained = request.pipe(function( data ) { console.log(data); alert('first response'); return $.ajax( '/echo/json/', { type: 'post', data: { delay: 3, json: '' + data.test } } ); }); chained.done(function( data ) { console.log(data); alert('second response'); });
Run:
jsfiddle.net/5gfS8Animation
In addition to improvements in the animation process itself, in version 1.6, you can use deferred-objects tied to the animation. No, the .animate () method (and its abbreviations .fadeOut (), slideUp (), etc.) did not return deferred, instead it was possible to use any jquery object as a parameter of the $ .when () function, and if the selected elements have some kind of animation, then the resulting deferred-object will be completed after the completion of the entire animation.
$(".elements").fadeOut(); $.when($(".elements")).done(function(elements) {
jQuery.holdReady ()
This method allows you to suspend the execution of ready event handlers, it will be used primarily by plug-in developers (at least, the jQuery authors plan this).
jQuery.holdReady( true ); // // - , // , jQuery.holdReady( false );
Selector ": focus"
Now it has become quite easy to find the element on which the focus is set in the document.
Additional features in DOM bypass functions
In the new version they added the ability to transfer the argument to the functions
.find()
,
.closest()
and
.is()
also jquery objects, finally ...
The text of this article is a free retelling of
blog.jquery.com/2011/05/03/jquery-16-released (not a translation, although very close to the text).