The fifth edition of ECMAScript introduced a
strict mode (hereinafter referred to as the Strict Mode article). Strict Mode imposes a layer of restrictions on JavaScript, it separates you from dangerous parts of the language (those parts that are historically, but it is better that they do not exist) and reduces the likelihood of error.
While reading this article, I wrote 38 tests covering all the Strict Mode rules declared in the ES5 specification. You can see how your browser supports these rules
right here .
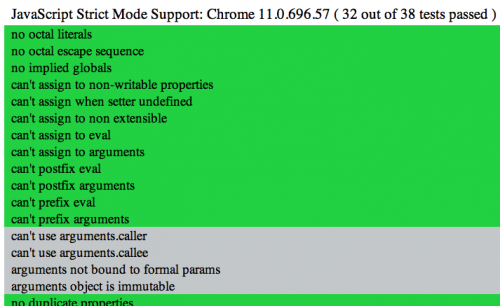
')
The code for each test is presented at the end of the article to help you better understand the specification. You can also perform the test manually by copying the code into the console. All source code is in my
repository .
Firefox 4 already fully supports Strict Mode, and Chrome 11 almost completely. Strict Mode is just around the corner - let's take a closer look at it!
How to enable Strict Mode?
If you add
"use strict"
to the top of your javascript code, then Strict Mode will be applied to all the code:
"use strict"; 012;
Alternatively, you can enable Strict Mode only in a separate function by adding
"use strict"
to the beginning of the body of your function:
012;
Do the internal functions of Strict Mode inherit from external functions?
An internal function declared inside an external one in which Strict Mode is enabled will also have Strict Mode:
var wrapper = function(fn) { 'use strict'; var deleteNonConfigurable = function () { var obj = {}; Object.defineProperty(obj, "name", { configurable: false }); delete obj.name;
It is important to remember that Strict Mode does not apply to “non-strict” (original non-strict) functions that are executed within a strict function (or they are sent to the function as arguments or are executed using call or apply):
var test = function(fn) { 'use strict'; fn(); } var deleteNonConfigurable = function () { var obj = {}; Object.defineProperty(obj, "name", { configurable: false }); delete obj.name;
Why can't I enable Strict Mode in my browser console?
When you execute code in the console or in other consoles, using
"use strict"
outside the function has no effect. This is because most consoles frame your code in eval, so your
"use strict"
not the first expression. This can be circumvented by framing your code into a closure (IIFE), at the beginning of which we will put
"use strict"
(but when I tested this method of enabling Strict Mode I realized that it was rather inconvenient, especially if you were working in the webkit developer tools console it is better to test your code on the page):
(function() { "use strict"; var a; var b; function bar() { x = 5;
What happens if my browser does not support Strict Mode?
Nothing. The
"use strict"
directive is a regular string expression that will be ignored by all JavaScript engines that do not support Strict Mode. This allows you to safely use the Strict Mode syntax in all browsers without any concerns, while browsers with Strict Mode support will use it.
What rules are included in Strict Mode?
The rules are defined in the Strict Mode specification and include restrictions during the "compilation" and interpretation (execution of the script). This is an introductory review (I described each rule with examples in the next paragraph):
ecma262-5.com/ELS5_HTML.htm#Annex_CSyntax Errors Syntax Errors
In most cases, Strict Mode prevents suspicious or illegal code from being executed during the boot process. Octal numbers, duplicate variable names, incorrect use of delete, and attempts to do something like eval and the arguments keyword, using with will result in a SyntaxError exception.
Word this
In Strict Mode, this object will not be updated. This is probably the most interesting part of Strict Mode and the hardest (shocking) for developers. Everyone knows that if the first argument to call or apply is null or undefined, then the value of this function being executed will be a conversion to a global object (for browsers it is a window).
Direct creation of global variables
Not everyone will agree with this, but the indirect creation of a global object is almost always a mistake. In Strict Mode you will be given a red card - ReferenceError.
arguments.caller and arguments.callee
These "useful properties" (from the lane. Never used them) are prohibited in Strict Mode. If you use them in your code, then Strict Mode will throw an exception.
Declare an existing object name
When you create an object with two identical keys, Strict Mode will throw a TypeError exception.
Tests
Here is the source of my Strict Mode tests. Each test suite has a comment referring to the part of the ECMAScript specification it is testing. This version can be executed in “console mode”. Those. You can copy the test, paste it into the console and execute it as is. The same code that works in the “HTML” mode I used to create a test page that I presented to you at the beginning of the article. This source with additional objects in my
github repository . I am sure that there are a couple of mistakes - do not hesitate to send errors!
From the translator: here in the article was a huge piece of code, threw it on pastebinConclusion
Prohibiting access to some of the features of the language to improve the code is a moot point, let's postpone these disputes. In defense of Strict Mode, I want to say that this is an excellent compromise between total changes (which will break backward compatibility) and doing nothing (which will lead to cluttering the language and teach the developers bad).
What else to read
ECMA-262 5th Edition:
The Strict Mode of ECMAScriptAsen Bozhilov:
Strict testerECMAScript 5 Compatibility Chart,
part of Strict mode . This is a great source, part of a large compatibility table developed by Yuri Zaitsev (Juriy Zaytsev aka "kangax")
From the translator. Strict Mode is supported by almost half of all browsers, except for its excellent limitations and immunity to common errors. Strict Mode also has other advantages ( mraleph article). Soon, the non-use of Strict Mode will be a bad tone (similar to requestAnimationFrame vs setTimeout ). Now is the time to start experimenting!