Mobile devices such as smartphones or tablets typically have a touch-sensitive capacitive screen for enhanced user interaction.
Every day, mobile applications are becoming more and more complex. Web developers also need an API to use all the features of the touchscreen. For example, some kind of arcade or fighting game need to press a few buttons, this feature can provide a screen with multitouch support.
Apple introduced its
touch events API in iOS 2.0, soon Android devices also got this feature and the touch events API became the de facto standard. A W3C working group was recently assembled to work on the
touch events specification .
In this article, I will look at the touch events API, which iOS and Android devices provide us with, we will study which applications can be created using the touch events API. The article has a lot of useful examples and techniques that make it easy to write applications with touch events API.
Developments
These three major events are presented in the specification and are supported by many devices:
touchstart : touch the DOM element (analog mousedown).
touchmove : finger movement over the DOM element (analogous to mousemove).
touchend : finger removed from the DOM element (similar to mouseup).
')
Each event includes three lists of touch points (finger lists):
touches : a list of all touch points on the screen.
targetTouches : a list of points on the current item.
changedTouches : a list of fingers participating in the current event. For example, in the event touchend this is the finger that was removed.
Each element of the list is a format object:
identifier : the unique identifier of the finger, which is now on the screen
target : DOM element, which is the target of the event.
coordinates client / page / screen : the point of occurrence of the event on the screen
radius and rotationAngle : an ellipse that describes the shape of a finger
Touch-enabled applications
The touchstart, touchmove, and touchend events provide a powerful enough API to create any touch-based interactions, including all the usual multi-touch gestures — zoom, rotation, and so on.
This example allows you to drag a DOM element using a single point touch:
var obj = document.getElementById('id'); obj.addEventListener('touchmove', function(event) {
Below is an example that displays all the touches on the screen.
Picture clickableHere is his code:
Demos
There are already a large number of applications with multitouch support, one of them is a
canvas-based drawing tool created by Paul Irish
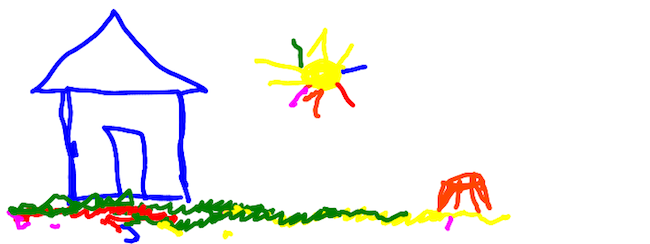
And the
Browser Ninja demo is Fruit Ninja clone using CSS3 transforms, transitions and canvas:
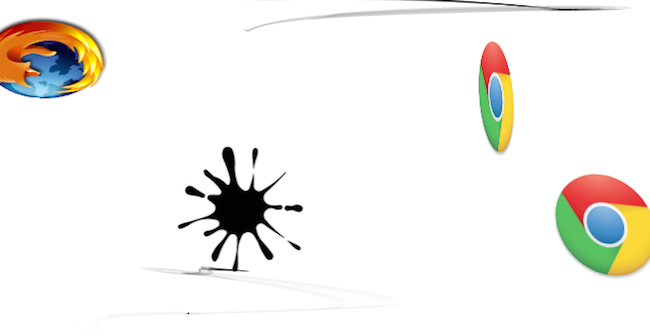
Important Moments
Zoom Prevention
The default settings are not very suitable for multitouch, often your movements with your fingers are perceived by the mobile browser as commands for zoom or scroll.
To disable zoom, you need to add the following meta tag:
<meta name="viewport" content="width=device-width, initial-scale=1.0, user-scalable=no">
For more fine-tuning, check out this article at
www.html5rocks.com/mobile/mobifying.html#toc-meta-viewportScroll prevention
Some mobile devices have default behavior for touch scrolling, such as classic over scroll in iOS, which causes the page to return back if the scroll has exceeded the allowed limits. This is confusing in multi-touch applications, but can be easily disabled:
document.body.addEventListener('touchmove', function(event) { event.preventDefault(); }, false);
Draw neatly
If you are writing a multitouch application that includes complex multi-finger gestures, then be careful with them since you will receive so many events. Consider the previous example in which all the touch points were drawn. You can do it this way:
canvas.addEventListener('touchmove', function(event) { renderTouches(event.touches); }, false);
This method does not scale with the number of fingers on the screen. Instead, you can use the loop to draw all the fingers and get much better performance:
var touches = [] canvas.addEventListener('touchmove', function(event) { touches = event.touches; }, false);
Timers on setInterval are not a very good way to create animations, since it does not synchronize with the browser redraw cycle. Modern desktop browsers provide the function
requestAnimationFrame , which is better suited for cyclical animations - it is more productive and spends less battery. Once it is supported by all modern browsers, using setInterval will be a bad tone.
Using targetTouches and changedTouches
It is important to understand that event.touches is an array of
all fingers that come into contact with the screen, not just those that touch the element DOM. The most appropriate is usually event.targetTouches or event.changedTouches.
Finally, if you are developing for mobile devices, you should know the important points that are described in the
Eric Bidelman article and in the
W3C document .
Device support
Unfortunately, not all devices support touch events in proper quality. I wrote
a diagnostic script that displays information about the support of the touch API by one or another device, as well as the accuracy of the touchmove. I tested Android 2.3.3 on the Nexus One and Nexus S, Android 3.0.1 on the Xoom, and iOS 4.2 on the iPad and iPhone.
In a nutshell, all of these browsers support touchstart, touchend, and touchmove.
The specification describes 3 more events, but none of the browsers support them:
touchenter : finger enter the DOM element.
touchleave : finger leaves the DOM element.
touchcancel : touch aborted (implementation dependent).
All tested browsers also provide touch lists - touches, targetTouches and changedTouches. However, none of the browsers support either radiusX or radiusY or rotationAngle, which define the shape of a finger.
The touchmove event triggered about 60 times per second on all tested devices.
Android 2.3.3 (Nexus)
The Android Gingerbread browser (Nexus One and Nexus S) does not support multi-touch. This is a known
issue .
Android 3.0.1 (Xoom)
The Xoom browser has multitouch support, but it only works on one DOM element. The browser cannot correctly handle two parallel events on different DOM elements. In other words, the following code will handle two parallel events:
obj1.addEventListener('touchmove', function(event) { for (var i = 0; i < event.targetTouches; i++) { var touch = event.targetTouches[i]; console.log('touched ' + touch.identifier); } }, false);
But this is not:
var objs = [obj1, obj2]; for (var i = 0; i < objs.length; i++) { var obj = objs[i]; obj.addEventListener('touchmove', function(event) { if (event.targetTouches.length == 1) { console.log('touched ' + event.targetTouches[0].identifier); } }, false); }
iOS 4.x (iPad, iPhone)
Devices on iOS fully support Touch API.
Developer Tools
In mobile development, it is always easier to prototype on a computer and, if necessary, use a live mobile device for testing. Multitouch is one of all things that is difficult to test on a regular PC, as many PCs do not support multitouch.
The need for testing on mobile devices can increase the development cycle, because each change you have to upload to the server, and then to the device (note. Very doubtful difficulty). Mobile devices lack the necessary developer tools.
The solution to this problem is to simulate a touch event on your PC. Single point touch can be simulated with a mouse. Multitouch can be simulated on devices with mulititouch support, for example, on the new Apple MacBook.
Single point events
If you want to simulate a one-point event on your PC, then try
Phantom Limb , which simulates touch events on pages.
There is also a
Touchable plugin for jQuery that connects mouse and touch events.
Multi-touch events (solution only for MAC)
In order to enable the ability to handle multi-touch events in your browser using multi-touch devices (Apple MacBook or MagicPad). I created
MagicTouch.js, it catches events from your trackpad and converts them into events compatible with the standard.
To get started:
1. Download and install the
npTuioClient NPAPI plugin in ~ / Library / Internet Plug-Ins /.
2. Download the
TongSeng TUIO MagicPad application and start the server.
3. Download the
MagicTouch.js JavaScript library to simulate standard-compliant touch events.
4. Connect magictouch.js and npTuioClient plugin:
<head> ... <script src="/path/to/magictouch.js"></script> </head> <body> ... <object id="tuio" type="application/x-tuio" style="width: 0px; height: 0px;"> Touch input plugin failed to load! </object> </body>
I tested this method only in Chrome 10, but it should work in other modern browsers.
If your PC does not support multi-touch, you can simulate touch events using other TUIO trackers such as
reacTIVision . Detailed information is available on the
TUIO project
website .
Your gestures may intersect with OS gestures. On OS X, you can configure system events in
System Preferences> TrackpadMultitouch events are supported by an increasing number of mobile browsers. I am very pleased that new web applications use this API in full force.
From translatorIn the comments on the original article also skipped the link:
Touching and Gesturing on the iPhoneSuggestions, suggestions, criticism is welcome!