Almost a year ago I
published an article on microframe called 'fat-free' written in PHP. Then this framework and the article itself caused some interest, so I decided to review another PHP microform in the style of the popular Sinatra - Silex project.
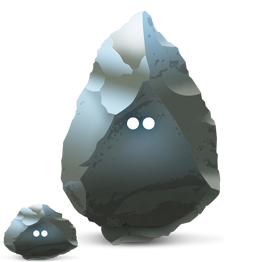
Fabien Potencier, created by Sensio Labs, well-known in PHP, is the author of one of the most popular PHP frameworks for today - Symfony, Silex is a lightweight version of Symfony2 expected by the army of fans (I dare to include it myself).
For its work, Silex uses the key components of Symfony2 in conjunction with an uncomplicated implementation of the design pattern “Dependency
Injection ” in the form of the
Pimple service container, which allows you to separate the logical parts of the code as easily as possible from each other - to make them independent.
Like Symfony2, Silex has an intuitive API that makes the development process quite enjoyable and allows you to add your functionality to the framework itself in just a few steps.
')
Sample application
The simplest application on Silex looks like this:
require_once __DIR__.'/silex.phar';
As you can see - everything is very simple. If the user makes a GET request for / hello / {name}, the code inside the closure function will be executed.
Installation
The framework requires PHP version 5.3 or higher installed in php.ini.
phar.readonly = Off
phar.require_hash = Off .
Installing the framework comes down to downloading the phar archive and connecting it to our bootstrap file (index.php), as shown in the example above.
It is worth mentioning that all requests must be redirected to this file.
If you are using Apache - create .htaccess with such contents in the root directory, next to index.php:
<IfModule mod_rewrite.c>
RewriteEngine On
#RewriteBase /path/to/app
RewriteCond %{REQUEST_FILENAME} !-f
RewriteCond %{REQUEST_FILENAME} !-d
RewriteRule ^(.*)$ index.php [QSA,L]
Routing
Silex allows you to accept routes of unlimited length:
$app->get('/hello/awesome/pink/ponnies', function(){ ... });
Variables can be used in routes:
$app->get('/hello/awesome/pink/{animal}', function($animal) { ... });
Unlimited number of variables:
$app->get('/hello/{impression}/{color}/{animal}', function($impression, $color, $animal) { ... });
Requests, of course, can be POST:
$app->post('/ponnies', function() use ($app) {
And PUT:
$app->put('/blog', function() { ... });
AND DELETE:
$app->delete('/blog', function() { ... });
You can attach a chain of validators to each route:
$app->get('/blog/show/{postId}/{commentId}', function($postId, $commentId) { ... }) ->assert('postId', '\d+')
And also assign a name:
$app->get('/', function() { ... }) ->bind('homepage');
which is useful for generating links to these routes.
Before and after each request, you can execute arbitrary code using the appropriate before / after filters:
$app->before(function() {
Services
Implementing “Dependency Injection” in the form of a Pimple is fairly simple and straightforward.
Create a new application instance:
use Silex\Application; $app = new Application();
and refer to it as an array, assigning it as ordinary variables:
$app['some_parameter'] = 'value';
and already customary closures:
$app['some_service'] = function() { return new Service(); };
For their further use, it is enough to refer to them as array elements:
$service = $app['some_service'];
If the service should be created in a single instance, use the application's share method:
$app['some_service'] = $app->share(function() { return new Service(); });
In this case, the $ app ['some_service'] will be created only once when it is called, and on subsequent calls its instance will be returned.
Extensions
The framework has a flexible system of extensions with the ability to write your own.
An example of connecting and using a far-fetched extension for working with a database:
use Acme\DatabaseExtension; $app = new Application(); $app->register(new DatabaseExtension(), array( 'database.dsn' => 'mysql:host=localhost;dbname=myapp', 'database.user' => 'root', 'database.password' => 'secret_root_password', ));
From the standard extensions are now available:
- MonologExtension - Logger
- SessionExtension - session management
- TwigExtension - template maker
- UrlGeneratorExtension - URL generation
On the way - Doctrine2 and the admin generator.
And this is just the beginning. Adaptation of third-party libraries as extensions for Silex is quite simple, so as soon as a community is formed around this framework, there will be a lot of them.
Results
Micro-frameworks will always occupy a solid place in creating web applications with a simple structure, and the output of a microfamir based on the shoulders of a giant like Symfony2 is certainly a very important event in the PHP world.
Framework Site: http://silex-project.orgUseful links:
Symfony2 websitePhar DocumentationDependency injection