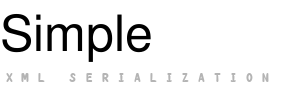
Hello, readers Habrahabr!
This post is inspired by another
post and comment from the respected habrayuser
AnatolyB from there.
')
I think that many are familiar with this library, but, nevertheless, it turned out that it was not yet reflected in the pages of Habra. We will deal with this misunderstanding today.
And, of course, for those who are not familiar with this wonderful library, I recommend you to get acquainted as soon as possible, but I will try to help you with this.
Intro
Simple Framework is an Android virtual machine compatible library for serializing / deserializing Java objects in xml and vice versa.
The main advantage of Simple is a declarative approach to describing the links between classes with their contents and the XML representation. Those. it is enough to set the corresponding attributes to the fields of the class and you can immediately serialize them into XML, and, if desired, back. No additional garbage, such as listing nodes, is needed, everything is very simple and transparent. All work with mapping class fields, enumerating them, getting values, etc. takes on Simple, and helps her in this reflection.
The official
website has everything you need to get started with the library, as well as my favorite type of documentation: documentation with a large set of versatile
examples .
First of all we download the library
from here . Find the jar file and copy it to the lib folder inside our project. Next, we add our jar to the Build Path via “Add JARs ...”, and as a result we get something like this:
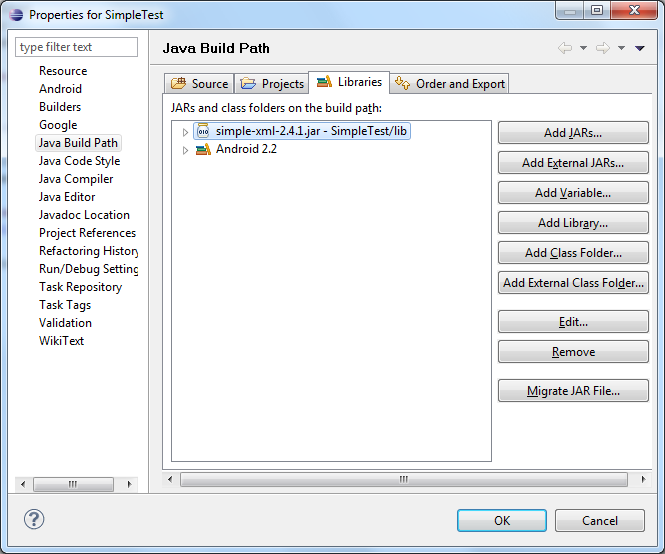
Examples of using
Now we can use Simple in our project. And the first thing we will try to do is convert simple XML into a Java class.
Suppose we have the following XML:
<Pet> <Name>Bobby</Name> <Age>8</Age> <NickName>Lucky</NickName> </Pet>
It represents the essence of "pet", which has mandatory fields: name and age, as well as one optional: nickname.
In order to associate this XML with some class, we need to create a class and add special attributes to it so that Simple can understand what and how we want to do. For our example, the class will look like this:
@Root(name="Pet") public class MyPet { @Element(name="Name") public String name; @Element(name="Age") public int age; @Element(required=false, name="NickName") public String nickName; }
Where:
- Root - points to the root XML element.
- Element - internal fields "Pet".
- required - says that this field may be optional.
- name - indicates the name of the element in the input XML, if this name and the field of the class are the same, then this attribute can be omitted.
If the Pet fields were given attributes, not elements, then the input XML would look like this:
<Pet Name="Bobby" Age="8" NickName="Lucky"/>
And our class would look like this:
@Root(name="Pet") public class MyPet { @Attribute(name="Name") public String name; @Attribute(name="Age") public int age; @Attribute(required=false, name="NickName") public String nickName; }
The deserialization code itself looks like this:
Reader reader = new StringReader(xml); Persister serializer = new Persister(); try { MyPet pet = serializer.read(MyPet.class, reader, false); Log.v("SimpleTest", "Pet Name" + pet.name); } catch (Exception e) { Log.e("SimpleTest", e.getMessage()); }
In the above code, we input two parameters to the input of the Simple serializer: MyPet.class is a pointer to a class with a description of the attributes to deserialize, and reader is a stream containing input XML. As you can see, the code is not complicated at all and very compact.
The code for the inverse transform is also quite simple:
Writer writer = new StringWriter(); Serializer serializer = new Persister(); try { MyPet pet = new MyPet(); pet.name = "Bobby"; pet.age = 8; pet.nickName = "Lucky"; serializer.write(pet, writer); String xml = writer.toString(); } catch (Exception e) { Log.e("SimpleTest", e.getMessage()); }
To comply with the principle of encapsulation, class fields can be wrapped in get'ery and set'ery and Simple will work with them:
@Root(name="Pet") public class MyPet { private String name; private int age; private String nickName; @Attribute(name="Name") public void setName(String name) { this.name = name; } @Attribute(name="Name") public String getName() { return name; } @Attribute(name="Age") public void setAge(int age) { this.age = age; } @Attribute(name="Age") public int getAge() { return age; } @Attribute(required=false, name="NickName") public void setNickName(String nickName) { this.nickName = nickName; } @Attribute(required=false, name="NickName") public String getNickName() { return nickName; } }
If the XML element has its own attributes or nested elements, then it can be declared as a separate class or as a list of them. Modifying our example, we add the essence of “nursery” (nursery), which can contain an arbitrary number of objects “pet” (Pet). Example:
<Nursery> <Pet Name="Bobby" Age="8" NickName="Lucky"/> <Pet Name="Rex" Age="3"/> <Pet Name="Pumba" Age="1"/> </Nursery>
For this example, the only thing we need is to add a class for “Nursery”:
@Root(name="Nursery") public class MyNursery { @ElementList(inline=true, name="Pet") public List<MyPet> pets; }
As you can see, everything is just as simple. The
inline keyword says that “Pet” elements are contained immediately inside MyNursery, without using an intermediate parent element.
The Nursery download code is similar to what we did for Pet:
Reader reader = new StringReader(xml); Persister serializer = new Persister(); try { MyNursery nursery = serializer.read(MyNursery.class, reader, false); Log.v("SimpleTest", "Pets in nursery: " + nursery.pets.size()); } catch (Exception e) { Log.e("SimpleTest", e.getMessage()); }
I think these examples are enough to start a self-study with the library Simple. Moreover, a large number of examples are presented on the official website.
Conclusion
Simple offers solutions, probably, for all constructions that are possible in XML. The following Java features are also supported: inheritance and interfaces.
When using Simple, you can note the following positive and negative sides.
Positive:- Easy to use and understand.
- The amount of code with this approach is minimal.
- Support Android platform.
- Rich features to support various XML constructs.
- The ability to use in non-Android applications, for example, in unit-untied unit tests.
- Apache license, i.e. can be freely used in commercial software.
Negative:- Simple attribute syntax overloads the representation of classes.
- For the Simple operation, the Reflection mechanism is used, and these are costly operations. Therefore, if you are going to use this Framework in a product demanding performance, you should think about the appropriateness of such a solution.
useful links
- Official site.
- Direct link to the documentation.
- Another article on the topic.