Introduction
Why do I need it?

Why not? For example, for general development, or just for interest, and later, perhaps, in order to make sure that F # is not just a language for familiarization.
So, what is next?

')
And then I'll probably start. F # is a multi-paradigm language, the roots of which grow from OCaml, which mixes functional, imperative and object-oriented paradigms in itself, which, in fact, is one of its biggest advantages. Another plus is the integration with the .NET Framework. One more thing is that I will try for a long time not to describe how all this is cool and convenient and I will get to the point: we get the opportunity to use as many as three paradigms, and with a huge amount of goodies packed in a box called .NET Framework. Many tasks are solved much easier and shorter on F # than on the same C #, however, the opposite is also true.
Great, you seem to have promised to get to the point.
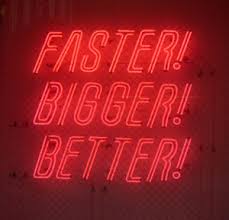
Well, having finished the classic intro, let's move on to the classic HelloWorld!
printfn "Hello, world!"
Yes that's all. Impressive? And I do not, so let's go further.
Closer to the point
We begin with the functional part of F # and, perhaps, the most important keyword:
let . It serves, oddly enough, to determine the identifier and its value.
let someId = 5
There are a couple of reservations:
- The identifier is a name to which any value is attached, and it cannot be changed , the identifier can only be redefined. By the way, this can be encountered not only in F #, but also in all other functional programming languages.
- Value is anything. Function, constant value, another identifier, and everything your heart desires, if only it returns the value or is it
A few examples:
// F# let someFloat = 0.5 // - int let someCalculation : int = 2 + 2 // helloWorld let someString = "Hello" let anotherString = ", World" let helloWorld = someString + anotherString
Now let's go even further, and feel the
functions .
let add xy = x + y let someFunc xy = let val = x + y val / y
First, we discuss the more abstract concept of a function in F #.
fun xy -> x + y
This is an anonymous function, or lambda function. Its arguments are x and y, the operator -> indicates that the function body will be located next. In this example, the body is the expression x + y, and it will also be the return value. For those who have never met with "lambdas" at this moment it is enough to understand that they simply have no name. All functions in F # are somehow represented in a similar way, for example
let add = fun xy -> x + y
Here you can see how functions are actually represented in F #: an anonymous function is bound to an identifier. What was cited as an example above is a simplified syntax.
Consider our example:
let add xy = x + y
Here I would like to speculate about the type of arguments. The fact is that F # automatically determines the type of the arguments and the return value, in this case the arguments can be of any type, if they support the addition operation.
let someFunc xy = let val = x + y val / y
Here the function has a more complex body, which uses an intermediate identifier.
The scope of a code is determined by its indentation , that is, the identifier val will be visible only inside the body of the someFunc function. The return value is val / y
Finally, I will give a small example that shows how functions can be called in code:
let add xy = x + y // 2. - , 2.0 let avg xy = add xy / 2. printfn "%A" add 5. 2. // //printfn "%A" add "Hello" ", World!" printfn "%A" avg 2. 10.
- Why is there a mistake in the second printfn call?
- Because the avg function returns a float type.
- Why does she return float?
“Because we divide the result add xy by float.”
- And here is an error when trying to add lines?
- Given that both arguments to the add function are of type float, respectively, it returns float
- How so?
- Simply in order for avg to return a float, it is necessary that the left and right operands of the division operator are of type float, and F # takes care of this, since the types of arguments in add are not explicitly specified.
I hope we do not say goodbye

This concludes a short speech in F #, not everything is written here, and not completely, and not without flaws. But if everyone likes it, I will try to write further, more and more, because the purpose of this post was not at all to give a comprehensive view of F #, but only slightly open the door to the world of FP, but we will enter into it in subsequent articles .
What to read / see?
For those who do well with English, you can read Beginning F #. Despite the title, the book gives a fairly deep insight into F # and its applications.
You can also see
An Introduction to Microsoft F #And
here is the link to the RIA (thanks to
ApeCoder 's), allowing you to use F # Interactive right in your browser window.
[ Next post ]