The HTML5 specification provides us with several new types of fields, attributes, and other useful functions for forms. One of the most interesting features is the validation of the form by the browser.
Unfortunately, not all browsers support these features and therefore we tried to focus on using these features in Firefox 4.
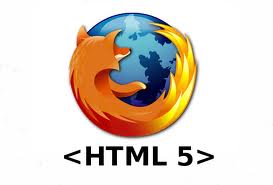
Why form validation by the browser?
The idea of ​​validating the form in HTML5 is to refuse to use JavaScript when checking the content of the fields: is this email address valid? is this field filled in? Do passwords match? Previously, in most cases JavaScript was used for this purpose. You can write your own javascript code or use the javascript library, but this will always be a repeat, boring and error-prone task. With the forms tested by the browser, you no longer have to worry about these tasks, it is enough to write simple HTML.
')
For the user, the validity check done by the browser is a guarantee of quality with the best availability and consistency.
How can I make my form using this feature?
If you use new attributes related to validating the form or new inputs with an internal validation check, the browser will automatically check the validity of the entered data (if they are, of course, supported by the browser). All new input types introduced by HTML5, excluding search and tel, use internal validation.
Firefox 4 is also going to support email and url, and when validating the form, the browser will check if the value entered in the field is a valid email address or url, respectively.
In addition, the attributes below will provide an automatic field validation mechanism:
* required: the field being validated will not validate if its value is empty. This refers to the <input> and <textarea> tags. If it is set for <input type = 'checkbox'>, then the element must be checked (checked) for successful validation. When using it for <input type = 'radio'>, one of the switches must be marked. If set to <input type = 'file'>, then the file will have to be selected.
< input required >
< textarea required ></ textarea >
* pattern: You can also define a regular expression to check the value of an element. This attribute can be used only for some <input> (text, search, tel, email and url). If you are using a pattern, it is highly recommended to use the title attribute to describe the pattern.
< input type ='tel' pattern ='\d\d \d\d \d\d \d\d \d\d' title =" 'XX XX XX XX XX'" >
< input type ='email' pattern =".*@company\.com" title =" email " >
* maxlength: maxlength may already be used in earlier versions of browsers, but it only blocks keyboard input for the <input> tag, so that users cannot enter more characters than the value specified in the maxlength attribute. In Firefox 4, this attribute can also be used for <textarea>. In addition, if the value is set via the value attribute, then the element will become invalid (invalid) if the new value has a length greater than maxlength.
< input maxlength ='10' >
< textarea maxlength ='140' ></ textarea >
How can I refuse to check?
If you have your own system for form validation, you should probably be able to turn it off when the browser can manage form validation itself. However, you may have any reasons why you want to disable the validation of the form by the browser. Serious reasons for this may be that if you want to use some new types of input, but you don’t want to check the validity of their content.
The easiest way to opt-in is to add a
novalidate attribute for the <form> tag. You can also set a
formnovalidate for your submit button. You must prefer formnovalidate over novalidate if you want to have one submit button to check the validity of the form and another without.
How can I define my validation rules?
You can make an element invalid by calling
.setCustomValidity (errorMsg) . This function accepts an error message as a parameter. You can pass an empty .setCustomValidity ('') if you want to remove a custom error.
With this method you can check, for example, whether the password matches in two fields:
<script>
function checkPassword(p1, p2)
{
if (p1.value != p2.value) {
p2.setCustomValidity( ' ' );
} else {
p2.setCustomValidity( '' );
}
}
</script>
<input type= 'password' id= 'p1' >
<input type= 'password' onfocus= "checkPassword(document.getElementById('p1'), this);" oninput= "checkPassword(document.getElementById('p1'), this);" >
How can I change the text of the error message?
Each type of error can have a string that would give a description of the error. For example, if <input> will be required and will not have any meaning, the message will be, "Please fill in this field." Firefox tries to be as smart as possible to display an appropriate error message, but you might want to redefine it in some situations where the rules are very complicated. For example:
<input type= 'email' required pattern= '.*@company\.com' >
You might prefer to have a simple message “Please enter your corporate email address.” Instead of three universal messages depending on the constraint that was not met.
To help with this, you can use the non-standard attribute
x-moz-errormessage , which allows you to define an error message for a given form field. Regardless of the error, if the
x-moz-errormessage is present and is different from the empty string, this value will be used as the error message instead of the default value.
This attribute is non-standard, so must be used carefully. In addition, you should be aware that .setCustomValidity () allows you to indirectly set your own error message.
How can I change the UI of the error that appears?
Unfortunately, there is no way to change the UI. The message is part of the browser, and at the moment it is not possible to change the browser styles from the content. This may change in the future, but it will not be for Firefox 4.
If you think that the message appearing is ugly, you should keep in mind that this is not the final version. The developers will try to improve this later,
see bug 602500 . Any comments are welcome!
New CSS pseudo-classes and default styles
CSS3 UI introduces new pseudo-classes that HTML5 now uses, such as
: invalid: valid: required and: optional . Firefox 4 supports all these pseudo-classes and adds a new pseudo
-class:: -moz-submit-invalid , which is used for the submit button when a form has an
invalid element.
In: invalid has a default style - this is red box-shadow. This default style will be removed when: -moz-ui-invalid is added. You can also easily delete this default style in your style sheets:
:invalid {
box-shadow: none;
}
Examples of the use of form validation .