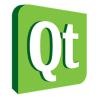
The article describes the interaction of Qt with software interfaces such as Vkontakte API and Phonon, in real-life examples and detailed descriptions.
At the end of the article there is a link to the repository with the source code which you can download and run for free.
Immediately I would like to note that I am not a guru, but the program code can, it would be, better to implement. I will gladly accept your amendments, comments and criticism.
In order not to duplicate information - I will provide links to the Wiki.
Also, probably, it should be noted that the program was tested and developed on the Gnu / Linux / Gentoo platform (KDE) and I did not check it under other platforms and distributions but it should work.
General algorithm:
1. Authorization in the social network through the Vkontakte API (hereinafter VC).
2. Getting a list of your favorite audio recordings.
3. Creating a list of tracks from the list for Phonon.
4. Implementation of the ability to download the selected song.
Authorization
According to the
documentation , for authorization you need to add a component that would allow us to view the web page. Qt has a
WebKit for this . In the component you need to load the page
vkontakte.ru/login.php where the user can go through the authorization procedure. Upon successful authorization, VC will redirect to the page /login_success.html and add the necessary data for us in the
URL , such as session ID, salt and user ID, which we will need to retrieve (parse) from the link.
We connect the necessary modules to the project:
QT += webkit \
network \
xml \
phonon
Since VC returns data to XML (or JSON), we need to connect the XML module in order to simplify our life and not reinvent the wheel.
The network module will allow you to download the desired song from the VC and generally work with the network.
')
Phonon module will allow us to play our video locally or from the network. It also supports playing video files. It is a pleasure to work with this software interface, but about it later.
To work with VK, I have a VKAuth class in whose constructor I pass my application identifier. You can get it by
registering your application in VK.
- #include <QApplication>
- #include <QObject>
- #include "mainwindow.h"
- #include "VKAuth.h"
- int main ( int argc, char ** argv )
- {
- QApplication app ( argc, argv ) ;
- QApplication :: setApplicationName ( "VKaudio" ) ;
- QApplication :: setApplicationVersion ( "0.01a" ) ;
- MainWindow window ;
- VKAuth vkAuth ( "2169954" ) ; // my application id
- QObject :: connect ( & vkAuth, SIGNAL ( unsuccess ( ) ) ,
- & app, SLOT ( quit ( ) )
- ) ;
- QObject :: connect ( & vkAuth, SIGNAL ( success ( QStringList ) ) ,
- & window, SLOT ( slotSuccess ( QStringList ) )
- ) ;
- vkAuth. show ( ) ;
- return app. exec ( ) ;
- }
The VKAuth class sends a success signal upon successful authorization and, as an argument, sends a list of links to songs and an unsuccess signal if the user refuses authorization.
Signal about successful authorization to connect to the slot of the main window of the application (the player itself) which adds the transferred list to the list of audio recordings, this process will be described in more detail later. The user’s denial of authorization signal is connected to the program shutdown slot (output).
- VKAuth :: VKAuth ( QString app, QWidget * parent ) : QWebView ( parent )
- {
- QObject :: connect ( this , SIGNAL ( urlChanged ( QUrl ) ) ,
- SLOT ( slotLinkChanged ( QUrl ) )
- ) ;
- m_app = app ;
- loadLoginPage ( ) ;
- }
As I wrote above, the application identifier and a pointer to the ancestor object are passed to the constructor. The class is inherited from QWebView which implements work with WebKit and allows us to display the required web page to the user. QWebView has a signal that is sent each time the page is changed. Since in the case of successful authorization, VC makes redirection (address change) exactly this signal suits us very well to catch this moment. As an argument, it passes a new link, which we will
parse .
The loadLoginPage () method implements loading the required page for authorization
- void VKAuth :: loadLoginPage ( ) {
- QUrl url ( " vkontakte.ru/login.php" ) ;
- url. addQueryItem ( "app" , m_app ) ;
- url. addQueryItem ( "layout" , "popup" ) ;
- url. addQueryItem ( "type" , "browser" ) ;
- url. addQueryItem ( "settings" , "8" ) ;
- load ( url ) ;
- }
The QUrl class makes working with links very, very convenient.
QUrl breaks the link into parts.



The addQueryItem method adds GET parameters to the link, where the first argument is the name of the parameter and the second is its value.
Table with parameter and possible values:

The settings value is necessary for us in order for the user to allow us access to his audio recordings.
We pass the generated URL to the QwebView :: load () class method and it will load the page for authorization.

Suppose a user has passed authorization - VC redirects, a signal is sent with a new address, and control is given to the slotLinkChanged slot, which is responsible for processing the signal about changing the address.
- void VKAuth :: slotLinkChanged ( QUrl url )
- {
- if ( "/api/login_success.html" == url. path ( ) ) {
- QRegExp regexp ( " \" mid \ " : ([^,] +), \" secret \ " : \" ([^,] +) \ " , \" sid \ " : \" ([^,] + ) \ " " ) ;
- QString str = url. fragment ( ) ;
- if ( - 1 ! = regexp. indexIn ( str ) ) {
- m_mid = regexp. cap ( 1 ) ;
- m_secret = regexp. cap ( 2 ) ;
- m_sid = regexp. cap ( 3 ) ;
- QUrl request ( " api.vkontakte.ru/api.php?" ) ;
- QString sig ( getSig ( m_mid, m_secret, "api_id =" + m_app + "method = audio.get" + "uid =" + m_mid + "v = 3.0" ) ) ;
- request. addQueryItem ( "api_id" , m_app ) ;
- request. addQueryItem ( "method" , "audio.get" ) ;
- request. addQueryItem ( "uid" , m_mid ) ;
- request. addQueryItem ( "v" , "3.0" ) ;
- request. addQueryItem ( "sig" , sig ) ;
- request. addQueryItem ( "sid" , m_sid ) ;
- QNetworkAccessManager * manager = new QNetworkAccessManager ( this ) ;
- m_http = manager - > get ( QNetworkRequest ( request ) ) ;
- QObject :: connect ( m_http, SIGNAL ( finished ( ) ) , this , SLOT ( slotDone ( ) ) ) ;
- }
- } else if ( "/api/login_failure.html" == url. path ( ) ) {
- emit unsuccess ( ) ;
- }
- }
- QByteArray VKAuth :: getSig ( QString mid, QString secret, QString params = "" ) {
- QString str ;
- str. append ( mid ) ;
- if ( ! params. isEmpty ( ) )
- str. append ( params ) ;
- str. append ( secret ) ;
- return QCryptographicHash :: hash ( str. toUtf8 ( ) , QCryptographicHash :: Md5 ) . toHex ( ) ;
- }
In the slot, we check where we were redirected if the redirection was on login_success.html then the authorization was successful (according to the
documentation ).
We create a
regular expression that will help us obtain the necessary data for further work with the VC, namely:
mid, secret, sid.

To get a list of audio recordings, we need to call the
audio.get method.
The Qhttp class simplifies working with the high-level
HTTP protocol .
Pass the required parameters as GET parameters and create a hash (method - getSig).
The Qhttp :: done signal that is sent when the request is completed (the response is received) is connected to the slotDone slot.
- void VKAuth :: slotDone ( bool error ) {
- if ( error )
- emit unsuccess ( ) ;
- else {
- QStringList list ; // list with mp3
- QDomDocument dom ;
- dom. setContent ( m_http - > readAll ( ) ) ;
- QDomElement root = dom. firstChildElement ( ) ; // <response> root element
- QDomNode audioElement = root. firstChildElement ( ) ; // <audio>
- while ( ! audioElement. isNull ( ) ) {
- QString url = audioElement
- . toElement ( )
- . elementsByTagName ( "url" )
- . item ( 0 )
- . toElement ( ) // <url>
- . text ( ) ;
- list. append ( url ) ;
- audioElement = audioElement. nextSibling ( ) ; // next element
- }
- emit success ( list ) ; // load player window with playlist
- hide ( ) ; // hide this window
- }
- m_http - > close ( ) ;
- }
The VKAuth :: slotDone slot reads the received XML and creates a list of links to mp3 songs and then sends a success signal with the same list, closes the join and hides the window. Authorization completed list of songs received.
Phonon
It's very easy to work with Phonon, you need to create a meta object (MediaObject class), create audio (and / or video) output and combine them through Phonon :: createPath.
In the method MainWindow :: appendToList () - we add the list to mp3 files to the list.
- void MainWindow :: appendToList ( ) {
- if ( VKAudioList. isEmpty ( ) )
- return ;
- int index = sources. size ( ) ;
- foreach ( QString string, VKAudioList ) {
- Phonon :: MediaSource source ( string ) ;
- sources. append ( source ) ;
- }
- if ( ! sources. isEmpty ( ) )
- metaInformationResolver - > setCurrentSource ( sources. at ( index ) ) ;
- }
Download selected song
To download a song, you need to select it and click Download at the top of the program window, a dialog will appear in which you need to choose a place to download the file, naturally you should have rights to create (write) a file.
The button click signal is connected to the MainWindow :: slotDownload () slot.

- void MainWindow :: slotDownload ( ) {
- QString path = QFileDialog :: getExistingDirectory ( this , "Save to ..." ) ;
- if ( ! path. isEmpty ( ) ) {
- m_file = new QFile ;
- int item = musicTable - > currentRow ( ) ;
- Phonon :: MediaSource src = sources. at ( item ) ;
- m_pSrcUrl = new QUrl ( src. url ( ) ) ;
- m_file - > setFileName ( path + "/" + musicTable - > item ( item, musicTable - > currentColumn ( ) ) - > text ( ) + ".mp3" ) ;
- if ( m_file - > open ( QIODevice :: ReadWrite ) ) {
- m_pTcpSocket = new QTcpSocket ( this ) ;
- m_pTcpSocket - > connectToHost ( m_pSrcUrl - > host ( ) , 80 , QIODevice :: ReadWrite ) ;
- connect ( m_pTcpSocket, SIGNAL ( connected ( ) ) , this , SLOT ( slotConnected ( ) ) ) ;
- connect ( m_pTcpSocket, SIGNAL ( readyRead ( ) ) , this , SLOT ( slotReadyRead ( ) ) ) ;
- connect ( m_pTcpSocket, SIGNAL ( error ( QAbstractSocket :: SocketError ) ) ,
- this , SLOT ( slotDownloadError ( QAbstractSocket :: SocketError ) )
- ) ;
- connect ( m_pTcpSocket, SIGNAL ( disconnected ( ) ) , this , SLOT ( slotDisconnected ( ) ) ) ;
- } else
- QMessageBox :: warning ( this , "Warning" , "File open Err!" ) ;
- }
- }
After choosing a directory and successfully opening (creating) a file of a song that has not yet been downloaded, you need to send a request to the VC server to “give” the song to us. This will help us QtcpSocket which implements and greatly simplifies working with TCP / IP. After successfully connecting to the VC server, send an HTTP request to it:
- void MainWindow :: slotConnected ( ) {
- m_pTcpSocket - > write ( QString ( "GET" + m_pSrcUrl - > path ( ) + " \ r \ n " ) . toAscii ( ) ) ;
- }
To which he gives us the contents of the song that we need to write to the file:
- void MainWindow :: slotReadyRead ( ) {
- m_file - > write ( m_pTcpSocket - > read ( m_pTcpSocket - > bytesAvailable ( ) ) ) ;
- }
After the VC returns the entire contents of the song, it closes the connection, and it remains for us to close the file.
- void MainWindow :: slotDisconnected ( ) {
- m_file - > close ( ) ;
- m_pTcpSocket - > close ( ) ;
- }
As promised, at the beginning of the article, the link to the repository (
Mercurial ):
https://bitbucket.org/denis_medved/vkaudioMany thanks to Max Schlee and bhv for the wonderful book “Qt 4.5: Professional C ++ programming” and of course to the entire open community.
upd :
Instead of outdated QHttp and QTcpSocket, QNetworkAccessManager is used.