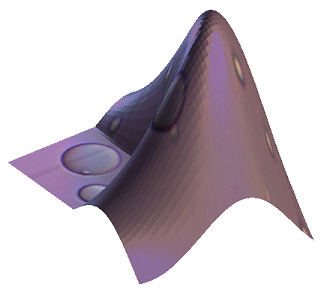
In this topic, I will give an alternative approach to the problem solved by
VasG comrade
here . As noted in the comments, the problem of detecting roundness in the image could be solved using the MATLAB Image Processing Toolbox, which I did. MATLAB makes me happy with very strong documentation with lots of good examples; as well as the convenience of the m-language, due to which the implementation time of computing solutions is greatly reduced. Of course, there are also disadvantages - in particular, the algorithms work slowly - but for this task it is not essential. I note only that it is quite simple to get C code from m-language, which will work much faster.
I tried to write from scratch, based on the formulation of the problem and not using the algorithmic developments of
VasG . Naturally, due to the simplicity of the problem, the solutions are very similar. The MATLAB help on Image Processing Toolbox revealed a set of demos devoted to object detection and, in particular, the search for circular forms, which helped me a lot. The following is the code of the resulting algorithm in m-language:
img = imread('kkk.jpg');
grayimg = rgb2gray(img);
grayimg = imadjust(grayimg);
bw = edge(grayimg,'canny', 0.15, 2);
bw = imfill(bw,'holes');
se = strel('disk',1);
bw = imopen(bw,se);
[B,L] = bwboundaries(bw);
stats = regionprops(L,'Centroid','EquivDiameter');
figure, imshow(img)
hold on
for k = 1:length(B)
boundary = B{k};
radius = stats(k).EquivDiameter/2;
xc = stats(k).Centroid(1);
yc = stats(k).Centroid(2);
theta = 0:0.01:2*pi;
Xfit = radius*cos(theta) + xc;
Yfit = radius*sin(theta) + yc;
plot(Xfit, Yfit, 'g');
text(boundary(1,2)-15,boundary(1,1)+15, num2str(radius,3),'Color','y',...
'FontSize',8);
end
Note that there are only 30 lines. Of these, half implements a beautiful result output. Let's break the code down.
img = imread ('kkk.jpg'); - open our image. I used an image from the
VasG article.
grayimg = rgb2gray (img); - translate the image in shades of gray.
grayimg = imadjust (grayimg); - contrasted.
bw = edge (grayimg, 'canny', 0.15, 2); - select the border method Canny. The
edge function offers the possibility of using the Sobel, Roberts, Prewitt, and some other algorithms, but I, like
VasG , chose Canny because he, I think, works best. Parameters were chosen empirically to highlight the boundaries of all objects in the image.
bw = imfill (bw, 'holes'); - we fill in the closed areas on the image.
Next, you need to get rid of thin lines.
se = strel ('disk', 1); - we form a structural element, a cross 3 by 3.
bw = imopen (bw, se); - morphological discovery, thin lines disappear.
[B, L] = bwboundaries (bw); - This function produces two results.
B is a cell array of size P x 1, where P is the number of objects in the image. The elements of array B are matrices of size Q x 2, where Q is the number of pixels belonging to the boundary of the corresponding object. The rows of the Q matrix are the coordinates of these boundary pixels.
L is a matrix whose size is equal to the size of the image containing non-negative numbers that correspond to closed regions. The region with the number k on the matrix L is denoted by the elements with the value k. The background corresponds to 0.
stats = regionprops (L, 'Centroid', 'EquivDiameter'); - this function creates an array of
stats structures, the element fields of which
Centroid and
EquivDiameter contain, respectively, the coordinates of the center of the area and (in our case) the diameter of the area.
Actually the problem is solved. Further output of the results is realized.
The results are similar to those obtained by
VasG up to coefficients of some functions. The time for implementation was spent about the same as for the design of this article, about an hour.
VasG , as far as is clear from the article, spent an order of time more. I have quite a lot of experience with MATLAB, but I think that even if a person is not familiar with m-language, in a working day you can figure out the system and write a similar implementation. Thus, there is a high rate of implementation of solutions to applied problems in the MATLAB system. I advise
VasG , as well as other scientists and engineers to evaluate the MATLAB system in action!
upd. Replaced a pair of imerode + imdilate to imopen. Thank you
ScayTrase .