The second part ofForeword
Those of you who use the social network VKontakte and subscribed to the official
Habra page in it, noticed that all the new topics from the main page appear on the page as links:
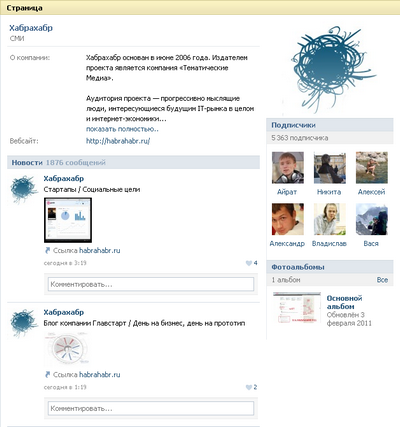
So, if you have your own blog and you want to publish the same link messages automatically on your personal page - the topic may be of interest to you. Today we will try to publish simple links of the message, and then add to them the "previews" of the picture.
Implementation
So, to work, we need PHP with the curl module connected. To interact with the VKontakte site, we will need to go through authorization on it, as well as receive the value of the unique variable posthash, which is transmitted when each record is posted on your wall.
')
Consider the simplest authorization function:
function _auth( $cookies ) { $e = urlencode('my@email.ru');
As the only variable of the function, we pass the path to the file with cookies, where we save the authorization data. In the future, when entering our page, we will check whether we are authorized and if not, then re-launch this function.
Now we need a function to get the id of the current user, the value of his posthash variable, as well as the value of the id variable from the handlePageParams block, which determines which user is currently viewing the page - if it is 0, then we are not authorized and need to refer to the above functions. So:
function _params($cookies) { $c = curl_init(); curl_setopt($c, CURLOPT_HEADER, 1); curl_setopt($c, CURLOPT_RETURNTRANSFER, 1); curl_setopt($c, CURLOPT_REFERER, 'http://vkontakte.ru/settings.php'); curl_setopt($c, CURLOPT_FOLLOWLOCATION, 1); curl_setopt($c, CURLOPT_USERAGENT, 'Mozilla/5.0 (Windows; U; Windows NT 6.1; ru; rv:1.9.2.13) Gecko/20101203 Firefox/3.6.13'); curl_setopt($c, CURLOPT_COOKIEJAR, $cookies); curl_setopt($c, CURLOPT_COOKIEFILE, $cookies); curl_setopt($c, CURLOPT_URL, 'http://vkontakte.ru/'); $r = curl_exec($c); curl_close($c); preg_match_all('/"post_hash":"(\w+)"/i', $r, $f1); preg_match_all('/"user_id":(\d+),/i', $r, $f2); preg_match_all('/handlePageParams\(\{"id":(\d+),/i', $r, $f3); return $f = array( 'post_hash' => $f1[1][0], 'user_id' => $f2[1][0], 'my_id' => $f3[1][0]); }
As a result, we get an array of three variables that we need to work. Now it remains to implement the function of creating a message links. The al_wall.php file is responsible for the publication of messages on your wall, which has a lot of received parameters and, depending on each, can create different messages. The following parameters will be the most important for us:
- act - the actual action for this php file, we pass the value of post
- hash - the same post_hash that we received earlier
- message is our message, no longer than 255 characters, otherwise a note will be created
- note_title - the name of the note, if above you have exceeded the limit of characters
- status_export - the parameter that determines “Export to Twitter”, if your account is connected to VKontakte
- to_id - user id on which wall we publish the message
- type - so far two possible values ​​have been found, all - publication on your wall, feed - publications in your News section (“What's new” section) media_type - message type, set share to get the link
- url - the url of the link transmitted by us
- title - the name of your link, the limit of 81 characters
- description - a pop-up description of the link, here you can transfer, for example, the first lines of your news, a limit of 255 characters
Based on this data, we will write a message creation function:
function _status($cookies, $hash, $url, $message, $title, $descr, $id) { $u = urlencode($url); $m = urlencode($message); $t = urlencode($title); $d = urlencode($descr); $q = 'act=post&al=1&hash=' . $hash . '&message=' . $m . '¬e_title=&official=&status_export=&to_id=' . $id . '&type=all&media_type=share&url=' . $u . '&title=' . $t . '&description=' . $d; $c = curl_init(); curl_setopt($c, CURLOPT_HEADER, 0); curl_setopt($c, CURLOPT_HTTPHEADER, array('X-Requested-With: XMLHttpRequest')); curl_setopt($c, CURLOPT_RETURNTRANSFER, 1); curl_setopt($c, CURLOPT_POST, 1); curl_setopt($c, CURLOPT_REFERER, 'http://vkontakte.ru/id'.$id); curl_setopt($c, CURLOPT_FOLLOWLOCATION, 1); curl_setopt($c, CURLOPT_USERAGENT, 'Mozilla/5.0 (Windows; U; Windows NT 6.1; ru; rv:1.9.2.13) Gecko/20101203 Firefox/3.6.13'); curl_setopt($c, CURLOPT_POSTFIELDS, $q); curl_setopt($c, CURLOPT_COOKIEJAR, $cookies); curl_setopt($c, CURLOPT_COOKIEFILE, $cookies); curl_setopt($c, CURLOPT_TIMEOUT, 15); curl_setopt($c, CURLOPT_CONNECTTIMEOUT, 15); curl_setopt($c, CURLOPT_URL, 'http://vkontakte.ru/al_wall.php'); $r = curl_exec($c); curl_close($c); return $r; }
The function will return the response of the VK server to us - this will be either the error text or the last 10 messages from the wall that can be processed at your discretion. Actually, the work with the VKontakte server is finished, it remains to write a general function that will check the authorization, receive variables and create a message.
function vkPost($url='http://habrahabr.ru/', $message='message', $title='title', $descr='descr') { $o = 'aqwdhfyrfd.txt'; $h = _params($o, 'http://vkontakte.ru/id1', true); if($h['my_id'] == 0) { _auth($o, $d, true); $h = _params($o, 'http://vkontakte.ru/id1', true); } if($h['my_id'] != 0) { $r = _status($o, $h['post_hash'], $url, $message, $title, $descr, $h['user_id']); $c = preg_match_all('/page_wall_count_all/smi',$r,$f); if( $c == 0 ) { return false; } else { return true; } } }
Integration capabilities
Now you can use it anywhere, on any engine. Or create a separate php file through which to publish any links with your description on your wall. As an example, I will show the integration with the Wordpress engine, where when you publish a blog entry, you will automatically publish a link to it. So, you need to transfer all the above functions to functions.php, which is in the directory with your theme, if it is not there, then create it. Then we will add the following function to it and define it as a hook:
function wp_vk_post_add($post_ID) { $post = get_post($post_ID); $title = $post->post_title; $link = get_permalink($post_ID); $descr = $post->post_content; $vkont = get_post_meta($post_ID, 'vkontakte', true); if(mb_strlen(trim($descr), 'UTF-8') >= 250) { $descr = strip_tags($descr); $descr = mb_substr($descr,0,250, 'UTF-8').'...'; } $message = ' ' . $title; if(mb_strlen(trim($message), 'UTF-8') >= 250) { $message = mb_substr($message,0,250, 'UTF-8').'...'; } if(mb_strlen(trim($title), 'UTF-8') >= 78) { $title = mb_substr($title,0,78, 'UTF-8').'...'; } if($vkont != '1') { $status = vkPost($link, $message, $title, $descr); if($status) { update_post_meta($post_ID, 'vkontakte','1'); } else { update_post_meta($post_ID, 'vkontakte','0'); } } return $post_ID; } add_action('publish_post', 'wp_vk_post_add');
A little bit of explanation. The fact is that the publish_post action in WordPress is practiced not only when you click the Publish button in the admin area, but every time you save a post. To receive a link each time you edit your post after publication is certainly not comme il faut. Therefore, if a link is successfully published, the user field vkontakte with the value 1 is added to our post, and its presence is checked before the publication - if it exists and contains 1, then the vkPost function is skipped.
Conclusion
This is how we got the opportunity to interact with VKontakte and be more interactive. Of course, it would be more correct to arrange all the utility functions in a separate class, combine curl's initialization, and then just connect this class in the work, but it’s not difficult to do it yourself, but the goal was to show how this can be done. You also need to remember that VKontakte is unlikely to miss your request without using a proxy server. Later you can add and insert images into this link, but this is the story of another topic.
It is also worth noting that al_wals.php will request an additional captcha parameter if your script sends the statuses too hard or returns “Access error” altogether - so this method is unlikely to help you in sending spam.
Here is an example:
