The social network VKontakte provides ample opportunities for integration with third-party sites. Basically, these features are represented by ready-made widgets. However, there is also the
Open API , which allows not only to authorize the user, but also to perform any method available to VKontakte applications (
VKontakte API ).
Let's try to create a small game application using the Open API features. We will train on the game "Memory", the essence of which lies in the fact that it is necessary to clear the playing field, revealing 2 identical pictures each. As pictures we will use photos of the player’s friends.
Getting Started
First of all, you need
to create a new application , indicating its name and type.
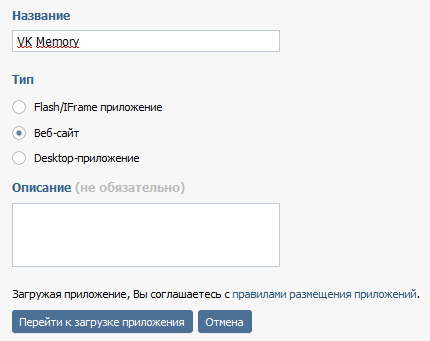
')
After that, go to the settings of the application and specify the address of the site and the base domain.

Implementation
Create an HTML page that will contain several blocks:
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en" lang="en"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8"/> <title>VK Memory</title> <script type="text/javascript" src="./js/jquery.min.js"></script> <script type="text/javascript" src="./js/jquery-ui.min.js"></script> <script type="text/javascript" src="http://vkontakte.ru/js/api/openapi.js"></script> <script type="text/javascript" src="./js/jquery.pnotify.min.js"></script> <script type="text/javascript" src="./js/jquery.blockUI.js"></script> <script type="text/javascript" src="./js/general.js"></script> <link rel="stylesheet" href="./css/styles.css" type="text/css"/> <link rel="stylesheet" href="./css/ui-lightness/jquery-ui-1.8.9.custom.css" type="text/css"/> <link rel="stylesheet" href="./css/jquery.pnotify.default.css" type="text/css"/> </head> <body> <div id="content"> <div id="header"> VK Memory </div> <div class="flash-notice" style="display: none" id="login-bar"> <a href="#" id="vk-login"></a> </div> <div class="flash-success" style="display: none" id="logout-bar"> <a href="./index.html" id="new-game"> </a>, <a href="#" id="game-scores"> </a> <a href="#" id="vk-logout"></a>. </div> <div class="flash-error" style="display: none; text-align: center" id="stats"></div> <div id="game-container"></div> </div> <div id="scores-dialog" style="display: none"></div> </body> </html>
Assignment of blocks:
- div # login-bar - contains a link to authorize the user
- div # logout-bar - contains links to start a new game, view statistics and exit
- div # game-container - a container for the playing field
The important point is to connect a JavaScript file containing Open API methods:
<script type="text/javascript" src="http://vkontakte.ru/js/api/openapi.js"></script>
The main functionality of our application will be located in the general.js file, so let's move on to its consideration.
Open API Initialization
An important part of the script is the initialization of the API, which consists in calling the VK.init method, into which an object containing at least one field is transferred — apiId — application ID (it is displayed on the application settings page):
VK.init({ apiId: 2151186 });
Authorization
To authorize a user, add a handler for the a # vk-login link, which will call the VK.Auth.login method. The first parameter is the callback function, and the second is the necessary user settings for the application, which are the list of required access levels. In our case, you only need access to the list of friends, so the handler for the link will look like this:
$('a#vk-login').click(function(event){ event.preventDefault(); VK.Auth.login(null, VK.access.FRIENDS); });
Session termination
In order for the user to terminate the session, we will add a handler for the link a # vk-logout, which will call the VK.Auth.logout method. The first parameter is the callback function, which in our case will update the page:
$('a#vk-logout').click(function(event){ event.preventDefault(); VK.Auth.logout(function(){ refreshPage(false); }); });
Event Tracking
In order to go to the game (in our case it is to refresh the page), after the user has logged in, you must subscribe to the auth.login event. We will also subscribe to the auth.sessionChange event, it will be generated when the data associated with the user authorization changes. This is necessary in order to go to the game if the user is already authorized before and only confirms access to the list of friends. To add an event handler, you must call the VK.Observer.subscribe method, which takes two parameters — the event to which you want to subscribe, and the callback function — the event handler:
VK.Observer.subscribe('auth.login', function(response){ refreshPage(true); }); VK.Observer.subscribe('auth.sessionChange', function(response){ refreshPage(true); });
The refreshPage method refreshes the page if the user has not been previously successfully authorized:
function refreshPage(checkLogged) { if((checkLogged && !isLogged) || !checkLogged) { location.href = 'index.html'; } }
Collection of initial data
To check the current status of the user there is a method VK.Auth.getLoginStatus. We enable it to determine whether the user is authorized, and to hide / show the necessary blocks from the above. Also, if the user is already authorized, we will immediately move to the top of the game. One parameter is passed to the VK.Auth.getLoginStatus method - a callback function, to which, in turn, an object containing the user's session data is passed:
VK.Auth.getLoginStatus(function(response){ if(response.session) {
To call any VKontakte API method, use the VK.Api.call method, which takes three parameters - the name of the method, an object representing the parameters passed to the specified method, and a callback function to which the result of the method call will be passed. To get the list of friends, call the friends.get method. The method has no required parameters and in the case of a call without parameters it will return a list of all friends. Since we need photos of the user's friends, we pass in the method the fields parameter with the value 'photo_rec', which corresponds to a square photo with a side of 50 pixels:
VK.Api.call('friends.get', { fields: 'photo_rec' }, function(data){
In the event of an error, the data object will contain an embedded error object containing information about the error. Error code 7 indicates that the application does not have enough rights, that is, in our case, that the user did not allow access to the list of friends.
If the method call is completed successfully, the data object will contain a response field, which is an array of objects, each of which represents information about one of the user's friends.
After the list of friends is received, we select from it those who have a given photo (that is, it is not a standard picture with a question mark) and proceed to initializing the game:
VK.Api.call('friends.get', { fields: 'photo_rec' }, function(data){ if(!data.error) { if(data.response.length > 0) { data.response.shuffle(); for(i = 0; i < data.response.length; i++) { var friend = data.response[i]; if(friend.photo_rec && friend.photo_rec.indexOf('images/question_c.gif') == -1) { photos.push({ photo: friend.photo_rec, id: friend.uid }); } } } initGameField(); } else { if(data.error.error_code == 7) { isLogged = false; $('div#login-bar').show(); $('div#logout-bar').hide(); $.unblockUI(); } else { showError(data.error.error_msg); } } });
Preparing gameplay
Preparing the gameplay is that we randomly select the required number of photos (if the user doesn’t have enough friends, inform him about it) and form a table that is filled with the "reverse side" of the photos. For each cell-photo, we add a handler, which when you click on a photo:
- if two photos are already open, close them
- displays the "front side" of the photo assigned to this cell
- if one photo is already open and it coincides with the current one, then clears both cells (successful move)
In the case of a successful move, the VKontakte API getProfiles method is called, which returns information about the specified users. The list of users for whom you want to get information is passed as a parameter to uids. Calling this method is used to display the name and link to the user profile that was successfully guessed.
If the entire field is cleared, we save the current result (the number of steps for which the field was cleared) to the high score table. To do this, use the setUserScore method, which takes a single parameter, the user record:
VK.Api.call('setUserScore', { score: stepsCount }, function(data){ if(data.error) { showError(data.error.error_msg, false); } });
It is necessary to consider that in our game the best result is a smaller result, therefore ideally the described method is not quite suitable for saving the results, because when the maximum possible number of records is reached, the best results will be superseded by the worst.
List of champions
To get the table of records, use the getHighScores method, which has no parameters. The method returns an array of objects, each of which contains information about the user and his record.
So that the user can see the table of records, add a handler reference a # game-scores, which will call the above method, create a table of results and show it in the dialog box:
$('a#game-scores').click(function(event){ event.preventDefault(); VK.Api.call('getHighScores', {}, function(data){ if(!data.error) { var scoresTable = ''; if(data.response && data.response.length > 0) { for(i = 0; i < data.response.length; i++) { scoresTable = '<tr><td><a href="http://vkontakte.ru/id' + data.response[i].user_id + '" target="_blank">' + data.response[i].user_name + '</a></td><td>' + data.response[i].score + '</td></tr>' + scoresTable; } scoresTable = '<table border="0" class="scores-table" align="center"><thead><tr><td></td><td></td></tr></thead><tbody>' + scoresTable + '</tbody></table>'; } else { scoresTable = '<div style="text-align: center"> </div>'; } $('#scores-dialog:ui-dialog').dialog('destroy'); $('#scores-dialog') .html(scoresTable) .dialog({ title: ' ', modal: true, draggable: false, resizable: false, buttons: { '': function(){ $(this).dialog('close'); } } }); } else { showError(data.error.error_msg, false); } }); })
As can be seen from the handler code, each new row of the table is added before the previous one, which is related to the feature of the game results described above.
Result
The final file general.js can be viewed
here , the game itself is available through the link
VK Memory .
To create the application, plugins for jQuery
Pines Notify and
BlockUI were used. All information about the VKontakte API can be obtained on
the developer page .