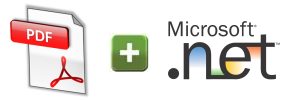
To write this article, I pushed the
HTML topic
to PDF , though due to the fact that it is dedicated to the php language, it was of little use to me personally, since My experience with php was limited to the translation of several scripts in C #, so I decided to make a small overview of what is available for working with pdf using the means of C #.
I got into the list of 7 libraries, which I will say a few words, and for the most popular (judging by the answers to stackoverflow), I will write how to make the simplest document with it. I’ll say
right away that this is
iTextSharp and work with it will be described at the end of the article.
Library Overview
1.
iTextSharpIText library allows you to create and manipulate PDF documents. It allows developers to improve web and application applications through dynamic generation and / or manipulation of PDF documents.
Developers can use
iText for:
- Transfer PDF to browser
- Generate dynamic documents from XML files or database
- Use a lot of interactive features PDF
- Add bookmarks, number of pages, watermarks, etc.
- Separate, merge and manipulate PDF pages
- Automate filling out PDF forms
- Add digital signature to PDF file
iText is available in two languages: Java and C #.
')
2.
Report.NETReport.NET is a powerful library that helps you generate PDF documents in a simple and flexible way. A document can be created using data that was obtained from any ADO.NET data set.
Library features:
- Fully written in C # for the Microsoft .NET framework
- Very compact code (Hello World: 6 lines)
- Supports graphic objects: text, lines, rectangles, jpeg images
- Easy alignment and transformation of graphic objects
- ASP.NET can generate dynamic PDF pages
- XML Documentation (Comment Web Pages)
3.
PDFsharpPDFsharp is a C # library that very easily creates PDF documents on the fly. It looks like GDI + drawing procedures, like you can create PDF documents, draw on the screen or send to print any printer. PDFsharp can modify, merge and split existing PDF files or move pages from existing PDF files to new PDF documents.
PDFsharp is an open source library that easily creates PDF documents from any .NET language.
4.
SharpPDFSharpPDF is a C # library that can create various objects in PDF documents in a few steps. It was created for .NET framework 1.1 and can create 100% compatible PDF files (tested with Acrobat Reader, Ghostscript, JAWS PDF Editor and other PDF readers). The most important goal of a library is simple use.
Library features:
- You can use with Windows Forms to generate new pdf files or save them to the database.
- You can use with Web Applications (ASP.NET) to generate pdf files or send the result directly to the browser.
5.
PDFjet Open Source EditionPDFjet is an open source library for dynamically creating PDF documents from Java and .NET.
Library features:
Drawings: points, lines, boxes, circles, Bezier curves, polygons, stars, a complex of paths and shapes.
Text: Unicode support, kerning text using Helvetica and Times-Roman base font families, inserting hyperlinks, just using a table class with the flexibility of reporting features.
Supports the insertion of the following types of images: PNG, JPEG, BMP
6.
ASP.NET FO PDFFO PDF is similar to ASP.NET Server Controls, written in C #. It receives a DataTable and several other parameters for generating XSL FO and renders the DataGrid as a PDF report using NFOP (Apache FOP Port in J #) PDF Formatter.
7.
PDF ClownPDF Clown is a C # 2.0 library for reading, processing, and writing PDF files with multiple layers of abstraction to suit different programming styles: from the lower level (PDF Object Model) to the highest (PDF document structure and streaming content). Its main target platform is GNU / Linux, but thanks to the Mono project, it is practically platform-independent.
Quick start with iTextSharp
As I wrote earlier, this library was chosen not because I tested all of the above and chose it, but because it is most often referred to and advised. So, go to the project site, we see that you can buy a book, as well as download files:
I don’t have the desire to collect something myself, so I’m not downloading the source code, but the
itextsharp-5.0.5-dll.zip library right
away . With one library, a quick start will not work, therefore, download examples
iTextSharp.tutorial.01.zip . Opening the archive of examples, I realized that the start would be much faster than I thought, because there are as many as 13 chapters of examples. I immediately thought that this could be finished, but I decided to make a small application.
I will outline in steps what is needed to use the library:
- I create WinForms application.
- Add a link to
itextsharp.dll .
- I install the button on the form and add the following code to the click handler:
var doc = new Document();
PdfWriter.GetInstance(doc, new FileStream (Application.StartupPath + @"\Document.pdf" , FileMode .Create));
doc.Open();
iTextSharp.text.Image jpg = iTextSharp.text.Image.GetInstance(Application.StartupPath + @"/images.jpg" );
jpg.Alignment = Element.ALIGN_CENTER;
doc.Add(jpg);
PdfPTable table = new PdfPTable(3);
PdfPCell cell = new PdfPCell( new Phrase( "Simple table" ,
new iTextSharp.text. Font (iTextSharp.text. Font .FontFamily.TIMES_ROMAN, 16,
iTextSharp.text. Font .NORMAL, new BaseColor(Color.Orange))));
cell.BackgroundColor = new BaseColor(Color.Wheat);
cell.Padding = 5;
cell.Colspan = 3;
cell.HorizontalAlignment = Element.ALIGN_CENTER;
table.AddCell(cell);
table.AddCell( "Col 1 Row 1" );
table.AddCell( "Col 2 Row 1" );
table.AddCell( "Col 3 Row 1" );
table.AddCell( "Col 1 Row 2" );
table.AddCell( "Col 2 Row 2" );
table.AddCell( "Col 3 Row 2" );
jpg = iTextSharp.text.Image.GetInstance(Application.StartupPath + @"/left.jpg" );
cell = new PdfPCell(jpg);
cell.Padding = 5;
cell.HorizontalAlignment = PdfPCell.ALIGN_LEFT;
table.AddCell(cell);
cell = new PdfPCell( new Phrase( "Col 2 Row 3" ));
cell.VerticalAlignment = PdfPCell.ALIGN_MIDDLE;
cell.HorizontalAlignment = PdfPCell.ALIGN_CENTER;
table.AddCell(cell);
jpg = iTextSharp.text.Image.GetInstance(Application.StartupPath + @"/right.jpg" );
cell = new PdfPCell(jpg);
cell.Padding = 5;
cell.HorizontalAlignment = PdfPCell.ALIGN_RIGHT;
table.AddCell(cell);
doc.Add(table);
doc.Close();
* This source code was highlighted with Source Code Highlighter .
The result of this code:
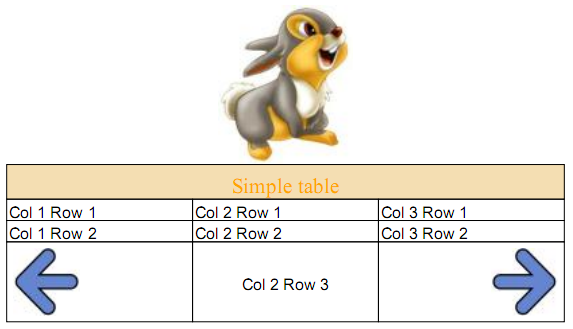
findings
For each of the libraries listed on the Internet, you can find a lot of information (I recommend starting with the developer sites), preparing this library selection, I met different opinions, although all the libraries seemed to be designed to work with pdf, but the possibilities are different, everything depends from your needs. Honestly, I didn’t use all of them, I just tried a few of them and settled on iTextSharp.
Wish: If you indicate that you have stopped at a particular library, then do not be lazy to indicate what was decisive for you in choosing.