Anyone who studies Ruby knows that all entities in it are objects, but sometimes they don’t know how much. So, classes in Ruby are also objects.
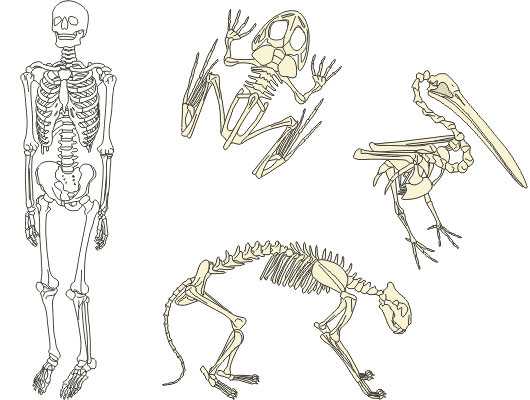
')
In Ruby, classes are objects that are instances of Class.
Reminder from OOP
A class is a type that describes the structure of objects.
Object - an entity in the address space of a computer that appears when creating an instance of a class.
In the future, for readability, I will sometimes use the term “instance” for an
objectand the term
type for
class .
Class properties need to be stored somewhere.
Since each described class has different properties and executable code, they need to be stored somewhere. Where does Ruby store data? That's right, in objects. And it is logical that objects describing a class should have their own type. So it is, the type (class) of objects describing classes is
Class .
Anonymous class
The mechanism for creating a class in Ruby can be understood in such an unpopular way.
myklass = Class.new do def test puts "TEST" end def self.ctest puts "CLASS TEST" end end myklass # => #<Class:0xb6f3e768> myklass.class # => Class myklass.name # => "" myklass.ctest # => "CLASS TEST"
We have now created an absolutely normal, but anonymous class (the name method returns an empty string) and
put it in the variable
myklass . What is
myklass ? This is an object that stores the description of our class, the class of this object is
Class .
Our anonymous class, all its methods and variables, exists only in the
myklass object.
Instance of an anonymous class
An instance of an anonymous class (that is, its object) is created as well as for any other:
m = myklass.new m.test # => 'TEST' m.class # => #<Class:0xb6f3e768> m.class==myklass # => true
Anonymous class inheritance
Inheriting an anonymous class is the same as a non-anonymous class.
class MyKlass2 < myklass # ... end MyKlass2.superclass == myklass # => true # : mk2 = Class.new myklass do # .. end mk2.superclass == myklass mk2.name # => ""
Call me by name
There's a bit of Ruby magic here. The class name appears at the moment when the object describing the class is assigned to the Constant.
As you know, in Ruby, constants begin with a capital letter, hence the names of classes.
MyKlass = myklass MyKlass # => 'MyKlass' MyKlass.name # => 'MyKlass' myklass.name # => 'MyKlass'
Please note that
MyKlass is not a class name, it is a constant that stores an object describing a class. But at the moment when we assigned it to this constant, our class had a name that coincides with the name of a constant.
In general, the class name more often is merely informative. After all, you use a class not by name, but with the help of the same variable or Constant in which it is stored.
Chicken or egg?
The
Class type, as well as the other classes, is inherited from the
Object type. But, after this:
Object.class # => Class Class.class # => Class Class.superclass # => Module Module.class # => Class Module.superclass # => Object
figure out what is primary -
Object or
Class is impossible. How can a
Class be a constant storing an object of a class of which it is an instance? In fact,
Object ,
Class and
Module are the primary structures inherent in the interpreter, and they are not formed from each other by ruby-code, although Ruby successfully pretends that this is so.
Interesting fact.
In fact, in Ruby there are no class methods (which, we think, we describe it by
def self.aaa ). There are only methods of objects, including objects of type Class.
But more about that next Thursday ...
Related Links
rhg.rubyforge.org/chapter02.htmlrbdev.ru/2010/07/izuchaem-yadro-ruby-klassy-i-obektystackoverflow.com/questions/1219691/why-object-class-class-in-ruby