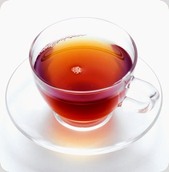
What programming language do you prefer? C ++? Or pure C? Or are you a fan of scripting languages - Python or PHP?
Of course, you will answer that it depends on the tasks that you face.
Good. Let's try to implement a simple algorithm - to make a cup of tea - in different programming languages.
You can see what happened with me. Implementations do not claim the right to be perfect, and the results are different. But it's not tea - it's about the language! So...
Immediately make a reservation - the programs are not complete. And why are they needed? After all,
The Hello World Collection has long existed in more than 400 languages and
99 Bottles of Beer , with almost 1,000 more implementations. But the disadvantages of these collections are that they very weakly reflect the style of programming as such, and boil down to a maximum of one cycle with print'ami. We will try to look a little deeper.
Let's start, according to tradition (or perhaps without tradition), from C. Language. Laconically, consistently and without any frills, we got this piece of code:
<br> #include <stdbool.h> <br><br> #define TEA_OK 0 <br><br> typedef enum _tea_type { <br> TEA_BLACK , <br> TEA_GREEN<br> } tea_type ; <br><br> typedef struct _tea_cup { <br> tea_type type , <br> bool sugar<br> } tea_cup ; <br><br> int drink_tea ( tea_cup * cup ) { <br> return TEA_OK ; <br> } <br>
Sorry, I didn’t go into details, highlight the headings.h and describe the use of all the "tea" parameters ... But there is something to compare with.
Next is C ++. A huge creative space for those who like to "decorate" the dry code C with all sorts of gadgets and abstractions. But most importantly, what should not be forgotten -
when I
drank some tea - take it with you! (it was a hint of destructors and discussions around them)
<br> #include <string> <br><br> class TeaCup { <br> public : <br> enum Type { <br> BLACK , <br> GREEN<br> } ; <br> TeaCup ( Type type ); <br> TeaCup ( std :: string sType ); <br> ~ TeaCup (); <br> protected : <br> Type type ; <br> } ; <br><br>TeaCup ::~ TeaCup () { <br> DishWasher :: wash ( this ); <br> } <br>
It would seem that the ideal object implementation? Is everything in its place? Not here it was! Let's look in the direction of Java, where the engineering idea of designing the internal space of the application and organizing the code is not dormant and should not be asleep! The goal is clear - use our code in other interfaces.
<br>public interface Drinkable { <br> int drink (); <br> } <br><br>public class Boiled { <br> protected bool boiled ; <br> void setBoiled ( bool boiled ); <br> bool getBoiled (); <br> } <br><br>public class TeaCup extends Boiled implements Drinkable { <br> int drink () { <br> return 0 ; <br> } <br> } <br>
And we have not come up with TeaCupFactory yet and have not planted a person for a cup of tea to implement it as intended! But let's not get going ... Next in line is Python. The meaning is simple: by redefining the magical methods of objects we achieve a better interface and usability. Simplicity and conciseness, rampage underscores.
<br> class TeaCup : <br><br> def __init__ ( self ): <br> self . want = [] <br><br> def __setattr__ ( self , name , value ): <br> if value : <br> self . want . append ( name ) <br><br> def __str__ ( self ): <br> return "Tea: %s" % ( ", " . join ( self . want )) <br><br> def drink ( self ): <br> return str ( self ) <br><br> if __name__ == "__main__" : <br> cup = TeaCup () <br> cup . sugar = True<br> cup . lemon = True<br> cup . drink () <br>
Not the most convenient interface turned out? Nothing wrong. Another time it will be more accurate. Or maybe try Perl? Compact code, the syntactic richness of the language, ah ...
<br>use Carp qw ( croak ); <br><br> sub drink_tea { <br> croak "Shit!" if wantarray ; <br> my $type = shift || "black" ; <br> my ( $sugar , $lemon ) = @_ ; <br> croak "What?" unless $type =~ /^ black | green$ / i ; <br> 1 ; <br> } <br>
I hope you understand what I mean? Where without without! Yes, and performance probably does not lose. That's just sometimes difficult to understand ... My example is that I’m not a true Perl programmer. And imagine that you can skillfully know something? Not that miserable PHP programmers who interfere in one heap of procedural programming and OOP!
<br> set_include_path ( dirname ( __FILE__ ) . PATH_SEPARATOR . "lib" ); <br> require_once ( "tea.cup.php" ); <br>$cup = new Tea_Cup (); <br>$cup -> with_lemon = true ; <br>$cup -> fill (); <br> header ( "Content-Type: tea/black" ); <br> set_time_limit ( 0 ); <br>$cup -> drink (); <br>
Of course I forgot the triangular brackets with question marks ... Well, okay. But in JavaScript it's not at all like that. Nothing extra. Do not forget only to make global variables local (well, sort of, to optimize access to them) and arrange semicolons so that our code can be integrated into large files.
<br> ;( function ( w ) { <br> var d = w . document , g = d . getElementById ; <br> var cup = g ( "tea-cup" ); <br> if (! cup ) return ; <br> if ( cup . className . indexOf ( "black" ) >= 0 ) <br> cup . appendChild ( d . createElement ( "sugar" )) ; <br> cup . style . visibility = "hidden" ; <br> } )( window ); <br>
Here we are gradually getting to the end ... At least the code is over. Therefore, I hasten to answer your questions, which, probably, are already tormenting.
')
-
Why all this? - Let's look at programming stylistics in different languages! On the approaches to the organization of the program code, on the frequently used "chips" of languages, regardless of the tasks. Of course, a little (or even strongly) exaggerated, but still. Isn't that what most PHP coders write in this style? And aren't Apache or Nginx source files organized in a similar way?
-
Which language is better? “But I don’t know this ... I love some more, some less.” But this is subjective. The main thing is not what you write, but
how you do it. Then, after all, work can be a joy, and everything will turn out!
UPD: I apologize for the somewhat "strange" C ++, Java and Python. Something made me commit in these languages stupid mistakes when publishing. Thanks again to the commentators!