This is a very superficial guide that will only introduce you to the basic functionality of the Play framework using the example of creating the 'Hello World' application.
(Part 1)Form creation
Let's start Our application 'Hello World' with creating a form where you can enter a name.
Edit helloworld / app / views / Application / index.html as follows:
')
#{extends 'main.html' /} #{set title:'Home' /} <form action="@{Application.sayHello()}" method="GET"> <input type="text" name="myName" /> <input type="submit" value="Say hello!" /> </form>
We use the @ {...} notation for Play to automatically generate a URL on Application.sayHello. Now, refresh your browser homepage.
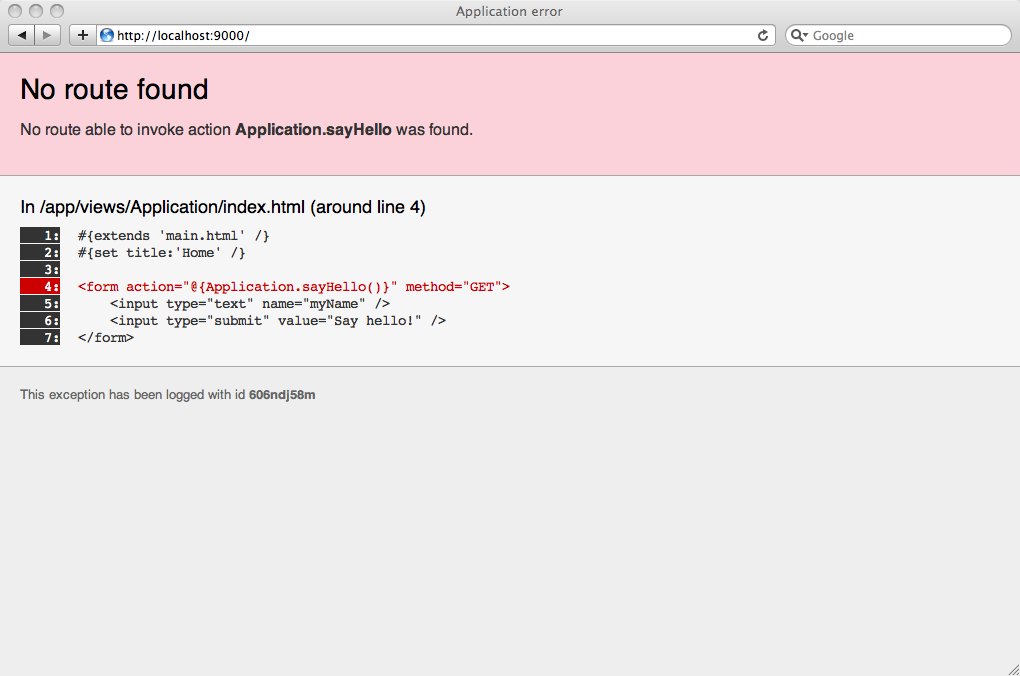
Sorry, you get an error. This is because you are referring to the non-existent Application.sayHello action. Let's create it in helloworld / app / controllers / Application.java:
package controllers; import play.mvc.*; public class Application extends Controller { public static void index() { render(); } public static void sayHello(String myName) { render(myName); } }
We declared the myName parameter in the sayHello method, so it will be automatically populated with the value of the myName parameter that will be received via HTTP. And we call the render () function to display the template that was passed to myName, so it will be available in the design template.
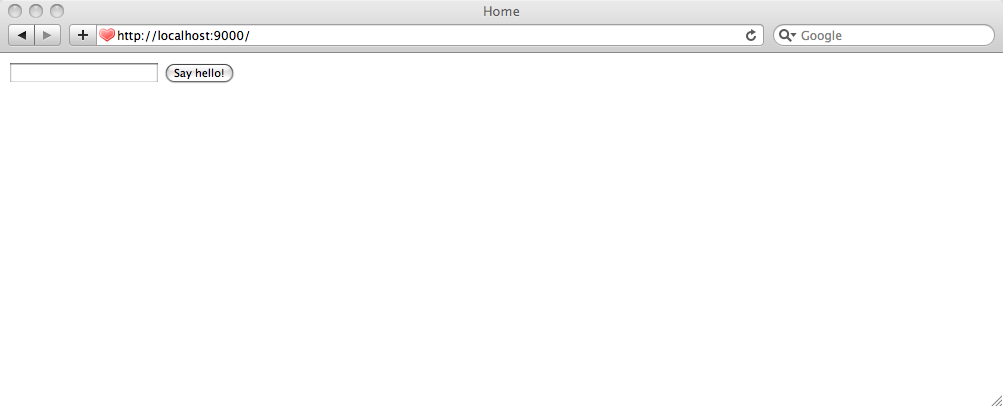
Now, if you try to enter your name and submit the form, you will receive another error:
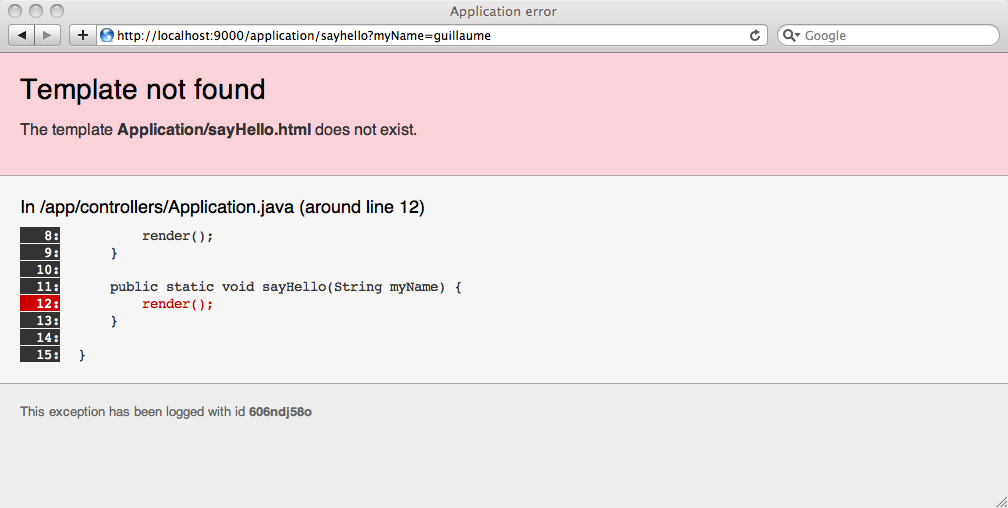
Understanding the cause of this error is quite simple. Play attempts to invoke the default template for this action, but it does not exist. Let's create it helloworld / app / views / Application / sayHello.html:
#{extends 'main.html' /} #{set title:'Home' /} <h1>Hello ${myName ?: 'guest'}!</h1> <a href="@{Application.index()}">Back to form</a>
Now refresh the page.
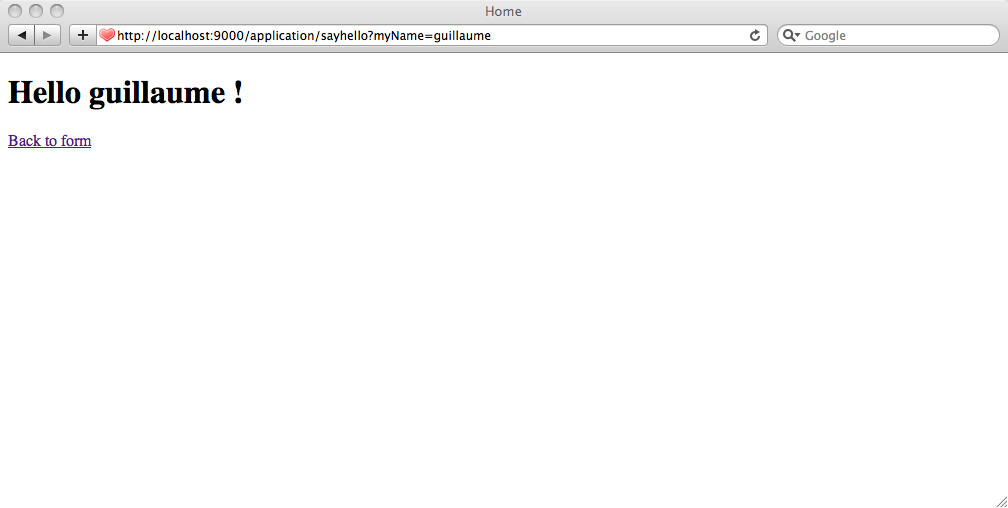
See how we used the Groovy?: Operator. It switches to the default value if the myName variable is not populated. So if you are trying to submit a form without entering a name, it will display 'Hello guest'.
CNC setting
If you look at the URL of the created page, you will see something like:
http: // localhost: 9000 / application / sayhello? myName = guillaumeThis is because Play uses the default route for all controller actions:
* /{controller}/{action} {controller}.{action}
We can set the best URL by specifying the path for the Application.sayHello user actions. Modify helloworld / conf / routes by adding the following after the first line:
GET /hello Application.sayHello
Now back to the form and re-enter your name to make sure that everything works.
Customize output template
Since templates inherit the main.html template, you can easily modify all templates. Modify helloworld / app / views / main.html:
... <body> The Hello world app. <hr/> #{doLayout /} </body> ...
The string "The Hello world app." Appeared on all pages.
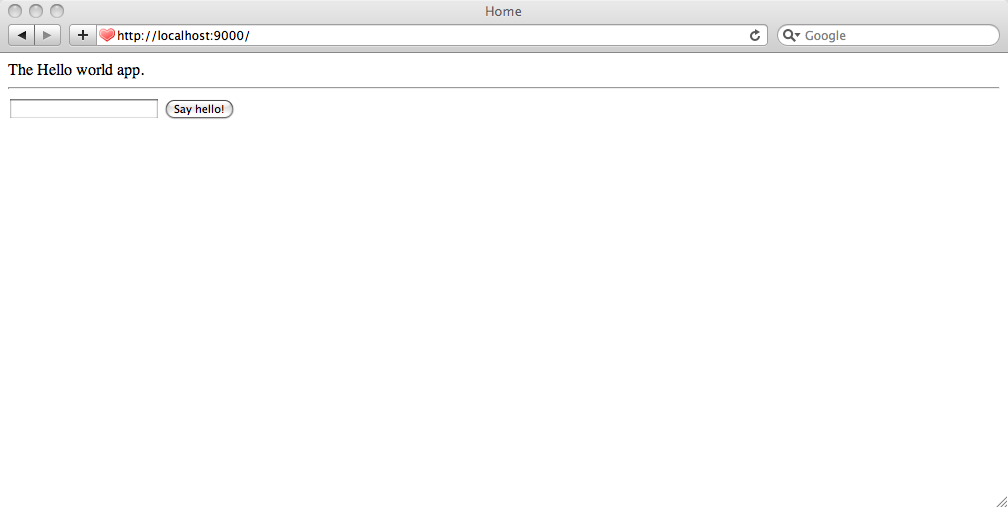
Input Validation
Add a check to the form. The form must be completed before shipping. For this we can use the Play validation framework.
Modify sayHello in the helloworld / app / controllers / Application.java controller as follows:
... public static void sayHello(@Required String myName) { if(validation.hasErrors()) { flash.error("Oops, please enter your name!"); index(); } render(myName); } ...
Remember to import play.data.validation. * To use the @Required annotation. Play will automatically check that the myName field is filled in or will add an object containing an error. Then, if there is any error, we will add a message and redirect it to the root of the site.
Flash object allows you to save messages when redirecting to the page.
Now you just have to show the error message, if any. Edit helloworld / app / views / Application / index.html:
#{extends 'main.html' /} #{set title:'Home' /} #{if flash.error} <p style="color:#c00"> ${flash.error} </p> #{/if} <form action="@{Application.sayHello()}" method="GET"> <input type="text" name="myName" /> <input type="submit" value="Say hello!" /> </form>
Check how it works:
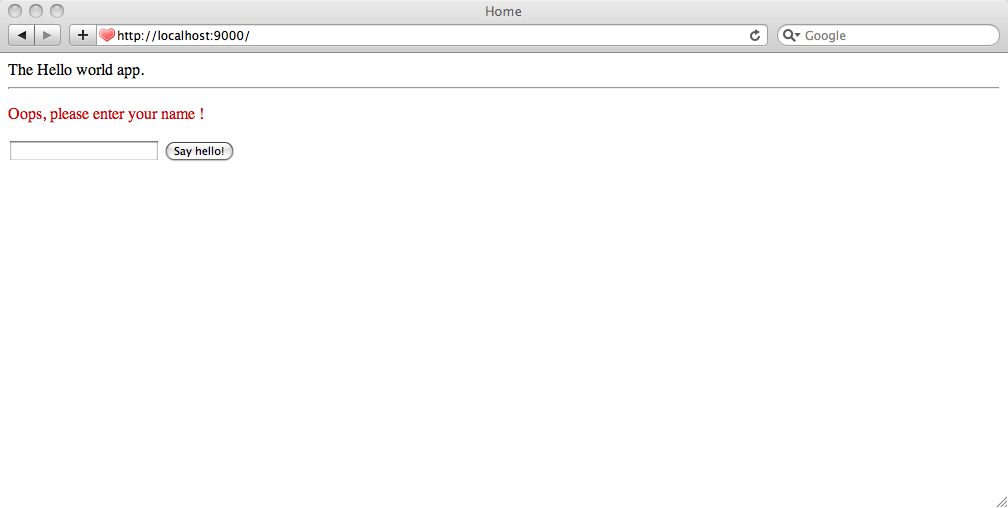
Automated Testing
Let's finish the guide by writing a test for the application. Since there is no logic in the Java code to check, we need to check the web application. Therefore, we will write a test for Selenium.
To start, run the application in test mode. Stop the application and restart it with the command:
$ play test
The play test command is almost the same as the play run, except that the Test Runner module is additionally loaded, which allows you to run a test suite directly from the browser.
Open the following address in the browser
http: // localhost: 9000 / @ tests to see the tests. Try to select all the default tests and run them, all should be green ... But these are standard tests, which generally do not test anything.
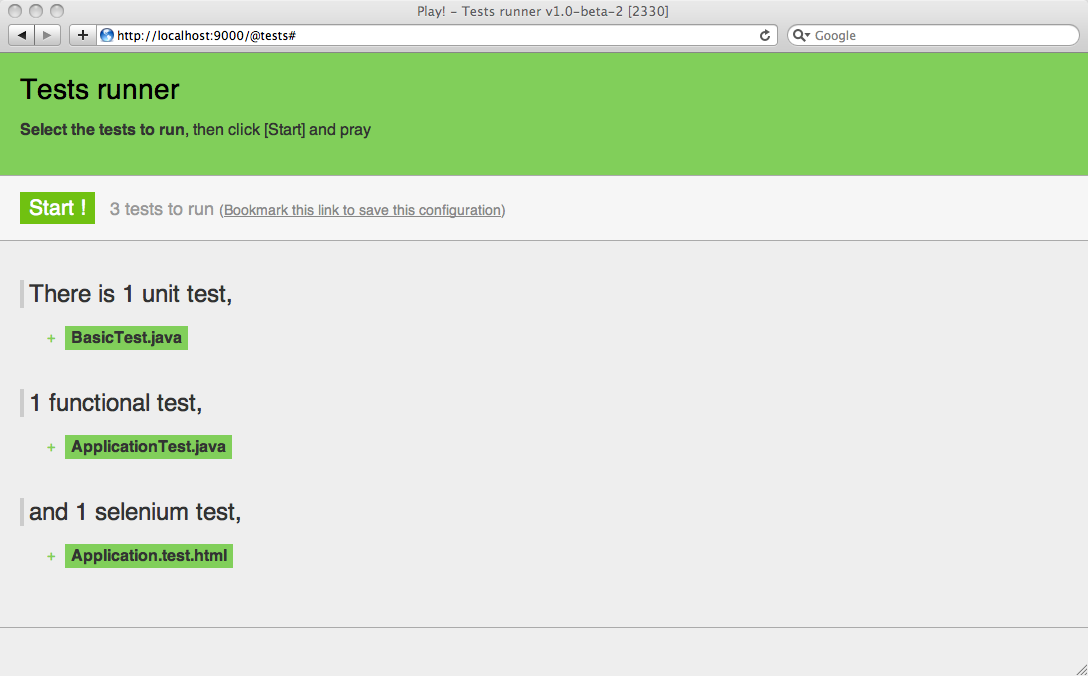
Tests for Selenium are usually written as an HTML file. Writing tests for Selenium is a bit tedious (formatting using HTML table elements). The good news is that Play will help you generate it using the Play template engine, which supports a simplified syntax for Selenium scripts.
When creating a Play application, it automatically created tests, including tests for Selenium. Open helloworld / test / Application.test.html:
*{ You can use plain selenium command using the selenium tag }* #{selenium} // Open the home page, and check that no error occurred open('/') assertNotTitle('Application error') #{/selenium}
This test should work without any problems. It simply opens the main page and makes sure that the pages do not contain the text 'Application error'.
We write a test for our application. Change the test content:
#{selenium} // , open('/') assertNotTitle('Application error') // assertTextPresent('The Hello world app.') // clickAndWait('css=input[type=submit]') // assertTextPresent('Oops, please enter your name!') // type('css=input[type=text]', 'bob') clickAndWait('css=input[type=submit]') // assertTextPresent('Hello bob!') assertTextPresent('The Hello world app.') // clickAndWait('link=Back to form') // ? assertTextNotPresent('Hello bob!') #{/selenium}
We have fully tested the application. Simply select this test in Test Runner and click Start. Everything should be green!
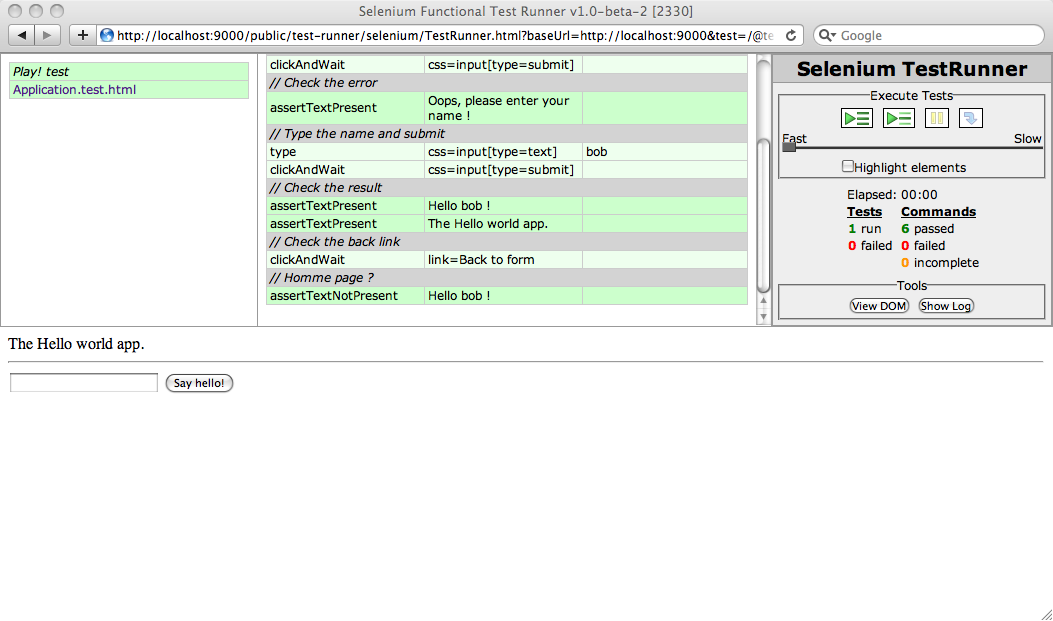
Want more?
It was a very simple lesson. If you want to learn more about the Play framework, read
"Tutorial - Play guide, a real world app step by step .
"