In this article I will tell you how to write a simple application for
BlackBerry smartphones, which are manufactured by the Canadian company RIM (Research In Motion).
Development tools are provided by RIM for free. Debugging of the application can be done both on the simulator, and directly on the device. In order to be able to run the application on the device, the compiled file with the .cod extension must be signed with a special electronic key that
is provided by RIM and is perpetual. It is theoretically possible to do without an electronic key. The key is not necessary if you run the written program only in the simulator. There is also no need for a key if you, when writing an application intended for execution on a device, use only classes that are not included in the “controlled API” list. I should note that most of the RIM API classes are included in this list and applications that use them must be signed, otherwise they will not start on the device.
The process of obtaining the key is very simple. Fill out the
form on the RIM website , specify the details of your credit card and make a purchase (now the keys are provided free of charge). In a couple of days, e-mail files with instructions for their use come to your e-mail. After some time a paper letter with documents confirming the purchase of keys will be sent to the usual mail.
')
Developing applications for BlackBerry smartphones can be conducted using the following tools:
- BlackBerry Java Development Environment (BlackBerry JDE) - was one of the first tools to develop BlackBerry applications in Java. Now released more out of habit than out of necessity. To develop a somewhat complex application in it is quite difficult. There are not many convenient features with which modern IDEs are equipped, such as Eclipse, Netbeans, IDEA and others.
- BlackBerry JDE Plugin for Eclipse - plugin for Eclipse, by installing which, you can create and debug applications for BlackBerry. Much more convenient tool than the BlackBerry JDE. Before installing it, you need to install the version of Eclipse required by the plugin. The path to the installed Eclipse will be requested during the installation of the BlackBerry JDE Plugin.
- A set of tools for creating the so-called "Browser Applications", which are written using HTML, CSS and JavaScript and run in the browser BlackBerry.
I will create the application described in this article using Eclipse and the BlackBerry JDE Plugin for Eclipse. It should be noted that development tools that use Java do not support innovations that have appeared in Java since version 1.5. That is, unfortunately, it is not necessary to think about the use of generics, enums and other new features.
So, install the version of Eclipse supported by the BlackBerry JDE Plugin, and then install the plugin itself.
Then we run Eclipse and create our first project.

Specify the name of the project. The value of “BlackBerry JRE 5.0” in the “JRE” section of the dialog box indicates which version of the RIM API is used in the project. In this case, RIM API version 5.0.0 is used.

You can install support for other API versions and updates for BlackBerry JDE Plugin for Eclipse through the
standard Eclipse update mechanism .
A detailed description of the subtleties of working with the BlackBerry JDE Plugin for Eclipse is given in its documentation, which can be
downloaded from the site .
After closing the project creation dialog, an application descriptor window will open in front of us. It describes all the necessary properties of the application.

Here we will stop and consider the descriptor fields in more detail. We need only some of them.
- Title - the name of the application that will be displayed on the device screen.
- Version - version of the application
- Vendor - the author of the application
- Description - a brief description of the application. During installation, the user will be able to read it by clicking the corresponding button in the BlackBerry Desktop Manager , if the installation is done via a USB cable, or he will see the description when installing the application via the Internet by opening the corresponding jad file on the server.
- Application type - the type of application. We leave the default "BlackBerry Application", which means - "BlackBerry application." In addition to this type, there is also the “Library” type, to compile a library that can be used by other programs installed on the device. The “MIDlet” type implies creating a MIDlet using Java2ME classes.
- Application argument is the argument that will be passed to main (String [] args). In our case, we need this field unnecessarily.
- Auto-run on startup - turn on if the application should start when the device starts. Startup tier tells what queue your application will be running. In the BlackBerry Java Development Environment, it was possible to specify a small value for this field, which caused the application to start before the components of the operating system needed for its operation were run. In Eclipse, only two possible values ​​were left: 6 and 7. Seven means to run as the last thing at the start of the operating system.
- Do not display the home screen - turns on when we create a non-UI application that runs in the background and usually starts when the device is turned on.
- Locale resources section - used to specify localization resources that will be used in this application. In this article we will not dwell on this in detail.
- Application icons - allows you to set one or more icons for the application. We also will not dwell on this issue in this article.
We create the first and main class of our application.

Applications for BlackBerry are both visible (the so-called Ui-applications) and background. As we write the Ui-application, we inherit the main class of our application from
net.rim.device.api.ui.UiApplication .
Initially, the main class of our application looks like this:
package test.myfirstapp; import net.rim.device.api.ui.UiApplication; public class MyApplication extends UiApplication { public static void main(String[] args) { } }
Now create a class to display information on the device screen.

We inherit it from the class
net.rim.device.api.ui.container.MainScreenAfter creating the class, we write code for it:
package test.myfirstapp.view; import net.rim.device.api.ui.component.LabelField; import net.rim.device.api.ui.container.MainScreen; public class MyAppScreen extends MainScreen { public MyAppScreen() {
Then we return to the main class of the application and write the code for it:
package test.myfirstapp; import test.myfirstapp.view.MyAppScreen; import net.rim.device.api.ui.UiApplication; public class MyApplication extends UiApplication { public static void main(String[] args) {
That's basically it. You can run the application in the simulator.
The BlackBerry JDE distribution plugin for Eclipse 1.1 includes a set of simulators for BlackBerry devices. The set of simulators in Eclipse can be expanded by installing support for other versions of the API. The current used simulator can be set up in the dialog box by calling the menu: Run - Run Configurations, tab “Simulator”
The default is the simulator for the
BlackBerry Storm 9550 .
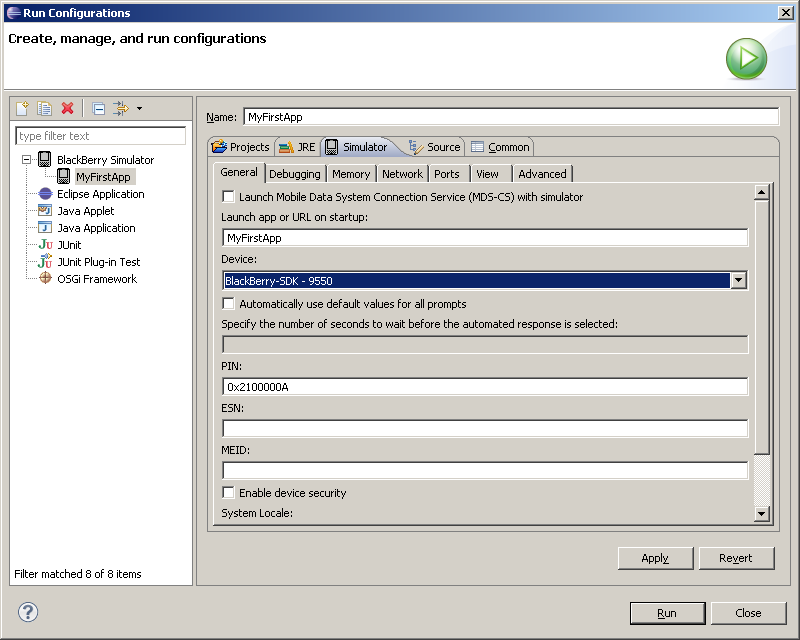
Run the program in the simulator by calling from the menu: Run - Run As - BlackBerry simulator

A simulator window will appear in which you can select the application we have written and run it. The process of selecting and launching an application is shown in the animated image below.
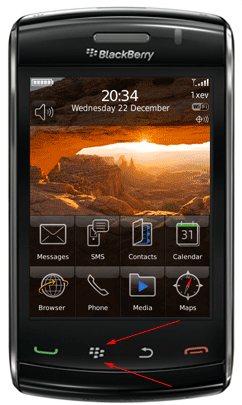
Those who are interested can read
about the RIM API version 5.0 classes and get acquainted with a large number of
various documentation and developer
guides .
There is an
official forum dedicated to the development of applications for BlackBerry , where you can chat with other programmers and with the employees of Research In Motion.