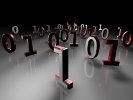
This article is the result of my first experience with static analysis of a fairly large program (1,665 source files at the moment). In addition, this is my first experience using the Microsoft Visual Studio environment. The development of the analyzed program was carried out exclusively in Ubuntu, Eclipse CDT, the GCC compiler.
Project preparationIf you are fluent in “studio” and have long learned to develop Qt-projects in it, feel free to skip this section, since here is my way of preparing a project for VS2010.
')
Since I am developing a program based on a Qt framework, there is a .pro file in my project. This file is the basis for generating a project. The Qt website (
http://doc.qt.nokia.com/stable/qmake-project-files.html ) indicates that qmake allows you to generate “studio” projects. To do this, use the command:
$ {QTDIR} \ bin \ qmake.exe -t vcapp -spec $ {QTDIR} \ mkspecs \ win32-msvc2010
After that, the project file * .vcxproj will appear, which opens with the “studio”. No more manipulations were required - the project is successfully compiled.
Installing and configuring PVS-StudioInstallation is as simple as it’s possible: downloaded, installed, restarted, and now there is a tab in the menu - PVS-Studio. I had to tinker a bit with the setup. For reasons unknown to me, when analyzing a project (PVS-Studio-> Check solution), PVS cannot find Qt header files (for example, QWidget.h or QObject.h), although, I repeat, the project is compiled without errors. To fix this situation, you need to go to the project settings (right click on the project -> Properties), select “VC ++ Directories” and in the “Include directories” section specify the paths to the Qt header files. Moreover, you need to specify not just the root folder Qt \ include, but include, by module, the subfolders that you use. That is, if the .pro file indicates that your project uses the core, gui, xml, sql, network, webkit, opengl modules, it means that you need to add include, include \ qtcore, inclide \ qtgui, include \ qtxml, include \ qtxmlpatterns, include \ qtsql, include \ qtnetwork, include \ qtwebkit, include \ qtopengl. After implementing these actions, PVS-Studio starts working correctly.
Static analysisThe analysis took about 4 hours, at that time I was snowboarding. So, it was discovered:
- 120 Category 1 Warnings
- 164 Category 2 Warning
- 287 Category 3 Warnings
Category 3In this category,
~ 250 (
87% percent of the total) warnings
“V001. A code fragment from 'file' cannot be analyzed ” , reporting that PVS did not cope with the analysis of a specific file. Many warnings refer to auto-generated files like moc * .cpp. It would be more convenient for me if PVS did not try to analyze these files.
The next most popular warning is the
“V550. An odd precise comparison. “Epsilon or fabs (A - B)> Epsilon” . Analysis of warnings showed
8 false positives and
1 true.
The following warning in this block:
“V126. Be advised that the size of the type of "long" varies between LLP64 / LP64 data models ” :
This is a diagnostic message.
Of course, the presence of the "long" type is in. But it may be necessary to review the program code.
Windows and Linux use different data models for the 64-bit architecture. This is an example of base data types such as int, float, pointer, etc. Windows uses the LLP64 data model while Linux uses the LP64 data model. In these models, the sizes of the 'long' type are different.
In Windows (LLP64), the size of the 'long' type is 4 bytes.
In Linux (LP64), the size of the 'long' type is 8 bytes.
For all types of formats, it is possible to make files on the linux or windows. So that you can use it to review all the code fragments where the 'long' type is used.
Let me remind you that I use Qt framework and therefore use the long type in general is a bad tone, you need to use qint16, qint32 and qint64 for cross-platform development. The only question is what the use of PVS in this case will be better than searching the entire project. After all, these warnings are related to Viva64 - a paid product. For these warnings, code navigation is disabled (it becomes very difficult to understand and edit).
The warning that I could not analyze because of the program’s triviality:
“V111. Call function 'foo' with variable number of arguments. N argument has memsize type ”Category 2There is a depressing situation in this category: warnings related to General Analysis (free analysis) -
4 pieces (
2% of the total) and
160 trial warnings from Viva64.
Paradoxically, the lion's share of the trial warnings pertain to
“V112. Dangerous magic number N used ” , which are also found in category 3. According to what principle, PVS put a part into category 2, and a part into category 3 is a mystery to me. I think the analyzer of this warning should be improved, because a quick inspection revealed
~ 90% of false positives. I would even say that there was not a single true trigger. But I didn’t check the entire sample, since the trialnost did its job - I was tired. Under this warning, magic numbers were mostly found in drawing procedures, such as: setting various indents and offsets.
Free access alerts:
“V537. Consider reviewing the correctness of 'X' item's usage ” :
The analyzer detected a potential misprint in code. Tries to identify the following type using the heuristic method:
int x = static_cast <int> (GetX ()) * n;
int y = static_cast <int> (GetX ()) * n;
In the second line, the GetX () function is used instead of GetY (). This is the correct code:
int x = static_cast <int> (GetX ()) * n;
int y = static_cast <int> (GetY ()) * n;
- false alarm. Here is the code:
int width = img_in->width();
int height = img_in->height();
if (width > height) {
if (width > maxWidth) {
height = ( int ) (( float ) maxWidth / width * height);
width = maxWidth;
}
} else {
if (height > maxHeight) {
/**/ width = ( int ) (( float ) maxHeight / height * width);
height = maxHeight;
}
}
* This source code was highlighted with Source Code Highlighter .
“V525. The code containing the collection of similar blocks. Check items X, Y, Z, ... in lines N1, N2, N3, ” - false positives.
Warnings for
paid access:
“V401. The fields of size can be ordered across the fields' order. The size can be reduced from N to K bytes, ” :
The analyzer detected a data structure memory.
Let's announce ineffective:
struct LiseElement {
bool m_isActive;
char * m_pNext;
int m_value;
};
This is a structure of 24 bytes in 64-bit code. But if you change the size of the field it will occupy 16 bytes. This is an optimized variant of the structure.
struct LiseElement {
char * m_pNext;
int m_value;
bool m_isActive;
};
...
At the moment I can not understand how this is possible, but if this is true, then this is great - this warning is very useful for me, thank you.
Category 1The situation is similar to category 2: only
1 free warning and
119 toll.
Free alerts:
“V524. It is odd that the "Foo_1" function is fully equivalent to the "Foo_2" function ” - false positive:
const qreal & getX () const {
throw std :: runtime_error ("Unsupported operation error");
}
const qreal & getY () const {
throw std :: runtime_error ("Unsupported operation error");
}
Paid alerts:
“V103. Implicit type conversion from memsize type to 32-bit type,“V104. Implicit type conversion to mems“V110. Returns from memsize type to 32-bit type,“V302. Member operator [] of 'foo' class has a 32-bit type argument. Use memsize-type here - these warnings were found in Qt source codes, specifically in the qtessolator.cpp file. Truth could not be verified due to triality.
For some errors, in particular
V114 ,
V121, PVS-Studio duplicates warnings. So, in the list of warnings, there are on average 4 copies of warnings of the same type referring to the same line.
ResultsThe feeling of using the tool is twofold. I assumed that the tool would reveal a greater number of errors, since I do not consider myself to be a genius programmer. In fact, there was one site when it was really worth fixing up. Either I think too badly of myself, or the tool is not fully polished.
The tool issued
6 (
2.11% ) free and
278 (
97.89% ) paid warnings (without the 3rd category). Very much a little free is obtained, you will not even try. The warnings associated with
V112 (magic numbers) give only false positives, and there are a lot of them - you get tired of checking them, especially they are paid.
Would I buy this tool? Unfortunately not. I spent a lot of time setting up and analyzing due to the large number of false positives. One is not a false positive for almost
500 warnings - too cruel. What is surprising, the useful warning was in 3 categories (as I understood in the most non-critical).
250 warnings found in auto generated files. I would like to see a pre-configuration which would exclude such files from processing (for example, Qt moc-files). Most of all I was pleased with the warning
V401 (you can reduce the size). Here I would buy it! I would buy all the warnings that would allow me to reduce the size and increase the speed of my application.
Thank.