In the last
article I described how to implement encoding and caching when processing xsl templates on the client side. Today, I will tell you what tools can be used to debug xslt transformations on the client side and the work of the <xsl: value-of select = "xxx" construction disable-output-escaping = "yes" /> in firefox.
Debugging XSLT on the client
During the processing of xsl documents in the browser, various errors can occur:
1) network errors - when xml or xsl data was not received;
2) template processing errors.
Network errors
To control network errors, you can use browser debugging tools. All firebug (firefox), dragonfly (opera) and devtools (chrome) debugging tools can show what resources were downloaded from the network by an asynchronous request directly. For example, if we process in_data.xml data using the first.xsl template, we will be able to control the loading of this data.
- section “net” in firebug
- “net” section in dragonfly
- section "resources" in devtools.
The situation is different if, for successful processing, you need to load an additional xsl file (includ). In this case, firebug (firefox) will not show that something has been loaded.
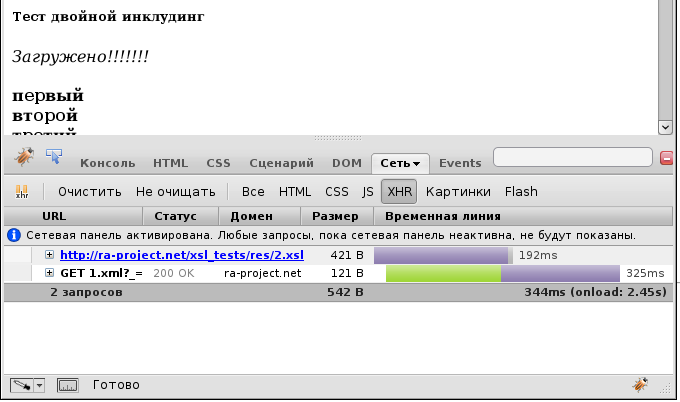
')
And the integrated debugging environments dragonfly (opera) and devtools (chrome) will show which additional xsl files have been uploaded.
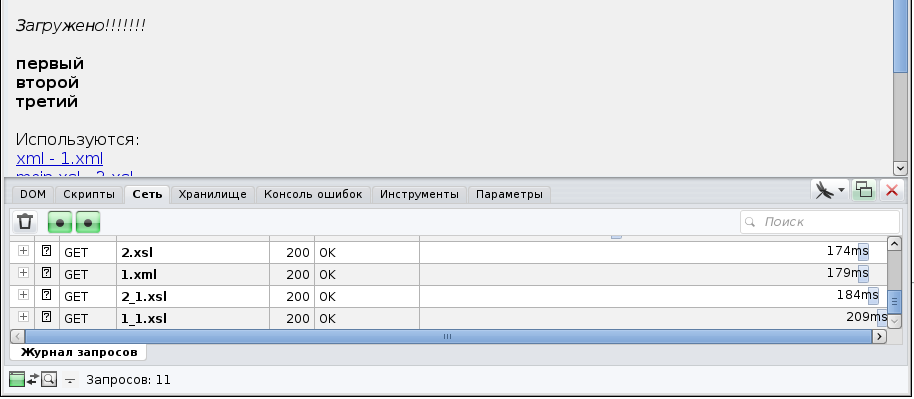
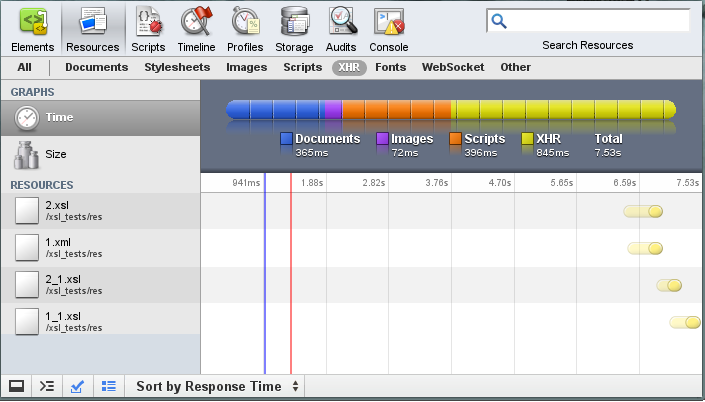
Pattern processing errors
If during the processing of the template an error occurred, then it can be caught and displayed in the form convenient for you. I find it convenient during development to drop such messages into the firebug console
jQuery( '#id_div' ).transform(
{ xml: 'res/1.xml' ,
xsl: 'res/1.xsl' ,
success: function (){
jQuery( '#id_info' ).html( '' );
},
error: function (xml_data, xsl_data, success, cur_obj, error){
console.log( 'xml: ' +xml_data);
console.log( 'xsl: ' +xsl_data);
console.log( 'success: ' +success);
console.log( 'cur_obj:' );console.log(cur_obj);
console.log( 'error:' );console.log(error);
}
});
* This source code was highlighted with Source Code Highlighter .
In this case, the error output will be something like this:
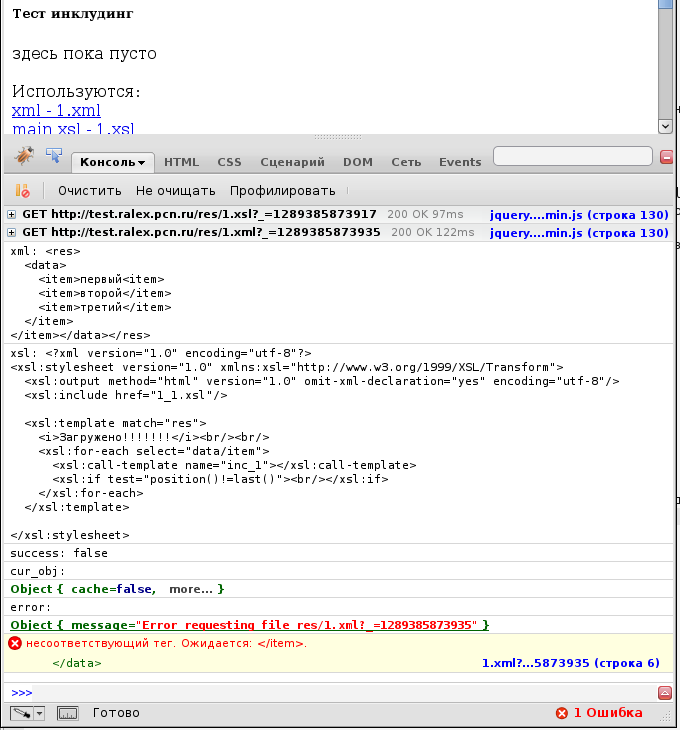
The “error” event will be triggered in the event of an error. Several parameters will be passed to the event function, the main of which is error - the error description itself.
disable-output-escaping = "yes" in firefox
Description of the problem
In some cases, in the xml-data there may come whole pieces of formatted html, in these cases these pieces need to be inserted into the final document without changes, that is, "as is". For example, it may be data containing search results in which the search word is bold (“we <b> searched </ b> all possible solutions”). You can make a simple text replacement with xslt, but you shouldn’t (try to do it at some leisure). Therefore, it is easier to transmit already formatted text. An experienced programmer will come up with a lot of examples. To insert unshielded data in xslt, there is a special attribute, disable-output-escaping, which must be set to “yes”. It looks like this
< xsl:value-of select ="xxx" disable-output-escaping ="yes" />
* This source code was highlighted with Source Code Highlighter .
But in firefox, starting with the very first version and ending with the entire third branch (in firefox 4 it was not tested), not the full implementation of the xsl processor. Their xsl processor, which identifies itself as “Transformiix”, does not handle disable-output-escaping = “yes”. This error has been for many years
https://bugzilla.mozilla.org/show_bug.cgi?id=98168 and we recently “celebrated” the 9th anniversary of this bug. The link also contains excuses to the firefox command, which can be reduced to one thing - there is a lot of work there, if you want to correct it yourself.
Solution to the problem
There is such a solution to the problem - instead of using the <xsl: value-of select = "xxx" construct, disable-output-escaping = "yes" /> call <xsl: copy-of select = "xxx" />. But in this case we cannot transfer data in the xxx node
< xxx > <! [CDATA[ < i > "" </ i > ]] ></ xxx >
* This source code was highlighted with Source Code Highlighter .
and you have to transfer data like this
< xxx > < i > "" </ i ></ xxx >
* This source code was highlighted with Source Code Highlighter .
that is, it will not be quite true xml-file. And then we get a problem in other browsers where this solution will no longer work.
Another solution
I decided to use a different approach. Use a special fix written in javascript.
1. Instead of the usual
<xsl:value-of select="xxx" disable-output-escaping="yes"/>
will have to use the call in our templates.
< xsl:call-template name ="inc_disable_output_escaping" >< xsl:with-param name ="param" select ="xxx" /></ xsl:call-template >
* This source code was highlighted with Source Code Highlighter .
Where the inc_disable_output_escaping template looks like this:
< xsl:template name ="inc_disable_output_escaping" >
< xsl:param name ="param" ></ xsl:param >
< xsl:choose >
< xsl:when test ="system-property('xsl:vendor')='Transformiix'" >
<!-- firefox -->
< div style ="display:none" class ="fix_ff_disable_output_escaping" >< xsl:value-of select ="$param" disable-output-escaping ="yes" /></ div >
</ xsl:when >
< xsl:otherwise >
< xsl:value-of select ="$param" disable-output-escaping ="yes" />
</ xsl:otherwise >
</ xsl:choose >
</ xsl:template >
* This source code was highlighted with Source Code Highlighter .
In this template by the name of the xsl processor (xsl: vendor), we will output the data with the standard construction with disable-output-escaping = “yes”, and in the case of the xsl processor from firefox, we insert the data into an invisible container like firefox can insert, that is, in shielded form.
And then, after successfully converting and updating the DOM document, we will encode the data in these containers to the correct ones and insert them in place of these containers.
function fix_ff_disable_output_escaping() {
jQuery( 'div.fix_ff_disable_output_escaping' ).each(
function (i, obj){
var j_obj=jQuery(obj);
j_obj.replaceWith(Encoder.htmlDecode(j_obj.html()));
}
)
}
* This source code was highlighted with Source Code Highlighter .
To decode an html mnemonic, the htmlDecode function of the js library Encoder is used.
An example of the operation of this technique and the
archive with the solution can be taken here
ra-project.net/xsl_tests/test2.htmlPS Do not forget to send the correct content-type for xml / xsl documents. Namely "Content-type: text / xml".