Often, the interface of even very popular Android applications is designed somehow and it seems that most developers have not even heard of the User Interface Guidelines. But following the recommendations is not very difficult, and sometimes even saves time for development. In order to show that this is indeed the case, I propose to go through several key elements of the application interface design using the example of a slightly advanced “Hello World”.
The article is designed for novice Android developers. The source code can be downloaded from the link at the end, and the explanation will concern:
- Create popup menu
- Design window settings
- Works with Nine-patch graphics
Preliminary preparation
1. Create an AdvancedHelloWorld application. For programs that do not use the specific functionality of a specific OS version, I prefer to install BuildTarget - Android 1.6, and Min SDK Version - 3. In this case, resource sharing by screen density (on hdpi, mdpi, ldpi) will work, but Android users 1.5 will not stay away.
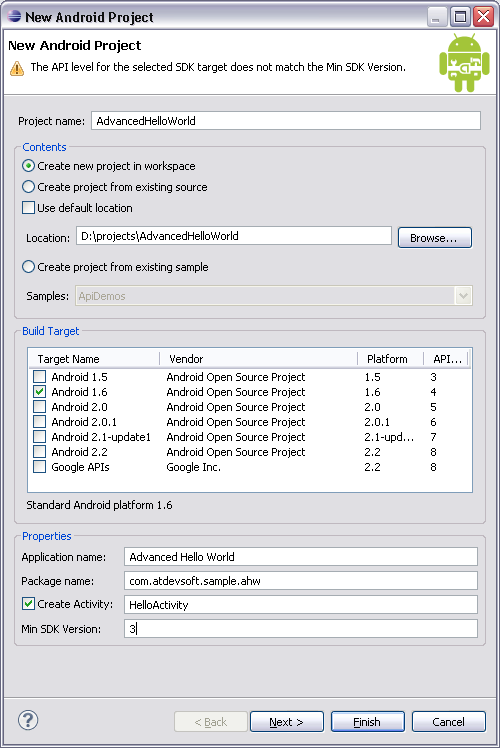
2. In res / layout / main.xml do one button "Say hello".
3. With the help of the wonderful project Android Asset Studio we make a nice icon.
4. As a click handler, we write a code that displays a Toast message with the phrase “Hello World”.
About Android Asset Studio
Open source online project whose value is difficult to overestimate. Allows you to quickly create application icons (launcher icons), menu icons, bookmarks and alerts. To work, he asks for Chrome 6, in other browsers, some elements do not work. Link at the end of the article.
Resource Remarks
In my opinion, the most rational choice is the use of a separate set of graphics for hdpi-devices and a separate one for all others, in resolution, adapted to mdpi. Yes, we do not use the possibility of adaptation under ldpi, but there are relatively few of these devices and you can leave a full adaptation for a “snack” when all the graphic design is already settled. In any case, since we declared support for Android 1.5, the drawable folder should contain all the graphic elements used. The rest is optional.
')
Pop-up menu
Menus are described, as it is not difficult to guess, in xml files. Let's make one such file for our main window: res \ menu \ main_menu.xml
<?xml version="1.0" encoding="utf-8"?>
<menu xmlns:android="http://schemas.android.com/apk/res/android">
<item android:id="@+id/menu_preferences"
android:icon="@drawable/ic_menu_preferences"
android:title="@string/menu_preferences" />
<item android:id="@+id/menu_theme"
android:icon="@drawable/ic_menu_theme"
android:title="@string/menu_theme" />
<item android:id="@+id/menu_close"
android:icon="@drawable/ic_menu_close_clear_cancel"
android:title="@string/menu_close" />
</menu>
Two points:
If the number of menu items is more than 6, the 6th and the following items are combined into an additional menu. An example can be viewed in a standard browser.
The menu can be nested, but not more than one level. To describe a submenu, a
menu tag is added as a child to the item that will “unfold”.
When creating a menu, the first thing to do is to look for icons in the standard set. All of them are in the installed SDK - android-sdk-windows \ platforms \ android-1.6 \ data \ res \ drawable \ ic_menu _ *. Png
The settings and exit icon is not difficult to pick up, but, for illustration, I added the theme menu item, which will switch the background from black to white and back. Even possessing quite basic computer graphics skills, it is easy to draw a template for this item as well. For example:
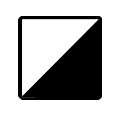
With the help of the same Android Asset Studio, you get quite a good icon:

The actual creation of menu items occurs in the onCreateOptionsMenu:
@Override
public boolean onCreateOptionsMenu(Menu menu) {
MenuInflater inflater = getMenuInflater();
inflater.inflate(R.menu.main_menu, menu);
return true;
}
Handle Processing - in onOptionsItemSelected:
@Override
public boolean onOptionsItemSelected(MenuItem item) {
switch (item.getItemId()) {
case R.id.menu_preferences:
...
default:
return super.onOptionsItemSelected(item);
}
}
Designing the settings window
And again, the main work in xml. In res \ xml, create settings.xml:
<?xml version="1.0" encoding="utf-8"?>
<PreferenceScreen
xmlns:android="http://schemas.android.com/apk/res/android">
<PreferenceCategory
android:title="@string/settings_general">
<CheckBoxPreference
android:key="center_message"
android:title="@string/center_message"
android:summary="@string/center_message_summary"
android:persistent="true"
android:defaultValue="true"/>
<EditTextPreference
android:key="text_message"
android:title="@string/text_message"
android:summary="@string/text_message_summary"
android:defaultValue="@string/hello_world"
android:persistent="true"/>
</PreferenceCategory>
</PreferenceScreen>
The set of supported settings is not very wide, but it will cover the basic needs. By setting the “persistent” flag we don’t even have to encode save / load. Just read the desired value from SharedPreferences:
SharedPreferences settings = PreferenceManager.getDefaultSharedPreferences(context);
boolean bCenter = settings.getBoolean("center_message", true);
As additional pleasant moments, you can note dependencies (android: dependency), which allow you to “turn on” certain items only in the case of a marked “master” element, as well as simple work with nested sets that look like separate windows in the application.
For the settings window, a descendant class PreferenceActivity is created, in the constructor of which our xml prototype is read:
addPreferencesFromResource(R.xml.settings);
PreferenceActivity is poorly styled. You can, of course, add android for the corresponding tag in AndroidManifest.xml: theme = "@ android: style / Theme.Light", but for the nested settings you will get porridge. Discussion of this problem on Stackoverflow boils down to the idea that, in general, it is better to leave everything as it is, against a black background.
Work with Nine-patch graphics
Nine-patch is a standard method for making graphics in an Android application independent of resolution and screen size. In fact, this is a regular image in png format with a single-click service frame. The SDK includes a special utility, draw9patch, to work correctly with this frame. A black line with a thickness of 1 pixel at the top and left marks the area that will be duplicated when the image is enlarged. The lines on the right and below define the “work area”. For a regular button, for example, this is the place for text.
In words, it’s quite difficult to explain the 9patch features, so it's best to experiment and watch, especially since when editing 9patch areas, you can immediately see the result in the preview window. Here is the background of the standard button in the editor:

For training, we will make a button in AdvancedHelloWorld non-standard, preparing 3 9patch images - for a normal button, a pressed state and a button in focus. Lesson is quite tedious, but it opens up great opportunities.
Important point: all 9patch images must have the extension .9.png, otherwise our application will not know that the image can be scaled according to the 9patch rules. Hence another limitation: in the resource folder there can not be two files with the same name and the extensions .png and .9.png (for example, button.png and button.9.png conflict, because they actually have the same resource name - button) .
useful links
Android Asset Studio on Googlecode:
http://android-ui-utils.googlecode.com/hg/asset-studio/dist/index.htmlOfficial documentation for creating submenus:
http://developer.android.com/guide/topics/ui/menus.html#submenuAn example with a complete list of types of settings:
http://www.kaloer.com/android-preferencesThe problem of styling settings:
http://stackoverflow.com/questions/2615528/preferenceactivity-and-theme-not-applyingSource codes