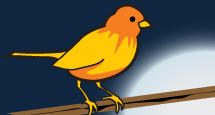
This article focuses mainly on those who have heard about this library, but does not mean where to start.
Intel Threading Building Blocks allows you to simplify the creation and deployment of multi-threaded applications, offering the developer a number of tools that allow you not to think about which architecture and which platform the program will be used on. It takes responsibility for working with threads.
Let's look at using Intel TBB using a simple example. Some time ago I gave an example of creating a multi-threaded application
using OpenMP , which brute forceed a password using its MD5 hash. Let's do the same thing, but using TBB.
I will make a reservation right away, this is not a guideline for writing a utility for selecting a password for its hash in MD5, in addition to brute force, for this kind of tasks, there are other, more elegant solutions.
')
First you need to
download the library . At the time of writing, the latest stable version of the library was version 3.0. Unpack the archive in any folder on the disk. In my case, it was the
D: \ Libs \ folder (the full path to the library, which turned out to be
D: \ Libs \ tbb30_20100406oss \ ).
This example was created in MS VS 2008, with a few exceptions, what was said in the article is true for other versions of MS VS.
Let's create a new console project. In the properties of the project, you must specify the path to the folder
Include , which contains the header files, and to the folder
Lib , containing the linker library.
Open the project properties, on the
C / C ++ -> General tab, in the
Additional Include Directories line, add the path to the
Include folder, in my case this is the path to
D: \ Libs \ tbb30_20100406oss \ include .

Next, go to the
Linker -> General section and add the path to the
Lib folder in the
Additional Library Directories field (
D: \ Libs \ tbb30_20100406oss \ lib \ ia32 \ vc9 ).
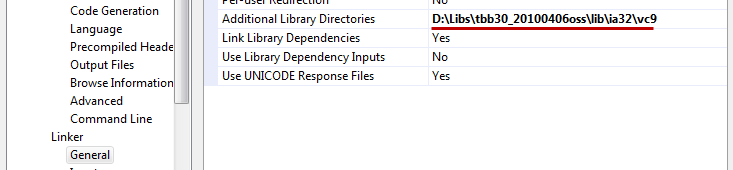
Please note that depending on the target platform and the MS VS version, the path to the lib folder may change, for example, for the 10th studio it will be
lib \ ia32 \ vc10 , for the 64-bit platform
lib \ intel64 \ vc9 .
Set up, you can start writing the program. Start by adding header files.
#include "tbb/task_scheduler_init.h"
#include "tbb/parallel_for.h"
#include "tbb/blocked_range.h"
using namespace tbb;
* This source code was highlighted with Source Code Highlighter .
In the
main function, before you start using, you must perform initialization by creating an instance of the class
task_scheduler_init .
int _tmain( int argc, _TCHAR* argv[])
{
task_scheduler_init init;
return 0;
}
* This source code was highlighted with Source Code Highlighter .
The concept of the library is such that the developer needs to create tasks that perform certain actions, for example, on data arrays that can be paralleled. In our case, the task will receive a certain range of numbers, within which it will calculate the MD5 hash for each number and compare it with the standard.
class MD5Calculate
{
public :
//////////////////////////////////////////////////////////////////////////
// , TBB
// ,
// ""
//////////////////////////////////////////////////////////////////////////
void operator () ( const blocked_range< int >& range) const
{
string md5;
stringstream stream;
for ( int i = range.begin(); i != range.end(); i++)
{
stream.clear();
stream << i;
GetMD5Hash(stream.str(), md5);
if (md5 == g_strCompareWith)
{
cout << "Password is: " << stream.str() << endl;
exit(0);
}
}
}
};
* This source code was highlighted with Source Code Highlighter .
This code will be called using the
parallel_for function
int _tmain( int argc, _TCHAR* argv[])
{
task_scheduler_init init;
//////////////////////////////////////////////////////////////////////////
// ,
// 8000000 8999999
//////////////////////////////////////////////////////////////////////////
parallel_for(blocked_range< int >(8000000, 8999999), MD5Calculate());
return 0;
}
* This source code was highlighted with Source Code Highlighter .
As you can see, everything is simple, and what is most remarkable, TBB is a cross-platform solution that will allow you not to think about how to work with streams under a particular platform.
Another advantage is that the library itself takes care of the optimal number of threads on the target platform. The number of threads will be equal to the number of processor cores.
The library is quite convenient and functional, in addition to the function for parallelizing the parallel_for loop, it also contains the functions
parallel_reduce ,
parallel_scan ,
parallel_do , containers, synchronization objects, and much more.
On the
official website of the library you can find library archives for various platforms including Windows, Linux, Mac OS X, Solaris, as well as documentation and examples of use.